Using Amazon Bedrock to generate images with Titan Image Generator models and AWS Lambda
The goal of this article is to document how one can test the Titan Image models in Amazon Bedrock using AWS Lambda. This article will be a quick start guide that can be adapted to suit your needs in the future. For developing the AWS Lambda, the AWS SAM framework will be used.
Published Jan 15, 2024
1. Create a skeleton SAM project
Using the sam init command, create a new skeleton project. This sample will use a Python lambda that is exposed via an API Gateway. The Hello World Example sam template has been followed to create the skeleton project.
Once the sam init command is finished running, tweak the generated code to suit theuse case you are working on.
Below is the template.yaml that has been generated for this article.
2. Deploy initial sam stack
Deploy the initial sam stack using the sam deploy command.
In the output section of the sam deploy command will be an AmazonBedrockApi endpoint as per the image below.
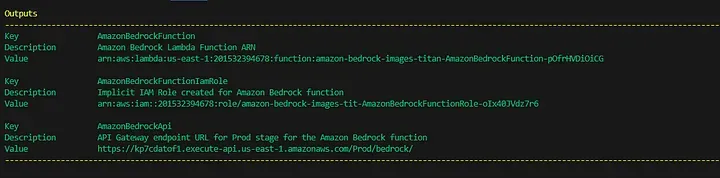
Click onto the AmazonBedrockApi link to validate it is running as expected.
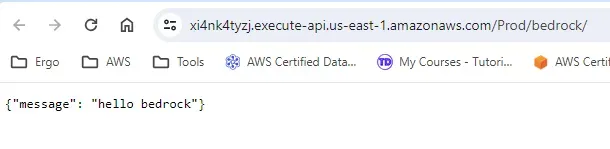
3. Enable the Titan Image models.
At the time of writing (14th December 2023), the Titan Image models are only available in certain regions. For this demo, we will configure resources in the us-east-1 (North Virginia) region as the required models are available in that region.
Navigate to the Amazon Bedrock service in the North Virginia region and select model access on the left hand menu.
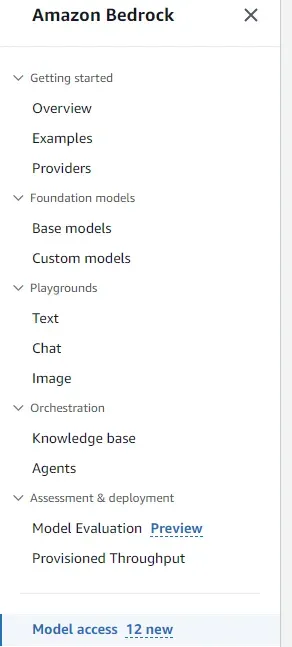
Select the Titan Image Generator models and click Request model access

4. Update the lambda code to call Amazon Bedrock
In this section, the lambda code will be updated to call the Titan Image Generator model in Amazon Bedrock.
Note: For python boto3 examples — see the following AWS Link
The boto3 library currently configured for python on AWS Lambda does not support the bedrock-runtime client. If you are to load bedrock-runtime from boto3 — you get the following error
To get around this, we can update the boto3 dependency in requirements.txt to specify which version of boto3 should be used
Or we can use a lambda layer — which is what we are going to do in this example.
Note: All code will be shared at the end of this article which also shows
The updated template.yml looks like the following
The final lambda code to call a Titan Image Generator AI model in Amazon Bedrock is as follows.
6. Build code and deploy
Then we can perform a build using the following command to download the required dependencies and finally deploy the code using the following commands
7. Test the code
By calling the API Gateway endpoint with a query string parameter of prompt — we can now test the solution.
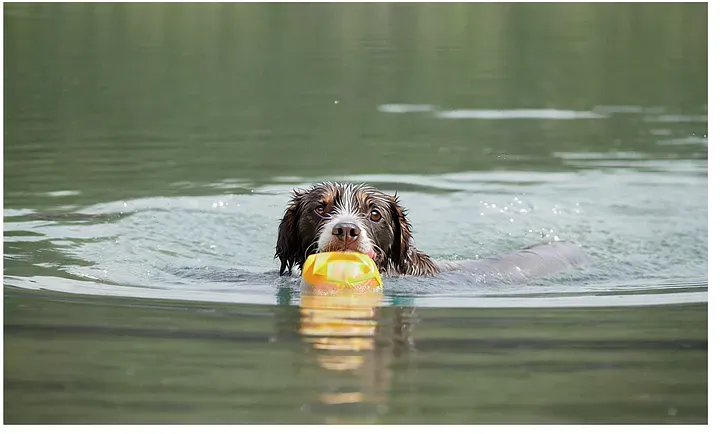
The above sample could be improved by using a HTTP Post request instead of a GET request but the purpose of this article was to get a working solution as quickly as possible.
8. Code
If you wish to view the code for this sample project — it can be viewed on GitHub.