
How to host a Plotly Dash app on AWS ECS
Creating the infrastructure using AWS Cloud Development Kit (CDK) in Python
Published Jan 17, 2024
This article presents the steps to host a Plotly Dash app on AWS Elastic Container Service with AWS Fargate, the serverless container solution from AWS. Part of the steps is how to create the Docker image of the application, as required by AWS ECS to host an application on it.
The required infrastructure to host the Plotly Dash application will be built using the AWS Cloud Development Kit (CDK) tool, in Python.
The Plotly Dash app presented here is a very simple dashboard, based on this tutorial. The most important point here is how to create the required infrastructure to host this application on AWS.
In this way, this article can be a good start to create a data analytics application on AWS.
The idea is to use the AWS ECS service with the AWS Fargate to host the Plotly Dash app with at least two instances. Also, the data source of the dashboard will be stored in a S3 bucket as a CSV file.
To this case, the AWS Application Load Balancer is good choice, but it's necessary to configure it properly to let the dashboard be presented to the user in the Web interface.
The following picture is a simplified diagram of the proposed solution:
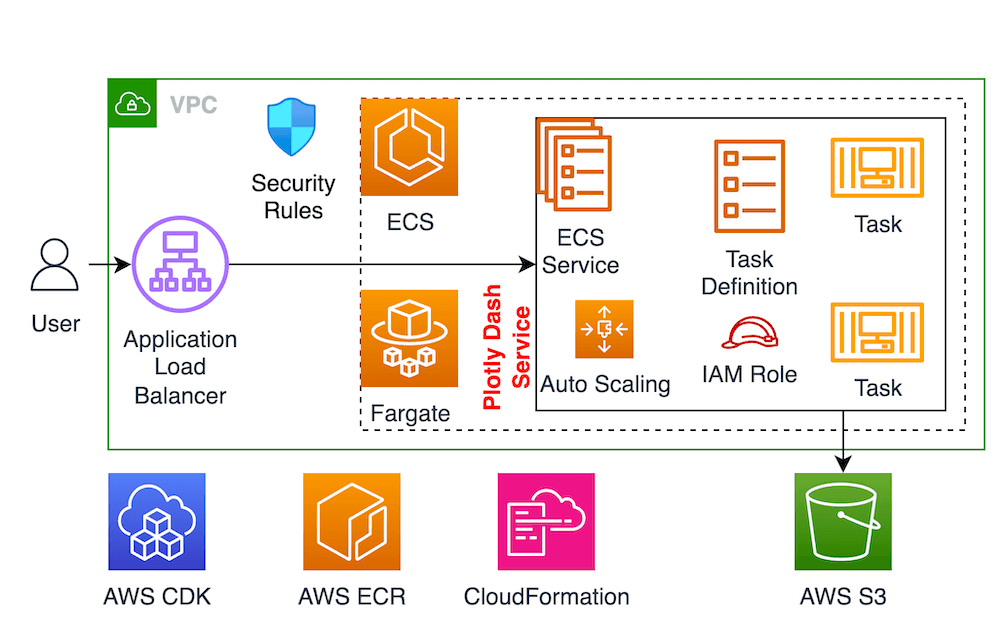
The Docker image of the Plotly Dash application will be hosted by a private repository, created on the AWS ECR service.
To create the proposed infrastructure, the AWS Cloud Development kit will be used.
To start, just create a new CDK project with the following command:
cdk init app --language python
This command will create the project using the Python language.
The next sections describe the required parts to be created in the CDK project, as stacks.
The ECR repository needs to be created to host the Plotly Dash Docker image that will be generated later in this article.
The following code creates a CloudFormation stack with an ECR repository. This repository will be used by the ECS task definition, to define the location of the Plotly Dash Docker image.
As explained in the project architecture, the Plotly Dash application will be hosted in an AWS ECS service, that's why an AWS VPC is required to build this infrastructure.
The following code creates a new VPC, with the default configuration, which includes two availability zones with a NAT Gateway.
As this project uses the AWS Fargate, the AWS ECS cluster creation is very simple, as described in the following code:
One of the key AWS resources to host this application is the Application Load Balancer, but for now, just the main part of this resource needs to be created.
The AWS ECS service section of this article will create the remaining pieces of the Application Load Balancer and their configurations.
The AWS S3 bucket is a good option to store the dashboard data source of this project, as a CSV file.
The following code creates a new CloudFormation stack with a new S3 bucket.
Now it's time to create the AWS ECS service to effectively host the Plotly Dash application. This is the most complex stack in this project.
The following code has the whole implementation, but next there are explanations about each part.
First, the AWS ECS task definition is created, defining the amount of CPU and memory to be used by the application. This task definition has a task role, which needs to have the permission to read the created S3 bucket, where the CSV to be used the Plotly application is.
Then, the application container is added to this task definition. Note that the created ECR repository is the source of the Docker image. The port mappings attribute has the network configuration required by the Plotly Dash application. The environment attribute carries the bucket name, which will be used by the AWS SDK inside the Plotly Dash application.
Next, the Fargate service is created using the created task definition. Note that this service will create two instances of the application, without public IP address.
The TCP port used by the application is added to the security group configuration, to let the traffic reach the application.
Now, a listener is added to the Application Load Balancer. This will expose the application through this service.
Finally, a target group is added to the Application Load Balancer. This will let the ALB forward the incoming traffic to the application port.
Besides the network configuration to use the same TCP port, there is the stickness attribute with the value true. This configuration let the ALB keeps the connection established from a client, which is required by a Plotly Dash application. This is very important, because there are two instances of the application and after a connection is established with one of the instances, it needs to maintain on the same instance.
Another important configuration here is the health check. This mechanism allows the target group of the ALB keep checking the healthy of each instance. This will guarantee the availability of the application.
The HTTP endpoint configured in the health mechanism needs to be created in the Plotly Dash app, as will be explained later.
These stacks need to be organized in the main file of the CDK project. So, just create an instance of each one, as describe in the following code.
Note that there some dependencies between some stacks, which is something normal to happen.
The Plotly Dash app presented here is a very simple dashboard, based on this tutorial.
The next sections will explain some important details, especially to host it on AWS.
These are the dependencies to be added to the project. The boto3 contains the AWS SDK library, to access the AWS S3 bucket.
This is a very simple Dockerfile to create the Docker image of this application.
This is the application code to expose a very simple dashboard using the Plotly express library.
The HealthCheck class creates an endpoint to be used by the health check mechanism, as explained in the AWS ECS Service section of this article.
The Boto3 S3 client is initialized to fetch the CSV file, that will be used as the dashboard data source.
This CSV file is read using the Pandas library to create a data frame.
Finally, this data frame will compose the dashboard of this application.
To deploy this application, first the ECR repository must be deployed using the following command:
cdk deploy Ecr --require-approval never
Then, the Docker image of the application must be generated and pushed to the created ECR repository.
Next, deploy the S3 bucket, with the following command:
cdk deploy Bucket --require-approval never
Now, upload this CSV file to the bucket, using the AWS S3 console.
Finally, the whole infrastructure can be created with the following command:
cdk deploy --all --require-approval never
After the deployment process, the ALB endpoint will be outputted by the CDK process. Use this endpoint with the 8050 port to access the application hosted on AWS.
This article explained a few steps to host a very basic dashboard application built with Plotly Dash.
The AWS CDK helps a lot when building the required infrastructure, which is a little bit complex.