
Deploy Your Web Application to staging and production with Elastic Beanstalk, AWS CDK, CloudFront, and Circleci pipelines
In this guide, I cover the following key aspects Elastic Beanstalk- Load balancers- EC2- Relational Database Service (RDS)- Route 53- CloudFront- Virtual Private Cloud (VPC)- Security Groups- SSL/TLS Certificate Manager - Secrets Manager- S3 Buckets- Namecheap Integration- NextJS/ReactJS CloudFront deployment- Papertrail monitoring & logging- CircleCI pipelines- Staging and Production Environments
Published Jan 22, 2024
Last Modified Feb 2, 2024
A demonstration of how to use AWS CDK Pipelines and Elastic Beanstalk to deploy a web application and speed up the development & deployment process.
In the second part of the guide, I will show you how to deploy the React/Next.Js app as well to s3 and Cloudfront.
In the end, we will build Circleci ci/cd pipelines to deploy our application to staging or production automatically & will set up Papertrail monitory logs for our elastic Beanstalk application.
AWS Elastic Beanstalk provides a solution for this. It’s a user-friendly service designed for deploying and scaling web applications and services. Supporting various programming languages and server configurations (Java, .NET, PHP, Node.js, Python, Ruby, Go, and Docker with servers like Apache, Nginx, Passenger, and IIS), Elastic Beanstalk simplifies deployment by accepting a single ZIP or WAR file. It takes care of tasks like capacity provisioning, load balancing, auto-scaling, and application health monitoring, while still granting us control over the underlying AWS resources.
In this guide, we will learn how to
- Deploy your web application by creating an Elastic Beanstalk environment and configuring its settings.
- Set up an RDS instance to host your application’s relational database with the desired engine and security settings.
- Manage your domain’s DNS records and hosted zones on Route 53 for efficient domain resolution.
- Accelerate content delivery and reduce latency by creating a CloudFront distribution, configuring origins, and associating it with your Elastic Beanstalk environment.
- Isolate and control your network environment by setting up a Virtual Private Cloud with proper subnets, route tables, and internet gateways.
- Define security groups for services like Elastic Beanstalk and RDS to control inbound and outbound traffic securely.
- Secure your domain with SSL/TLS certificates from AWS Certificate Manager, associating them with your CloudFront distribution and Elastic Beanstalk environment.
- Safely store and manage sensitive information, such as database credentials, using AWS Secrets Manager and integrate it with your Elastic Beanstalk environment.
- Create and configure S3 buckets for storing static assets, backups, and other necessary files with proper access controls.
- Automate your build and deployment workflows by configuring Circle CI with a .circleci/config.yml file and integrating it with your version control system.
- Point your domain to AWS by updating DNS records on Namecheap and associating the domain with your AWS resources.
A Platform-as-a-Service (PaaS) offered by AWS that simplifies the deployment and management of applications. It automatically handles infrastructure provisioning, capacity scaling, load balancing, and application health monitoring.
You can manually create Elastic beanstalk application by following the aws guide
https://docs.aws.amazon.com/elasticbeanstalk/latest/dg/GettingStarted.CreateApp.html
https://docs.aws.amazon.com/elasticbeanstalk/latest/dg/GettingStarted.CreateApp.html
A managed database service that simplifies the setup, operation, and scaling of relational databases like MySQL, PostgreSQL, and others.
You can manually create RDS by following the AWS guide
https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_CreateDBInstance.html
https://docs.aws.amazon.com/AmazonRDS/latest/UserGuide/USER_CreateDBInstance.html
Let’s focus on creating the Elastic Beanstalk app, RDS, and VPC via aws CDK 🚀
Copy the account ID, navigate to security credentials, and create access keys.
Copy the account ID, navigate to security credentials, and create access keys.
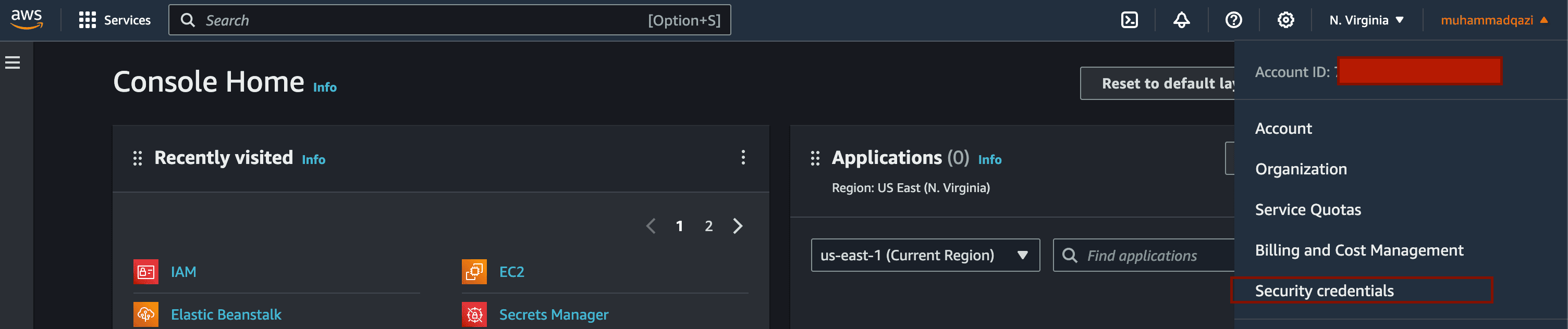
~/.aws/config
filePlease install the specific version of the CDK to match the dependencies that are installed later on.
Example:
Initialize the CDK application that we will use to create the infrastructure.
We are going to delete the default file created by CDK and define our code for all the ElasticBeanstalk resources stack, RDS, and VPC stacks.
Add the following code in /lib as elbtest-stack.ts, rds-infrastructure.ts, and vpc-stack.ts respectively.
Below AWS CDK script defines an AWS CloudFormation stack for an Elastic Beanstalk environment. It includes the creation of an S3 asset from a specified directory containing application code, an Elastic Beanstalk application, an application version tied to the S3 asset, and the necessary IAM roles and instance profiles. It also configures options such as autoscaling group settings and environment properties.
Make sure to replace the application source code directory with the correct one. you can also find this code in my GitHub repository
The below script creates an Amazon RDS (Relational Database Service) instance within an AWS CDK stack. It takes in a Virtual Private Cloud (VPC) and a security group as input parameters, generates a secret for database credentials using AWS Secrets Manager, and creates an RDS instance configured for MySQL. The script also creates an AWS Systems Manager (SSM) parameter containing the ARN of the generated database credentials secret.
Make sure to replace the name according to your application above.
Below AWS CDK script defines a VPC stack with multiple subnets for a fictional application named “YOUR_APP_NAME” It creates a VPC with public, private, and isolated subnets, configures a gateway endpoint for Amazon S3 in the private subnet, and sets up security groups for a bastion host, an Elastic Load Balancer (ELB), an Auto Scaling Group (ASG), an RDS instance, and an ElastiCache instance. Ingress and egress rules between these security groups are defined to control traffic flow within the VPC.
Make sure to replace the name according to your application above.
Finally, add the following code to /bin as aws-deploy.ts
The above script serves as the entry point for deploying AWS CloudFormation stacks using the AWS Cloud Development Kit (CDK). It begins by configuring the environment with necessary modules, loading environment variables, and initializing the CDK application. It defines three stacks representing VPC, RDS database, and Elastic Beanstalk, interconnecting them and establishing dependencies to ensure proper deployment order. The script concludes by synthesizing the CloudFormation templates for deployment. Overall, it orchestrates the deployment of a comprehensive AWS infrastructure, including networking, a relational database, and a scalable application environment.
You will need to install some additional dependencies, add those with the following command
You will need to also bootstrap the cloud formation if this is your first time.
Then to build and create the CloudFormation template:
The above profile is the name you add in
~/.aws/config
file.Finally, let's deploy the stack,
This might take a while, be patient.
You will need to make some changes in security groups to access the RDS database from your local machine via Beekeeper or SQL workbench.
Go to RDS, and select the database instance you want to access.
You will need to add your machine IP address to security groups, and sometimes add IP address to routing tables as well. Make sure the database is publicly accessible.

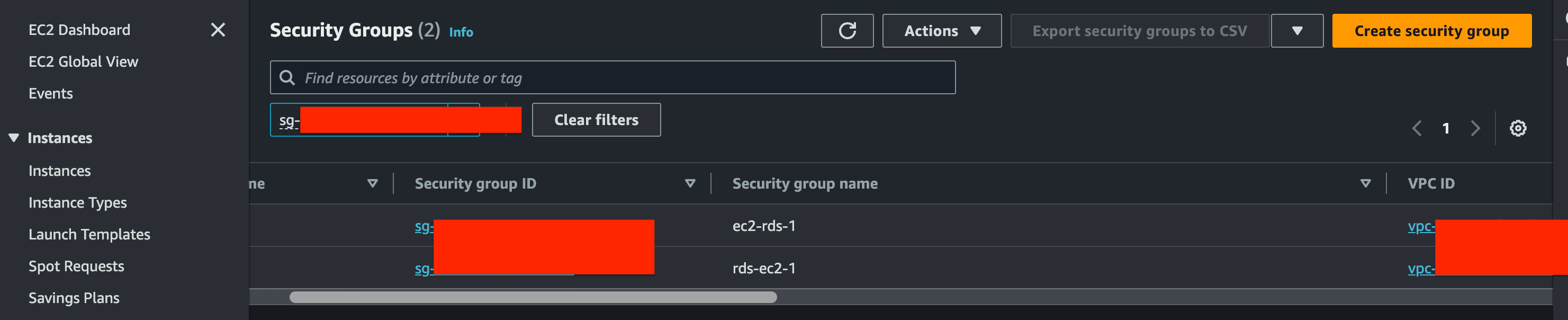
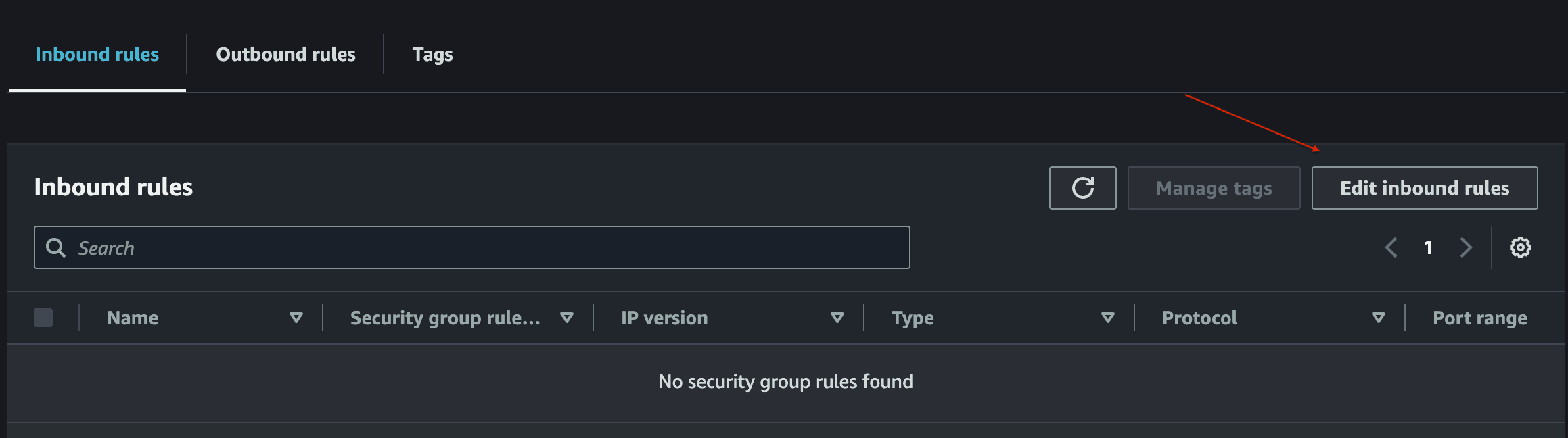

Click on the subnet shown in the above figure, locate the routing table press Edit routes, and add your IP address, you can find your IP by the link

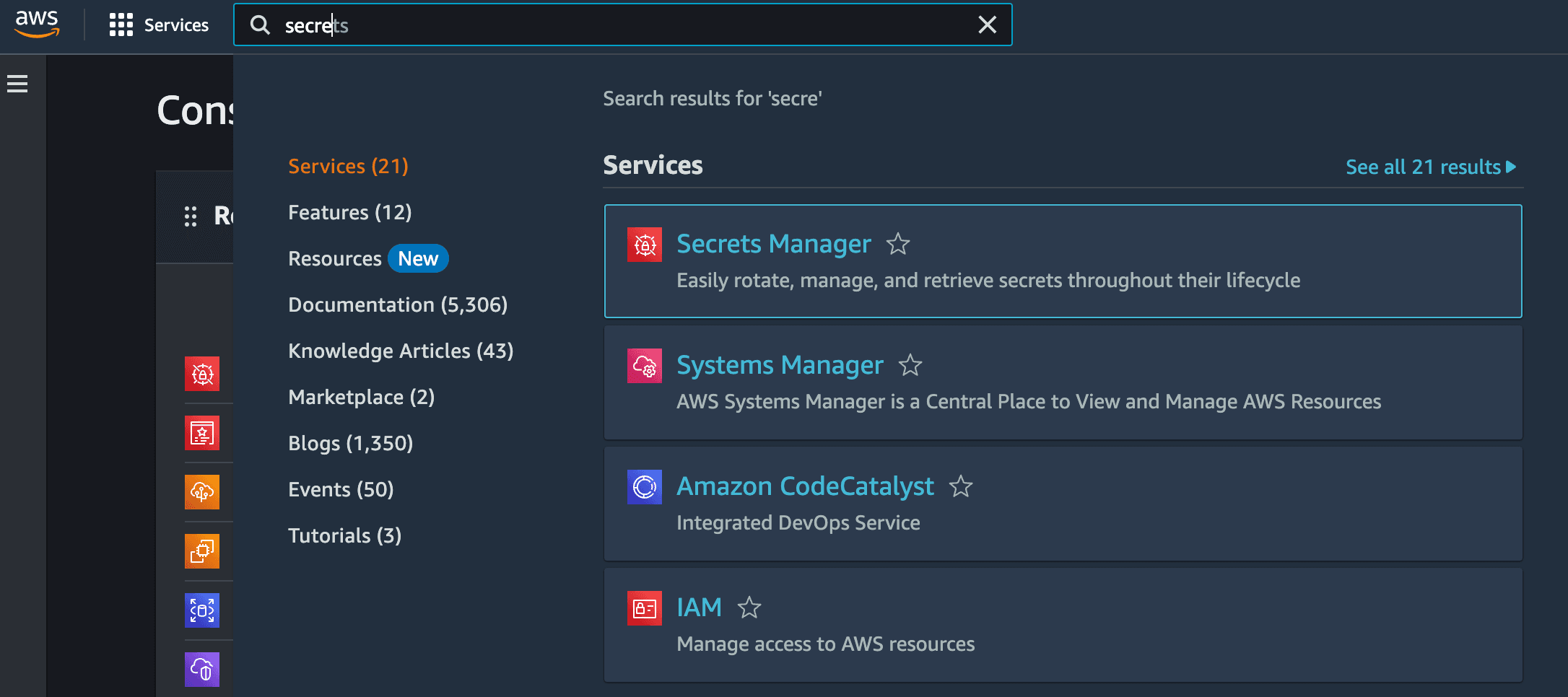
For this step, you will need to generate an SSL/TLS certificate for your domain, using AWS certificate manager, and add Route 53 DNS in your domain provider panel. I am using namecheap for my domain management and will show you how to do that.
If you want to enable HTTPS for your elastic Beanstalk auto-generated link, you can follow some additional steps below.
Search Certificate Manager in the AWS console, and press the request button, you will be asked to fill in your domain and add some certificate settings.
You will see a certificate generated but is pending.
Make sure to remove the (.) dot from the end with your domain name example:
_somevalue12312.domain.com.
just remove .domain.com.

Now, Let’s enable HTTPS for the elastic beanstalk auto-generated link, then use a custom domain for elastic beanstalk.
Open your elastic beanstalk environment, go to Configure instance traffic and scaling, in the configuration menu, and add the HTTPS listeners there.
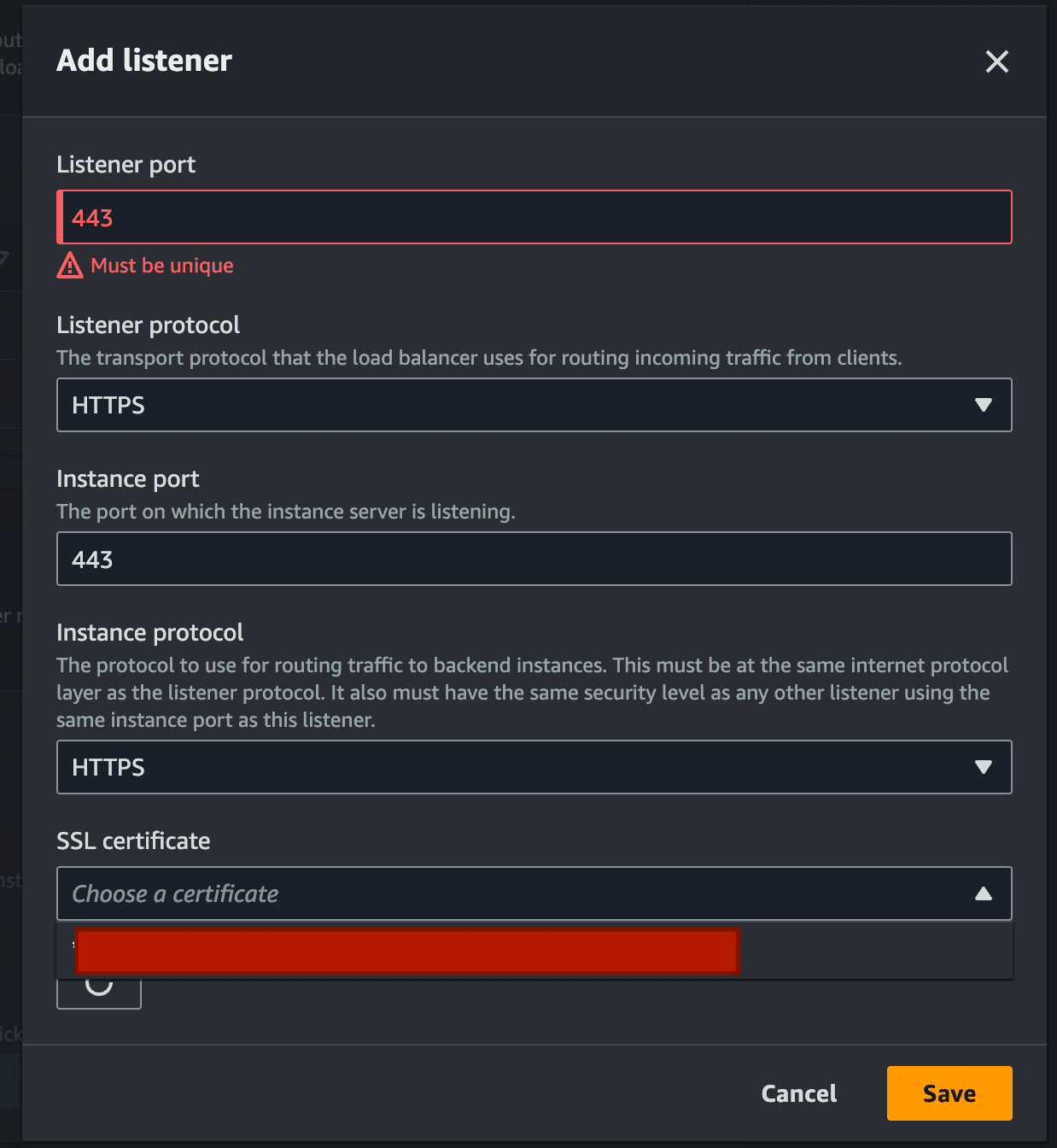
Fill out the above form and choose the certificate which you just generated.
To update the security policy using the console
- Open the Amazon EC2 console at https://console.aws.amazon.com/ec2/.
- On the navigation pane, choose Load Balancers.
- Select the load balancer, scroll down click Manage Listeners, and add the following setting
Rule for the Security Group of the Load Balancer
You should be good to go with the HTTPS protocol now.
Search for Route 53 in the AWS console, navigate to hosted zones from the menu, and press Create a hosted zone.




To host Next.js/React.js applications using S3 and CloudFront, first, deploy the Next.js application using the
next build
command to generate the production-ready build.Next, create an Amazon S3 bucket and upload the contents of the build output to the bucket. Make sure the bucket is publicly accessible, after you upload the contents of the application in the S3 bucket navigate to the properties tab and scroll all the way down you will see the option to make this app static, select that option and add index.js as root origin. After finishing this step a link will be generated to access your application.
Now, we need to add bucket policies, you should be able to see that in the permissions tab, click Edit and add the following policies
Now, here you need Cloud Front identity, go to the AWS console and search for Cloud Front.
Navigate to the security tab, then origin access, there you will be able to see your CloudFront identities, copy the ID, and add it to the above policy.
After finishing this step, create Cloudfront's new distribution.
Here in the origin domain, you need to add that static website link without http:// or https:// , which was generated in the above step, and choose HTTP only.

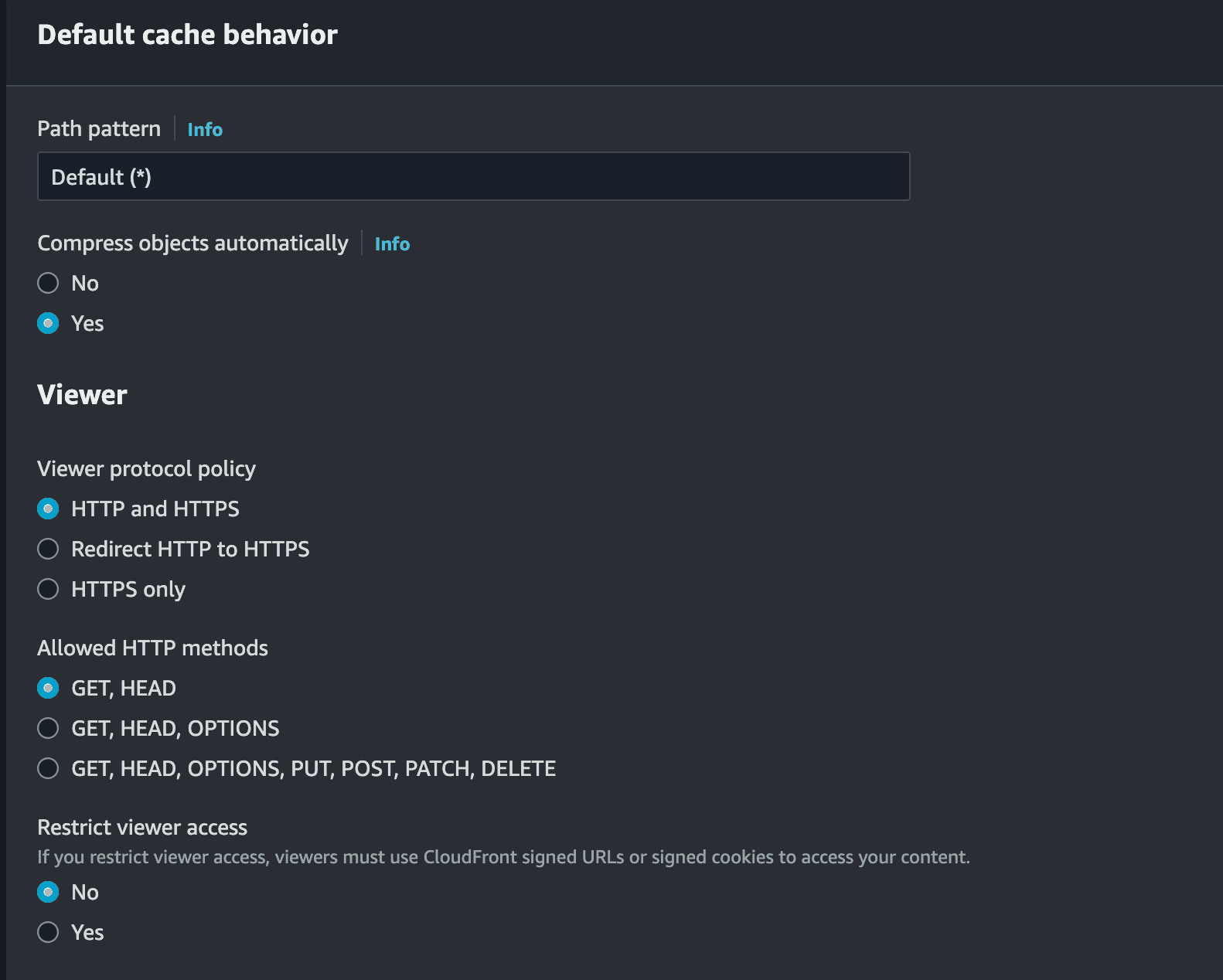
By using git now we will deploy our application to staging and production using CircleCi.
Firstly, add the following environment variables to your circle ci application.
Create .circleci/config.yml in your project root and add the following code this code will push the elastic Beanstalk application to stage or production depending on the GitHub branch you are pushing code to.
Now, create .elasticbeanstalk/config.yml in your project root directory and add the following code, make sure to change the application name, region, and platform depending on what you are deploying
After your first deployment with CDK, this pipeline will be triggered by GitHub which will automatically build and deploy applications to elastic Beanstalk.
Add the file to your project directory in .circleci/config.yml, make sure to replace the YOUR_DISTRIBUTION_ID with yours.
After your first deployment, this pipeline will be triggered by GitHub which will automatically build and deploy your application to S3 and invalidate the cloudfront.
Papertrail is a cloud-based log management and log aggregation service that allows users to collect, monitor, and analyze log data from various sources. It simplifies the process of centralizing log information, making it easier for developers, system administrators, and IT teams to troubleshoot issues, monitor system performance, and gain insights into the behavior of their applications and infrastructure.
To add a papertrail to your elastic beanstalk app, we need to first generate key pairs for EC2 your elastic beanstalk app is pointing to.
Search EC2 in the AWS console, and navigate to key pairs in the network and security menu, press create, give it a name and this will generate a .pem file for your EC2 instance.
Navigate to elastic beanstalk environment configurations, edit service access, and select the key pair file that we generated above.
connect to ssh, and run the papertrail install script.
You can check the GitHub repo for CDK code, and all the code used here.
Happy deploying 🚀