Generate and store images in S3 using Titan Image Generator G1
A quickstart guide on deploying a SAM app that stores Bedrock images in S3 and creates logs in CloudWatch.
Albert
Amazon Employee
Published Feb 8, 2024
Last Modified Feb 9, 2024
"Dogfooding" is core to how we ship at AWS.
Our teams worked hard to get Titan Image Generator G1 out the door for re:Invent last year, feeding large datasets into our model and test-running countless prompts to produce realistic images. Our internal teams are already finding use cases for G1, and we're just getting started.
To see for myself how easy it was to get started, I made a Python SAM app that invokes a Lambda to generate an image in Bedrock, stores the image in an S3 bucket, and finally logs the invocation in CloudWatch.
I logged into my AWS account and requested access in the Bedrock console for Titan Image Generator G1. Access was given almost instantaneously.
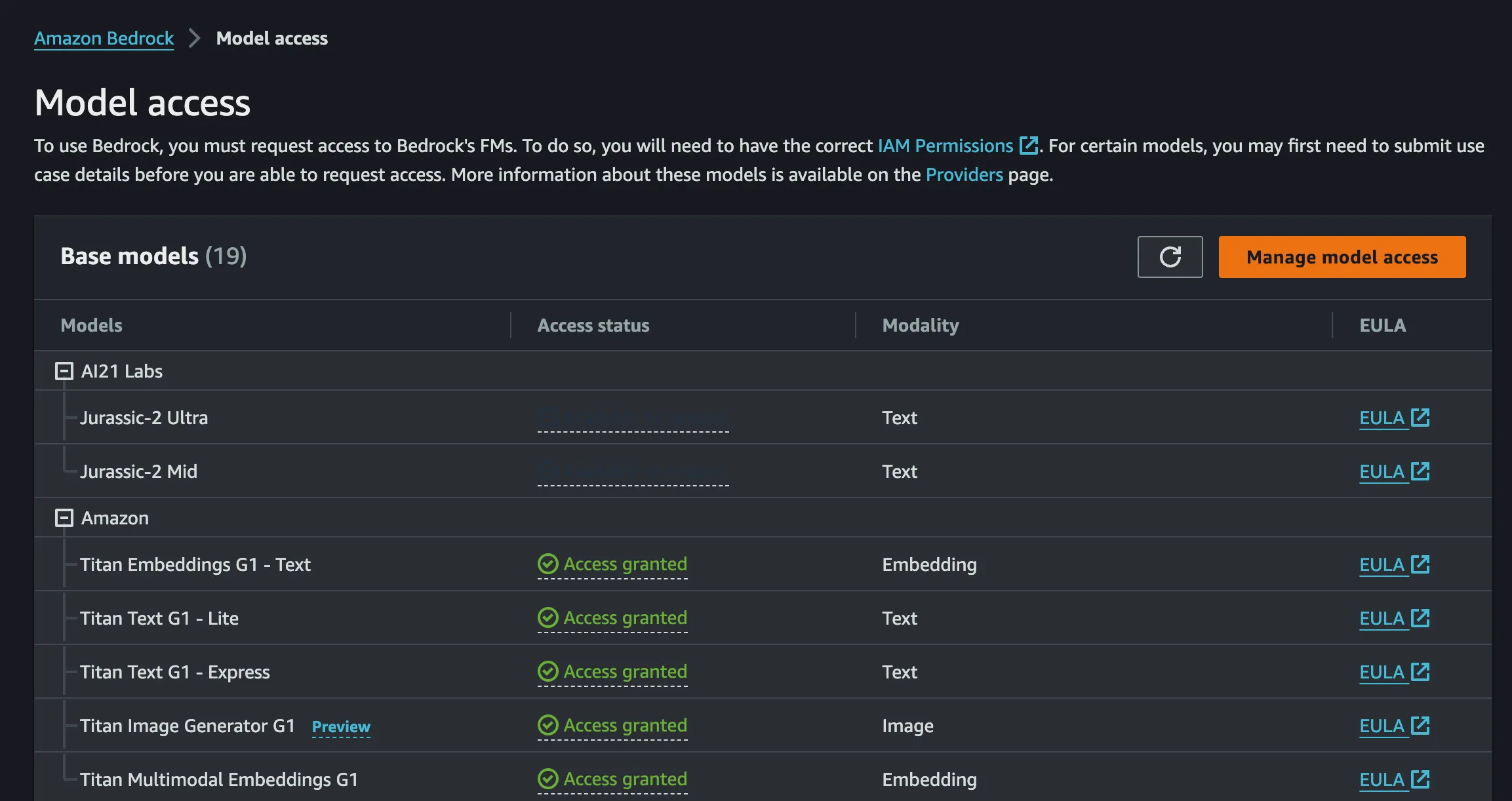
Time to generate my Python SAM app and get started:
The
Hello World Example
in SAM makes it easy to spin up a template.yaml
for me to use as a basis to connect all the AWS services. I also enabled structured logging in JSON for my Lambda functions for CloudWatch.I create a directory called
s3imagegenerator
in my SAM app for the new Lambda, then added my app.py
file. My Lambda needs to generate an image based on given text and seed parameters, and then uploads the image into S3.
When triggered, Lambda should first extract the
'text'
and 'seed'
values from the event object. Afterwards, it then calls a function that constructs a request to Bedrock with these parameters. I'll get a base64-encoded image in the response, but then I need to decode it into binary, so it'll upload into S3 under a dynamically generated object key. CloudWatch will log each step I just outlined.Time to set up my
template.yaml
and start putting together the AWS services I'll be using (Lambda, S3, CloudWatch, Bedrock).I run
sam build
and sam deploy
. Everything is looking good, so let's use the AWS CLI and invoke the Lambda locally with a prompt:
I get an error. I check the generated
response.json
, but it's unclear. Finally, I open CloudWatch and see this: "log_level": "ERROR", "errorMessage": "Unknown service: 'bedrock-runtime'
Ah, looks like the default boto3 SDK in Lambda is not up to date for Bedrock. I add a
requirements.txt
file in my s3imagegenerator
directory with the latest version: boto3==1.34.27
I run the Lambda again and boom: my S3 bucket now stores an image of a dog with a cowboy hat, which you can see in this post's header.
And so everyone is happy, here's a cat that G1 made:
Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.