Deploy a S3 Bucket and CloudFront using AWS CDK
Deploy a S3 bucket along with a CloudFront Distribution using AWS CDK with TypeScript. This method is used to access the S3 bucket assets publicly.
Published Feb 6, 2024
In this article, I'm going to explain about how to create a S3 bucket and expose it publicly with CloudFront using AWS Cloud Development Kit(CDK). CDK allows developers to define infrastructure as code, making it easier to manage and deploy AWS resources. Those stacks can be written in different languages but in this article I'm using TypeScript which is the most commonly used language.
CDK should be bootstrapped, you can follow Getting Started with the AWS CDK document. Once CDK and the AWS account is properly configured, required Node and npm modules should be installed for TypeScript and this Working with the AWS CDK in TypeScript document explain it clearly.
Once all the prerequisites are done. Add the below code blocks inside the CDK stack in the lib folder.
Above code is to create the S3 bucket and required CORS rules. If you want to grant read/write access to a user or a role in AWS it is possible to create the required code blocks in this CDK stack.
In the same file add above code blocks to create the CloudFront distribution and required OAI configuration. I have not configured HTTPS since I do not have a domain and a certificate. If you own a domain and SSL certificate there are extra configuration blocks to add in this same function.
After creating the proper code blocks and stack, it is possible to check what will be created and configured using below command,
cdk diff
To deploy the components use the below command with the correct stack name,
cdk deploy S3CdkStack
After all the components are created, it is possible to access S3 bucket using cloudfront distribution domain. I have added a temporary index.html file into S3 bucket and it is possible to access that page now,
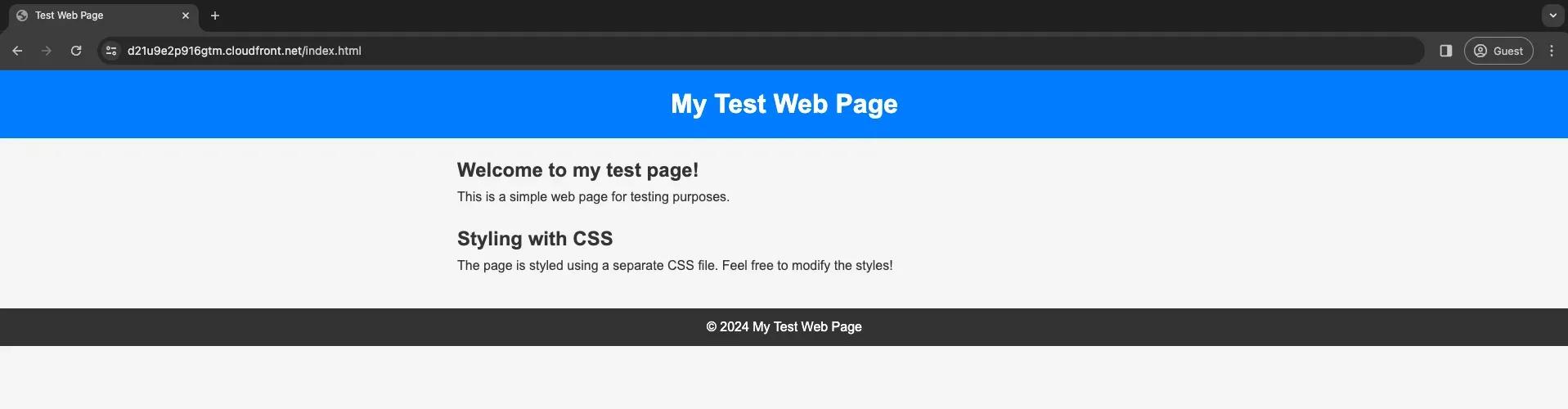
To destroy the stack simply execute below command,
cdk destroy S3CdkStack
Well done! You’ve created an S3 bucket and configured CloudFront using CDK and TypeScript. This infrastructure as code solution provides a clear and controllable way to manage AWS resources, making it easier to grow and maintain your applications. Explore further CDK features and AWS services to improve your cloud infrastructure.