Cricket Match Winner Prediction with Amazon Bedrock's Anthropic Claude 3 Sonnet
Utilizing Amazon Bedrock's new Claude 3.0 Sonnet Model, predict cricket match winners by extracting data from match statistics images for predictive insights.
1
2
! python --version
Python 3.11.5
1
! pip install --upgrade pip
1
2
3
4
! pip install --no-build-isolation --force-reinstall \
"boto3>=1.33.6" \
"awscli>=1.31.6" \
"botocore>=1.33.6"
1
2
3
4
5
6
7
import json
import os
import sys
import boto3
import botocore
bedrock = boto3.client(service_name="bedrock")
bedrock_runtime = boto3.client(service_name="bedrock-runtime")
1
2
# Define the prompt text to ask the model about the content of the uploaded image
input_text = "Your task is to create a comprehensive Cricket Match Stats Analysis & Outcome Prediction, Extract stats from uploaded images, including player performances and match conditions. Use predictive modeling to forecast match outcomes based on provide data"
1
2
model_id = 'anthropic.claude-3-sonnet-20240229-v1:0'
max_tokens = 256
1
2
3
4
5
6
7
8
9
10
11
from PIL import Image
from IPython.display import display
# Open the image
img = Image.open('stats_images/Star-batting-performance.png')
# Convert the image to RGB mode
img = img.convert('RGB')
# Display the image
display(img)
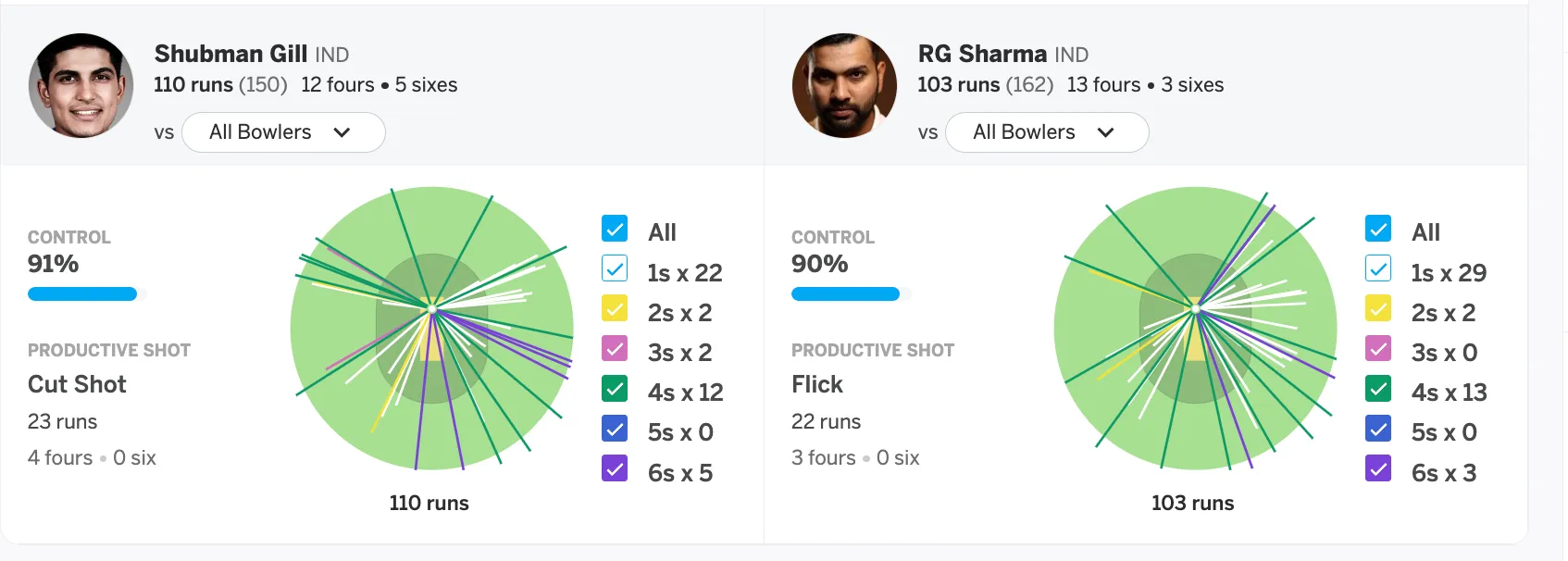
1
2
3
4
import base64
input_image = "stats_images/Star-batting-performance.png"
with open(input_image, "rb") as image_file:
content_image = base64.b64encode(image_file.read()).decode('utf8')
1
2
3
4
5
6
7
8
9
# Invoke the Model: Construct the API request body including the image data and prompt text, then send a POST request to the model API
message = {"role": "user",
"content": [
{"type": "image", "source": {"type": "base64",
"media_type": "image/png", "data": content_image}},
{"type": "text", "text": input_text}
]}
messages = [message]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
def run_multi_modal_prompt(bedrock_runtime, model_id, messages, max_tokens):
"""
Invokes a model with a multimodal prompt.
Args:
bedrock_runtime: The Amazon Bedrock boto3 client.
model_id (str): The model ID to use.
messages (JSON) : The messages to send to the model.
max_tokens (int) : The maximum number of tokens to generate.
Returns:
None.
"""
body = json.dumps(
{
"anthropic_version": "bedrock-2023-05-31",
"max_tokens": max_tokens,
"messages": messages
}
)
response = bedrock_runtime.invoke_model(
body=body, modelId=model_id)
response_body = json.loads(response.get('body').read())
results = response_body.get("content")[0].get("text")
return results
1
2
response_claude_sonnet = run_multi_modal_prompt(bedrock_runtime, model_id, messages, max_tokens)
print(json.dumps(response_claude_sonnet, indent=4))
The image shows detailed batting stats for two cricket players - Shubman Gill and RG Sharma - in what appears to be an innings from a cricket match. The stats are presented in circular charts that break down the runs scored through various shot types and scoring regions.\n\nFor Shubman Gill, the key stats shown are:\n- Total runs scored: 110 (off 150 balls)\n- Fours hit: 12\n- Sixes hit: 5\n- His most productive shot was the cut shot, scoring 23 runs including 4 fours
1
2
3
4
5
6
7
8
9
10
11
from PIL import Image
from IPython.display import display
# Open the image
img = Image.open('stats_images/Star-Batting-Bowling-Performance.png')
# Convert the image to RGB mode
img = img.convert('RGB')
# Display the image
display(img)
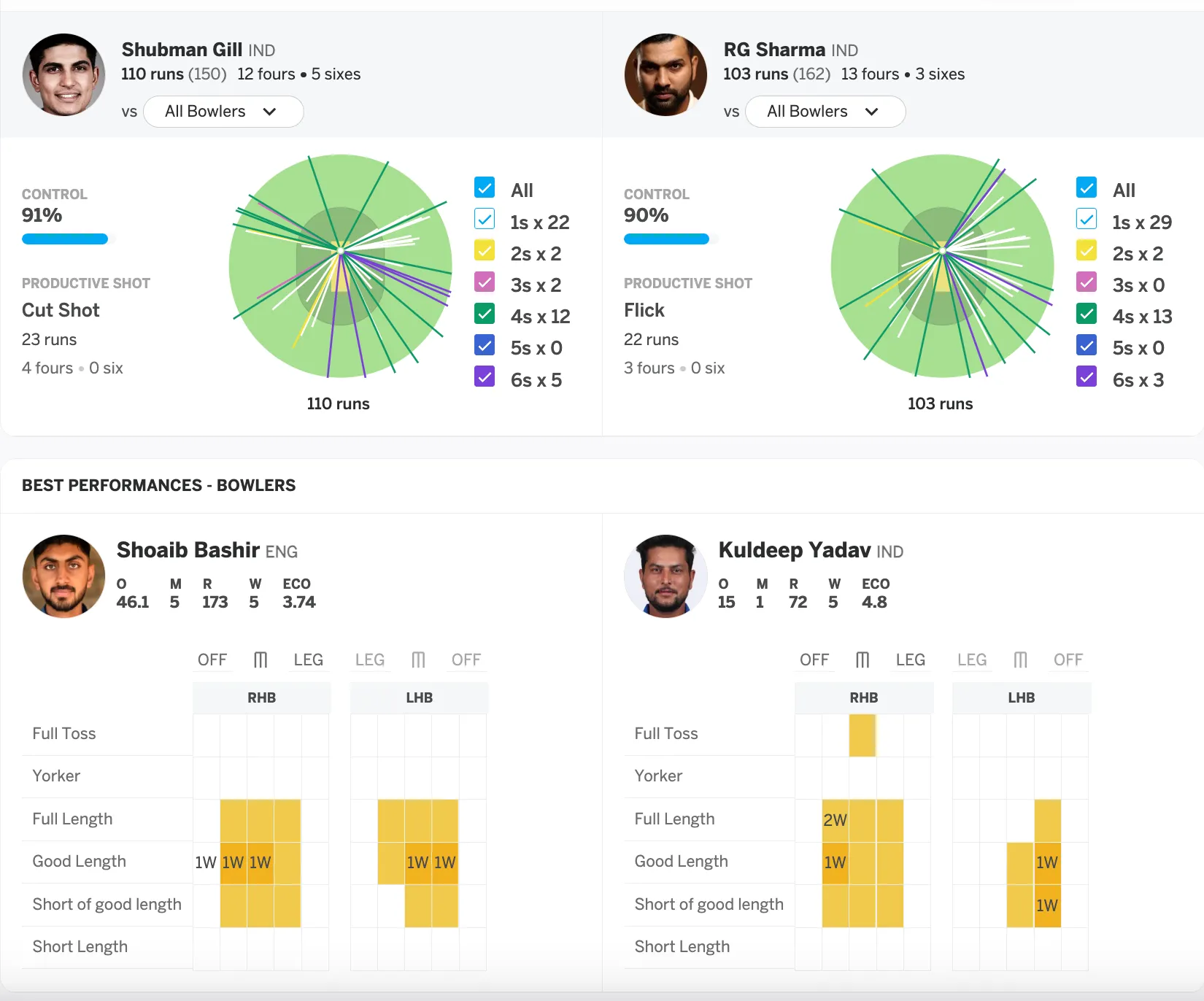
1
2
3
4
import base64
input_image2 = "stats_images/Star-Batting-Bowling-Performance.png"
with open(input_image2, "rb") as image_file:
content_image = base64.b64encode(image_file.read()).decode('utf8')
1
2
3
4
5
6
7
8
9
# Invoke the Model: Construct the API request body including the image data and prompt text, then send a POST request to the model API
message = {"role": "user",
"content": [
{"type": "image", "source": {"type": "base64",
"media_type": "image/png", "data": content_image}},
{"type": "text", "text": input_text}
]}
messages = [message]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
def run_multi_modal_prompt(bedrock_runtime, model_id, messages, max_tokens):
"""
Invokes a model with a multimodal prompt.
Args:
bedrock_runtime: The Amazon Bedrock boto3 client.
model_id (str): The model ID to use.
messages (JSON) : The messages to send to the model.
max_tokens (int) : The maximum number of tokens to generate.
Returns:
None.
"""
body = json.dumps(
{
"anthropic_version": "bedrock-2023-05-31",
"max_tokens": 256,
"messages": messages
}
)
response = bedrock_runtime.invoke_model(
body=body, modelId=model_id)
response_body = json.loads(response.get('body').read())
results = response_body.get("content")[0].get("text")
return results
1
2
response_claude_sonnet_2 = run_multi_modal_prompt(bedrock_runtime, model_id, messages, max_tokens)
print(json.dumps(response_claude_sonnet_2, indent=4))
Based on the image, cricket match statistics can be analyzed to provide insights as follows:
- Shubman Gill (IND) scored 110 runs off 150 deliveries, including 12 fours and 5 sixes. His most productive shot was the cut shot (23 runs, 4 fours).
- RG Sharma (IND) scored 103 runs off 162 deliveries, with 13 fours and 3 sixes. His most productive shot was the flick shot (22 runs, 3 fours).
- Shoaib Bashir (ENG) bowled 5 overs, conceding 46 runs while taking 5 wickets at an economy rate of 3.74. His lengths were mostly good length and full length deliveries.
- Kuldeep Yadav (IND) bowled 1 over, conceding 15 runs while taking 1 wicket at an economy rate of 4.8. His lengths were mostly short of good length and short length deliveries.
1
match_result_analysis = "Your task is to create a comprehensive Cricket Match Stats Analysis and Outcome Prediction based on the image provided, The match is between India vs England 5 Match Test series. Provide player name, country with statistics. Use predictive modeling to forecast match outcomes based on date from only uploaded image. Need the prediction of Match with team or country name on who will win the match based on the data. Give me the output in bullet points. I am giving some stats for this series"
1
2
model_id = 'anthropic.claude-3-sonnet-20240229-v1:0'
max_tokens = 512
1
2
3
4
5
6
7
8
9
10
11
from PIL import Image
from IPython.display import display
# Open the image
img = Image.open('stats_images/PerformanceSeries.png')
# Convert the image to RGB mode
img = img.convert('RGB')
# Display the image
display(img)
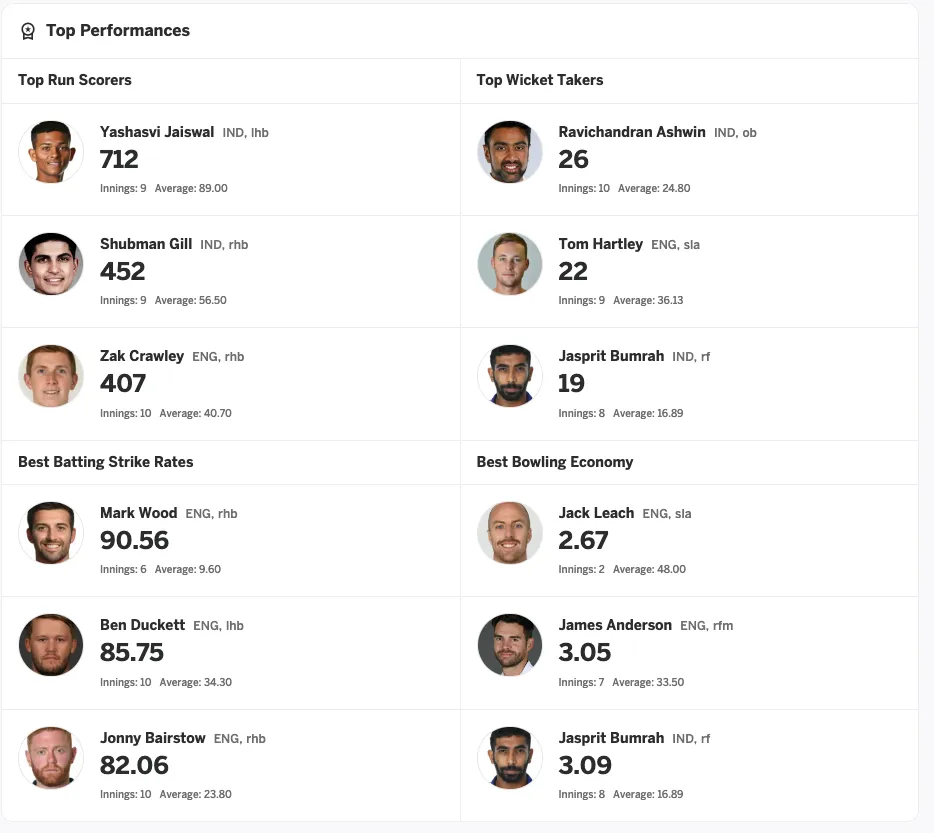
1
2
3
4
import base64
input_image3 = "stats_images/PerformanceSeries.png"
with open(input_image3, "rb") as image_file:
content_image = base64.b64encode(image_file.read()).decode('utf8')
1
2
3
4
5
6
7
8
message = {"role": "user",
"content": [
{"type": "image", "source": {"type": "base64",
"media_type": "image/png", "data": content_image}},
{"type": "text", "text": match_result_analysis}
]}
messages = [message]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
def run_multi_modal_prompt_analysis(bedrock_runtime, model_id, messages, max_tokens):
"""
Invokes a model with a multimodal prompt.
Args:
bedrock_runtime: The Amazon Bedrock boto3 client.
model_id (str): The model ID to use.
messages (JSON) : The messages to send to the model.
max_tokens (int) : The maximum number of tokens to generate.
Returns:
None.
"""
body = json.dumps(
{
"anthropic_version": "bedrock-2023-05-31",
"max_tokens": max_tokens,
"messages": messages
}
)
response = bedrock_runtime.invoke_model(
body=body, modelId=model_id)
response_body = json.loads(response.get('body').read())
results = response_body.get("content")[0].get("text")
return results
1
2
response_claude_sonnet = run_multi_modal_prompt_analysis(bedrock_runtime, model_id, messages, max_tokens)
print(json.dumps(response_claude_sonnet, indent=4))
Based on the provided stats for the India vs England 5-match Test series, here is a comprehensive analysis and match outcome prediction:
- Yashasvi Jaiswal (IND) - 712 runs at 89.00 average
- Shubman Gill (IND) - 452 runs at 56.50 average
- Zak Crawley (ENG) - 407 runs at 40.70 average
- Ravichandran Ashwin (IND) - 26 wickets at 24.80 average
- Tom Hartley (ENG) - 22 wickets at 36.13 average
- Jasprit Bumrah (IND) - 19 wickets at 16.89 average
- Mark Wood (ENG) - 90.56
- Ben Duckett (ENG) - 85.75
- Jonny Bairstow (ENG) - 82.06
- Jack Leach (ENG) - 2.67
- James Anderson (ENG) - 3.05
- Jasprit Bumrah (IND) - 3.09
• England has a solid batting lineup with good strike rates
• Both teams have quality bowlers, but India's attack looks slightly better