The Legend of AWS Warrior: A Free Opensource 3D RPG Adventure Game with Generative AI for learning AWS
"The Legend of AWS Warrior" is an innovative approach to learning AWS through 3D RPG gaming at Hong Kong Institute of Information Technology (HKIIT). By integrating various AWS services such as Amazon Bedrock, AWS Lambda, Amazon S3, and Amazon DynamoDB into an AWS SAM serverless application, it offers a practical, hands-on experience with AWS Academy.
Published May 3, 2024
Last Modified Oct 14, 2024
"The Legend of AWS Warrior" is an innovative approach to learning AWS through gaming at Hong Kong Institute of Information Technology (HKIIT) @ IVE(Lee Wai Lee). By integrating various AWS services such as AWS Lambda, Amazon S3, and Amazon DynamoDB into a AWS SAM serverless application, it offers a practical, hands-on experience. It's designed to help students using the AWS Academy Learner Lab and AWS Educate Lab to engage with cloud computing concepts in a fun and interactive way through Amazon Bedrock. The game allows for customization, enabling educators and learners to add new unit tests and expand the learning framework. This project not only makes learning about AWS more accessible but also encourages the development of practical skills in a simulated environment.
Student interacts with a virtual NPC (non-player character) girl to receive instructions generated by Amazon Bedrock. Their mission: to defeat a digital monster, an action that cleverly triggers a unit test in AWS Lambda. This gamified approach makes learning about cloud infrastructure engaging and fun.
First and foremost, we extend our gratitude to SimonDev. The game is a modification of his repository, Quick_3D_RPG.
AWS Academy Learner Lab is a long-running hands-on lab environment where educators can bring their own assignments and invite their students to get experience using select AWS Services. Due to limitations imposed by AWS, every student will be provided with an AWS account with US$50. However, this account has certain restrictions, including the disabling of certain AWS services such as Auroa serverless. Additionally, students will not have access to modify IAM permissions. As a result, assignments should not rely on SQL databases as a component.
This AWS account provides students with the ability to generate 4-hour IAM session tokens for the AWS SDK or AWS CLI. These tokens are used by our backend system to assess and validate the AWS resources and configurations of the students.
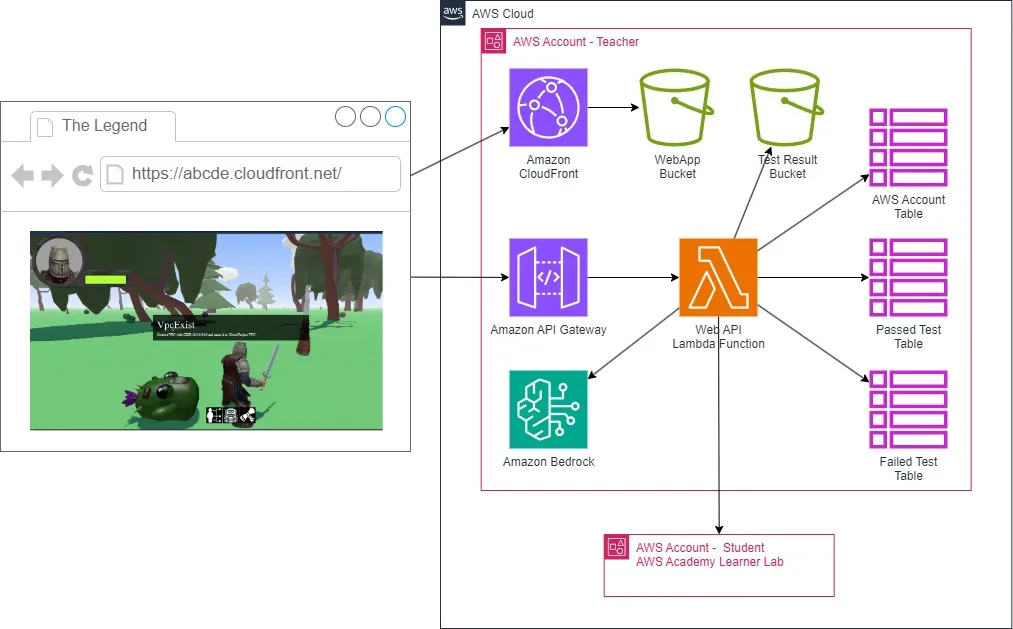
1. ReactJS Site (index.html): The static website is built using ReactJS. It includes a landing page for students to submit their IAM session key and check their marks. The hash key is from the teacher by email, and it is a unique key.
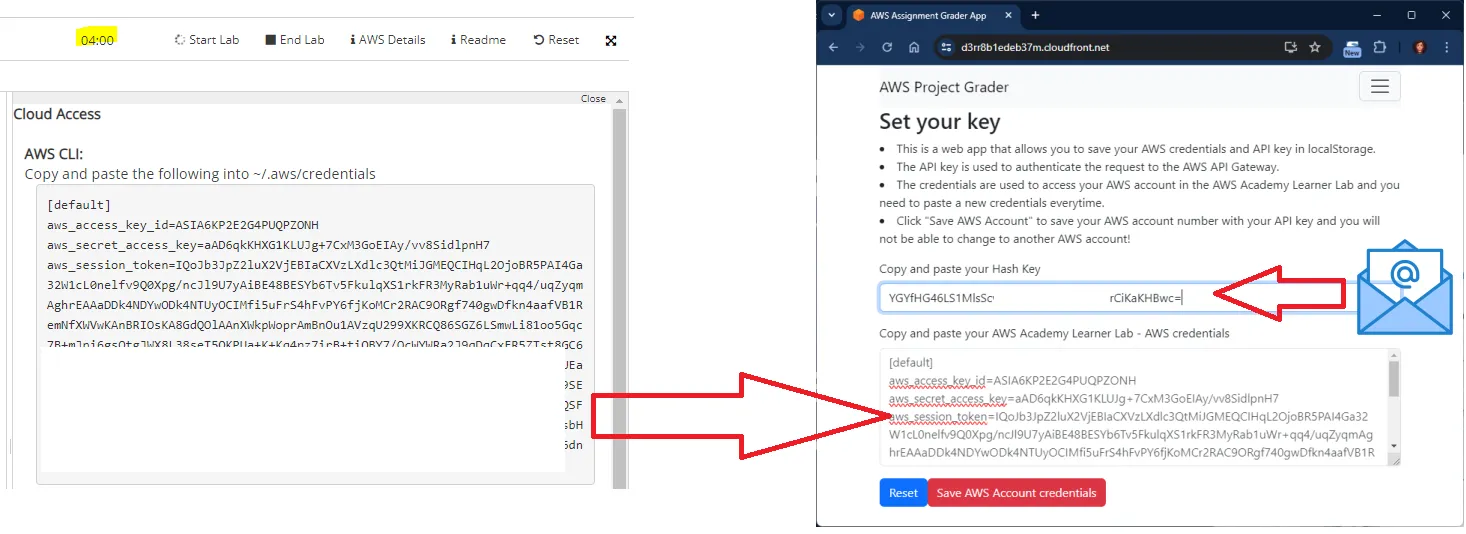
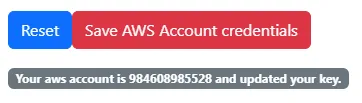
After 4 hours, students need to resubmit the key again.
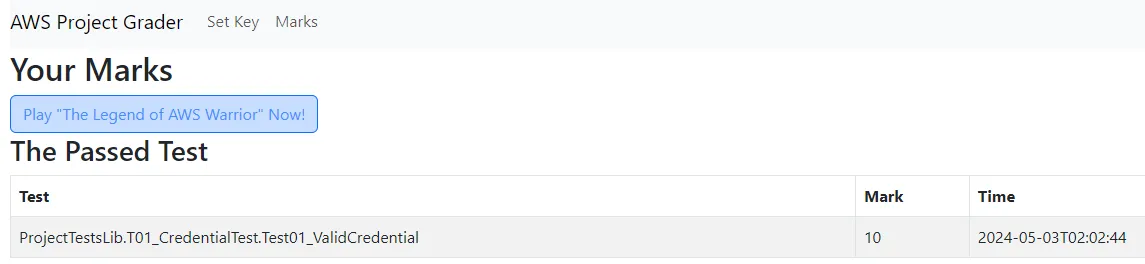
Students find an NPC and click on it. The NPC will give them an AWS task or an encouraging message with Amazon Bedrock.
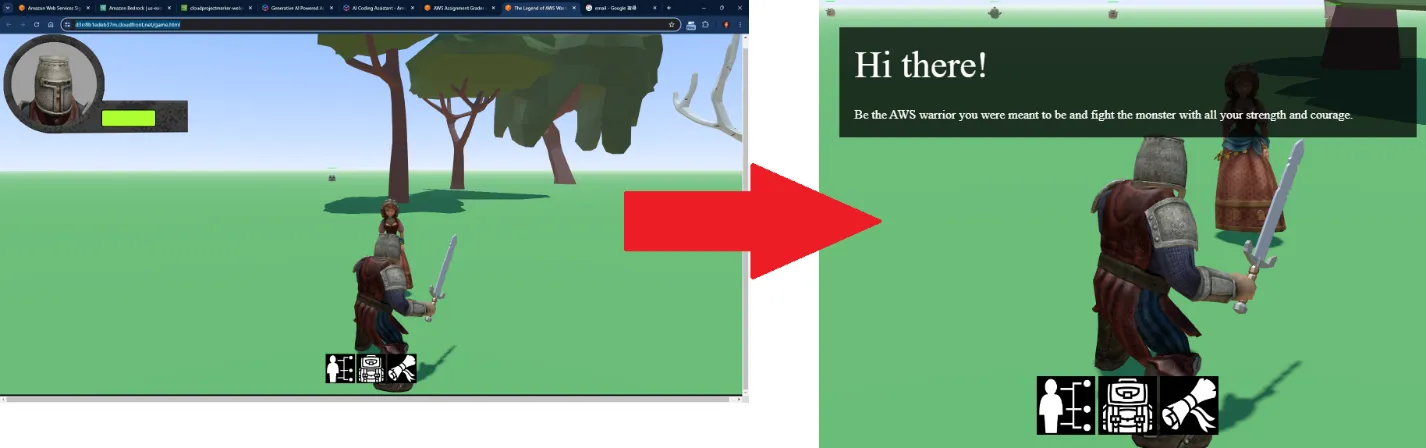
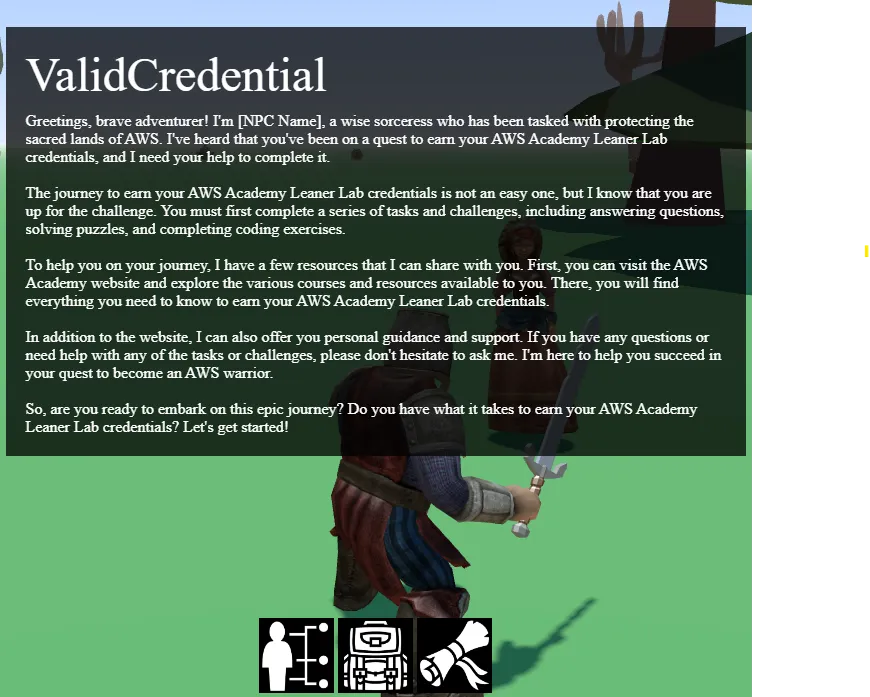
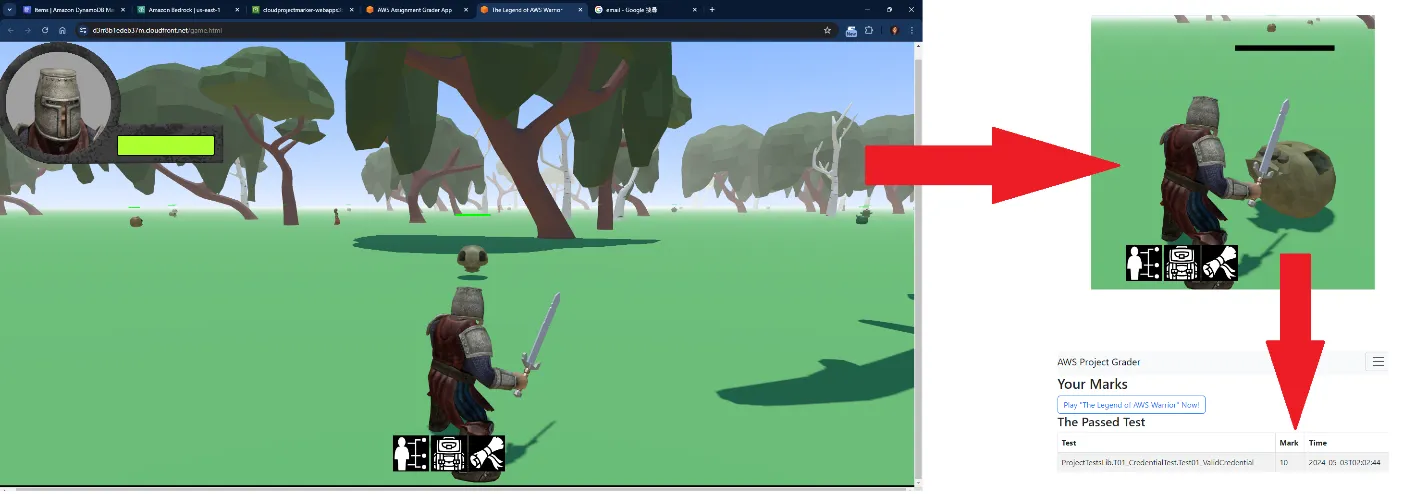
This is a C# .NET 8 WebAPI App Lambda with Amazon API Gateway REST integration, created from the default template app using the “sam init” command.

This library contains NUnit tests that utilize the AWS .NET SDK to query students’ AWS account resources and settings. The tests then assert the expected values.
- The GameClass attribute defines the order and timeout for game-related tasks.
- The GameTask attribute provides the raw instructions, time limit, and reward for specific tasks. Although the time limit is not currently in use, in the next version, the system will calculate the time between students receiving the task and completing it. This adjustment will allow us to fine-tune rewards based on completion time.
This is a Microsoft Asp Net Core Web Application and it uses the API key of usage plan for authentication.
Add CORS settings to global for the need of preflight "OPTIONS" support.
Then, add headers to all response headers
It manages AWS IAM session tokens. Behind the scenes, it checks and saves the email, AWS account number, and AWS IAM session token in an AwsAccountTable (Table will be refer to Amazon DynamoDB table).


This function is triggered when students click on an NPC. It has two possible behaviors:
- Random Message Generation: Sometimes, it returns a randomly generated message using the Amazon Bedrock model with the ID amazon.titan-text-express-v1.
- Task Retrieval: If not generating a random message, it checks the PassedTestTable in Amazon DynamoDB. If there’s an incomplete task, it returns the details of the next task to the student. To extract task details, the function uses reflection on the GameClassAttribute and GameTaskAttribute.
Additionally, before returning the instruction, there’s a 70% chance that it will be rephrased by Amazon Bedrock.
When a student defeats a monster, this function is activated. It executes the corresponding NUnit tests in the ProjectTestsLib for a specific task. The function then stores the raw test result XML, auto-converted JSON, and test output log in the Test Result S3 bucket. A task may consist of multiple tests. Passed tests are saved to the Passed Test Table, while failed tests are recorded in the Failed Test Table. This design allows for tracking student progress and trial behavior. Additionally, students can retrieve the most recent failed test output log from the mark’s web page by S3 presigned URL.
It provides simple URL to generate email hash for each student, and we can use Google Spreadsheet to create key easily.

Then, we use mail merge to send the hash key to students.
During practical test, we need to grade all students in parallel every 5 minutes instead of killing a monster. We use Event Bridge and AWS Step Function to solve the problem.

Not all unit tests have been included, as my students are still working on this assignment project.
1. Fork the repo.
2. Create a new Codesplaces.
3. Set configure AWS CLI.
4. Run “./deploy.sh b14ca5898a4e4133bbce2e123456123456” and remember to change the hash.
Students can design their own characters and monsters using the Amazon Titan Image Generator. Furthermore, it is recommended to include NUnit tests in all free AWS Educate lab exercises.
"The Legend of AWS Warrior" is an innovative approach to learning AWS through gaming at HKIIT. By integrating various AWS services such as AWS Lambda, Amazon S3, and Amazon DynamoDB into an AWS SAM serverless application, it offers a practical, hands-on experience. It's designed to help students using the AWS Academy Learner Lab and AWS Educate Lab to engage with cloud computing concepts in a fun and interactive way through Amazon Bedrock. The game allows for customization, enabling educators and learners to add new unit tests and expand the learning framework. This project not only makes learning about AWS more accessible but also encourages the development of practical skills in a simulated environment.
Cyrus Wong is the senior lecturer of [Hong Kong Institute of Information Technology (HKIIT) @ IVE(Lee Wai Lee).](http://lwit.vtc.edu.hk/aboutmit_message.html) and he focuses on teaching public Cloud technologies. He is a passionate advocate for the adoption of cloud technology across various media and events. With his extensive knowledge and expertise, he has earned prestigious recognitions such as AWS Machine Learning Hero, Microsoft Azure MVP, and Google Developer Expert for Google Cloud Platform & Ai/ML(GenAI).
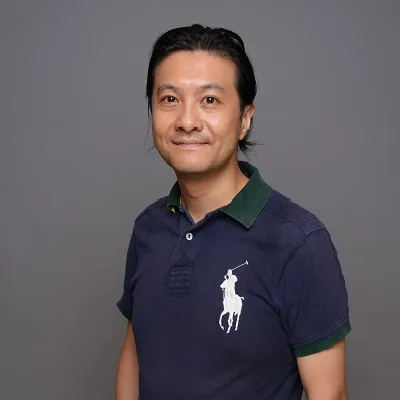