Secure your Burnbook: Learn how to add encryption in transit to your apps using Pangea Vault and AWS Amplify
Learn how to secure sensitive data in your application with encryption in transit. Just like the "Burn Book" from Mean Girls, your user data demands protection. Dive into data encryption in this blog post and implement a simple encryption-in-transit middleware for robust security.
Published May 7, 2024
With a new data breach every week securing sensitive data in the application layer is not just a good practice, it's a necessity. Like the infamous "Burn Book" from the movie Mean Girls, which taught us the repercussions of mishandling sensitive information, our application’s user data today also demands rigorous protection to prevent leaks and unauthorized access. In this blog post, we will dive into data encryption, why it’s not as tedious as it sounds, and how to implement a simple encryption-in-transit middleware to do all the heavy lifting for us (lazy devs 😉).
Implementing data encryption from scratch sounds like a tedious task that would increase latency in our application. I’m here to tell you that encryption can be implemented more efficiently.
When we refer to data encryption implementation, there are two common strategies: encryption at rest and encryption in transit. Encryption at rest is when you encrypt a whole database with an encryption key stored in a secure vault or KMS. The more convenient method of encryption in transit works in a way to only encrypt the fields you want to encrypt thus allowing you to retain search and regular CRUD operations on fields that aren’t as sensitive.
TL;DR: Here’s a visual explanation of how encryption in transit works
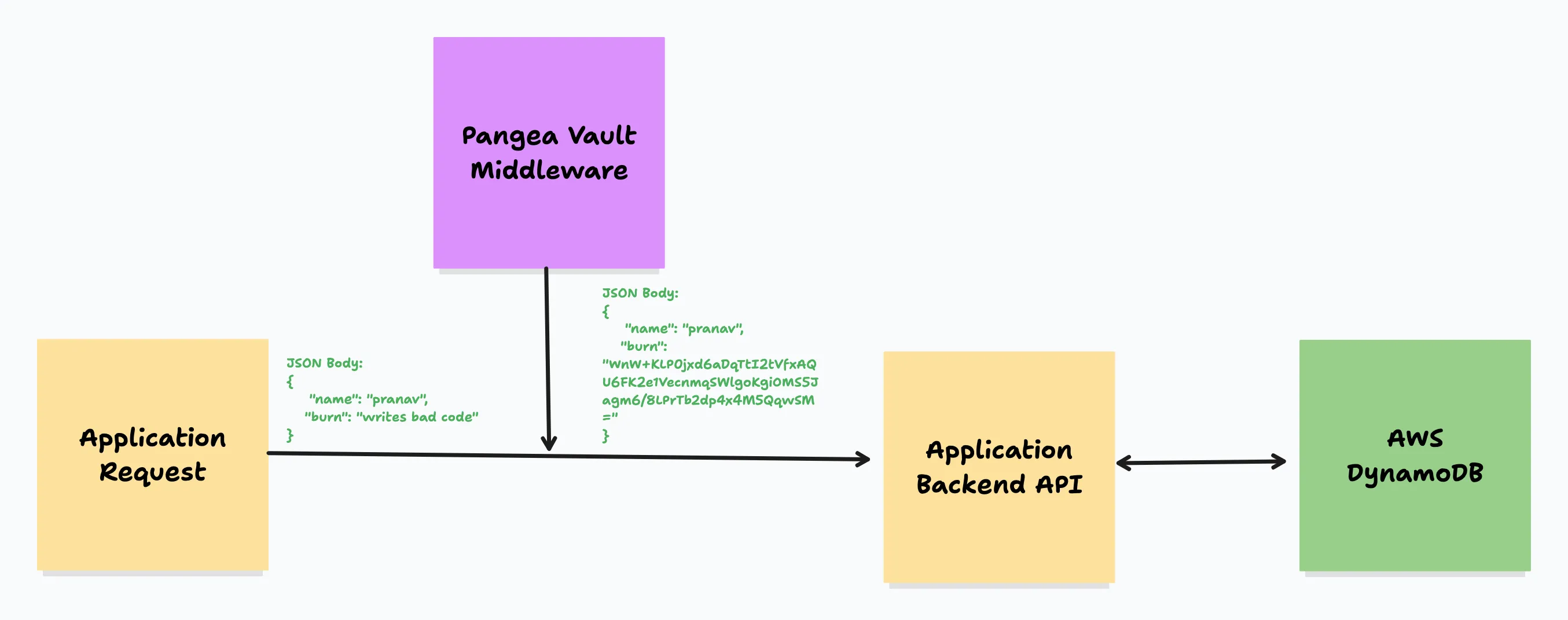
Notice how the middleware changes the “burn” text from the burn book into encrypted text while retaining the “name” in plaintext.
We will use this GitHub repository as an example to demonstrate how we can add encryption middleware to encrypt data in transit automatically. If you want to follow along, clone the repository and check out into the branch called “burnbook”
To perform data encryption in your application, you need to use a key manager. In this case we're using Pangea Vault since it has a robust set of APIs, so to follow along sign up for a free account on pangea.cloud run the setup based on the GitHub Readme. If you have any questions feel free to reach out to the Pangea Community.
Moving on, in this repo, look at the
src/pages/api/send-burn.ts
file. Notice how the exported API handler is wrapped with a middleware function encryptBodyInTransit
which is defined in src/utils/encrypt-middleware.ts
where the encrypt function looks something like this:As you can see here it takes in the request body and ONLY encrypts the sensitive “burn” field in the burn book entry while leaving all the other fields such as name, image, etc in plaintext. Thus, when the request body reaches the API handler, there is no change in the functionality of the API since the payload is already encrypted as necessary!
Finally notice, that to encrypt specific fields in the JSON payload, we use the
encryptStructured
API from Pangea Vault’s extensive API set allowing us to specify the fields we would like to encrypt. Similarly, we can do the same with decrypting data in transit.Finally, this can also be implemented on an AWS lambda or any place where you would like to secure data flowing through without having to significantly upgrade the API design and code. Thus, we’ve seen how adding encryption in transit in just a few lines of code can help secure user data without significant changes to the codebase.