Using Flux to Implement GitOps on AWS
GitOps is an effective way to achieve continuous deployment based on Kubernetes clusters while meeting enterprise-level requirements such as security, separation of privileges, auditability, and agility. These are the best practices for GitOps based on AWS EKS.
Amazon EKS-based Best Practices for GitOps
1.Deploy Cloud Infrastructure with IaC
1.1 Create a Project with CDK CLI
1.2 Create an EKS Cluster with EKS Blueprints
2.Deploy Flux CD on Amazon EKS Cluster
2.2 Prepare AWS CodeCommit Credentials
2.3 Install Flux on the Cluster
3.Deploy GitOps Workflow with Flux CD
3.3 Multi-Cluster Configuration
3.4 Deploy Microservices with GitOps Workflow
3.4.1 Adding the Microservices Repository Address
3.4.2 Adding CodeCommit Credentials
4.Image-Based Automated Deployment with GitOps Workflow
4.1 Defining the CodePipeline CI
4.2.1 Adding an image policy to the front-end of Git repository
4.2.2 Registering the Front-end of a Microservice Under Flux-repo
4.2.3 Configuring the access credentials for Amazon ECR
4.2.4 Setting image update policy
4.3.1 Update the Front-end Code
4.3.3 ECR Image Version Changing Confirmation
4.3.4 Verify Flux Image Information
4.3.5 Microservice Source Code Automatically Updates
4.3.6 Verify Pod Image Version
About | |
---|---|
✅ AWS experience | 300 - Advanced |
⏱ Time to complete | 90 minutes to complete |
💰 Cost to complete | Free tier eligible |
🧩 Prerequisites | AWS Account |
📢 Feedback | Any feedback, issues, or just a 👍 / 👎 ? |
⏰ Last Updated | 2023-05-19 |
Traditional CD | GitOps |
---|---|
Triggered by push events, such as code commits, timed tasks, manual, etc. | System constantly polls for changes |
Deployment of changes only | Declare the entire system for any deployment |
System might drift between deployments | The system will correct any drift |
Access to the deployment environment is a requirement | Deployment pipeline is authorized to run within system |
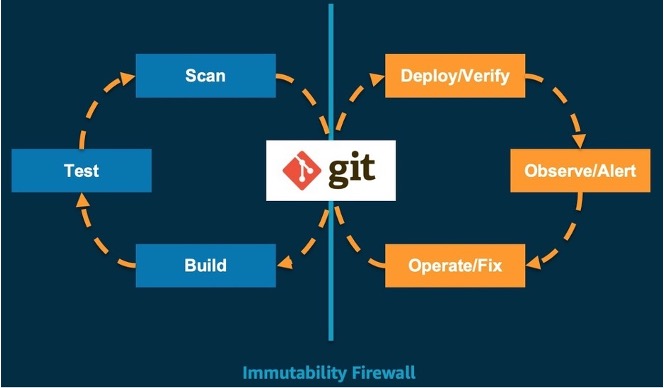

flux-repo
, the configuration repository for Flux CD, which is used to define Flux-related resources. The other is microservices-repo
, which saves microservice application configurations and deployment files. The third one is the source repository app-repo
for business services. In this post, a front-end project will be used as an example. We used AWS CodePipeline for continuous integration in the CI/CD pipeline, built and stored the docker image in Amazon ECR, and deployed the CD engine Flux as a pod in the Amazon EKS environment.- Coding engineers write code and push the final code to app-repo.
- Code changes in the app-repo trigger AWS CodePipeline.
- AWS CodePipeline edits and packages code, generates container images, and pushes them to the container image repository/Amazon ECR.
- The CD engine Flux, running in the EKS environment, regularly scans the ECR container image repository and pulls container image metadata for applications.
- The new container image address is automatically synced to the application deployment file stored in microservices-repo via git commit/push when a new version of the container image detected.
- Flux regularly pulls application configurations and deployment files from the Flux-repo. Since the Flux-repo repository references the microservices-repo, Flux checks the consistency of the workload running state of the cluster with the expectations described in the microservices-repo files. If there is any difference, Flux will automatically enable the EKS cluster to synchronize the differences to ensure that workloads run in the expected state.
- Deploy the cloud infrastructure using Infrastructure as Code (IaC)
- Deploy Flux CD on AWS EKS cluster
- Deploy GitOps workflow using Flux CD
- Implement automatic deployment based on images using GitOps workflow
cdk init
, which will create the folder structure and install the modules that TypeScript CDK project needs.quickstart
directory, and then run the following codes to install project dependencies.lib/quickstart-stack.ts
and add the following EKS Blueprints code.NoteWe suggest to customize the cluster parameters via clusterProvider and add plugins through the built-in addOns in EKS Blueprints.

NoteWe recommend defining infrastructure with CDK code and use pipelines to manage changes across multiple clusters that is also the best practice of GitOps.
cdk deploy
command to deploy the stack.- Initialized a CDK project using cdk init.
- Defined an EKS cluster quickly with EKS Blueprint while adding the AWS Application Load Balancer plugin.
So let's install Flux.
NoteWe recommend to dividing Flux-related resources into the infrastructure layer, cluster management layer, and application layer. We support multi-cluster deployment with Kustomization (base, overlays).
username
and password
in the command below with the HTTPS Git credentials for AWS CodeCommit.WarningEnable the image automatic update feature, add the--components-extra=image-reflector-controller,image-automation-controller
parameter when bootstrapping Flux. Otherwise, Flux will not install image-reflector-controller and image-automation-controller by default, and configurations such as automatic image updates will not take effect.
gotk-components.yaml
: defined the six controllers of Flux: helm, Kustomize, source, notification, image-automation, and image-reflector.gotk-sync.yaml
: the Git source of Flux, the Source Controller in the cluster monitoring code changes in the GitOps repository and making the corresponding changes.kustomization.yaml
: multi-cluster configuration.
gotk-components.yaml
, gotk-sync.yaml
, and kustomization.yaml
. The following is a summary of this section:- Flux client installation
- Creating an IAM user and CodeCommit credentials
- Installing Flux on an Amazon EKS cluster and enabling the image automatic update feature
NoteFlux regularly pulls the configurations and deployment files from the repository, and compares the current application load status of the cluster with the expected state described in the files. When differences are detected, Flux will automatically synchronize the differences to the EKS cluster, ensuring that workloads always run as expected.
- Best practices for microservices deployment (including examples of mistakes)
- Capabilities for Cross-platform deployment
- The advantages of continuous integration/deployment
- The complementary nature of DevOps and microservices
- A "real" testable application for various orchestration platforms

- Kustomize maintains application configuration across different environments through Base & Overlays.
- Kustomize uses Patch to reuse Base configuration and implementation, and resource reuse is achieved through the difference section between the Overlay description and the Base application configuration.
- Kustomize manages native Kubernetes YAML files, without requiring learning DSL syntax.

kustomization.yaml
:overlays/development/kustomization.yaml
file, without copying and modifying the existing complete-demo.yaml.NoteFlux will automatically merge base and overlays configurations according to the environment during service deployment. We recommend defining differential configurations across multiple environments, such as development, testing, and production. We do not favor the strategy of multi-repository and multi-branch strategy here, preferring instead to use different paths in a git repository to manage clusters in multiple environments. It helps simplify the process of code maintenance and merging, and which is also a Flux best practice.
https://git-codecommit.xxx.amazonaws.com/v1/repos/microservices-repo
.base/sock-shop/basic-access-auth.yaml
, and replace BASE64_USERNAME and BASE64_PASSWORD with the generated base64 encoding:flux get kustomizations -watch
and wait for Flux to update. When the READY status of all kustomizations is True, the deployment is complete.
- Sock Shop Introduction
- Learn a configuration management tool- Kustomize (base, overlays) and how to modify the microservice multi-cluster deployment
- Build a GitOps workflow and deploy microservices
buildspec.yml
file to the front-end project source code to define the CI process executed in the CodePipeline:weaveworksdemos/front-end
if any front-end code changed. The format of the image tag is [branch]-[commit]-[build number].--components-extra=image-reflector-controller,image-automation-controller
when you repeat the Flux bootstrap to enable it.- Register the image repository of the front-end microservice to allow Flux to periodically scan the ECR image repository correspondent to the front-end project.
- Configure the credentials for accessing the image repository. Flux needs the credentials to access ECR image repository to read the image information.
- Set the image updating policy. In most cases, we do not want all the image versions changes to trigger CD every time. Instead, we only want the specified branch (main) code changes to trigger CD. A special update policy is needed to fulfill this need.
ACCOUNT_ID
with your own ACCOUNT_ID).Note
Warning: The annotation$imagepolicy
, which is for locating, is mandatory. If Flux discovers the image version is changed, it will locate and modify the file content according to this annotation.
apps/overlays/development/sock-shop/registry.yaml
, and replace ACCOUNT_ID
with your own ACCOUNT_ID
.- Automatic authentication mechanism (image-reflector-controller retrieves credentials by itself, only applicable to: Amazon ECR, GCP GCR, Azure ACR)
- Regularly refreshing credentials (stored in the cluster through Secret) with CronJob
NoteWe used Amazon ECR to choose the automatic authentication mechanism, modifyclusters/dev-cluster/flux-system/kustomization.yaml
and add the--aws-autologin-for-ecr
parameter through patching. This approach is simpler and more efficient when compared to using CronJob to generate credentials regularly.
gitops/apps/overlays/development/sock-shop/policy.yaml
. The following rules match image versions such as master-d480788-1
, master-d480788-2
, and master-d480788-3
.gitops/apps/overlays/development/sock-shop/image-automation.yaml
. Flux's automatic image configuration will specify a Git repository for the application configuration, including branch, path, and other information.

weaveworksdemos/front-end
image version:


- Implementing the CI process through CodePipeline to achieve continuous integration of front-end code.
- Locating and modifying business configuration file by annotating Flux.
- Configuring Flux's image update policy to enable Flux to monitor the specific versions of images and complete automatic deployment.
- How to Gray-Release with security and increments for critical online-production-systems?
- How to improve the GitOps Key Management on cloud when Sealed Secrets introduced additional private key management requirements.
- How to Coordinate manage for Coding of IaC and EKS GitOps
- How to develop Kubernetes manifests (YAML) more efficiently
Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.