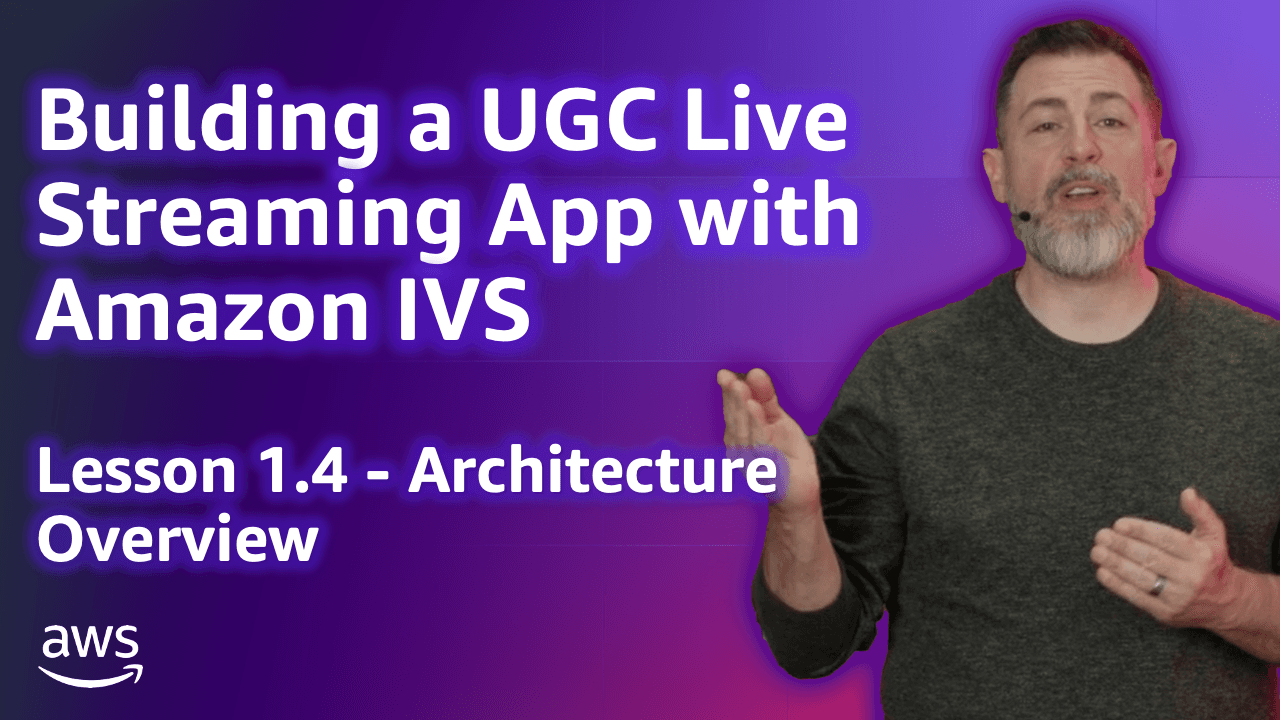
Build a UGC Live Streaming App with Amazon IVS: Architecture Overview (Lesson 1.4)
Welcome to Lesson 1.4 in this series where we're looking at building a web based user-generated content live streaming application with Amazon IVS. This entire series is available in video format on the AWS Developers YouTube channel and all of the code related to the sample application used in this series can be viewed on GitHub. Refer to the links at the end of the post for more information.
Todd Sharp
Amazon Employee
Published Dec 12, 2023
This lesson provides an architectural overview of the StreamCat UGC application.
The StreamCat application architecture is divided into two categories: a collection of pre-built resources that are deployed via the AWS Cloud Development Kit (CDK), and several resources that are created on-demand by the application itself via the AWS SDK for JavaScript (v3) when users register a new account in the application. To give you an idea what this looks like, here is an overall architecture diagram that we'll break down further in this lesson.
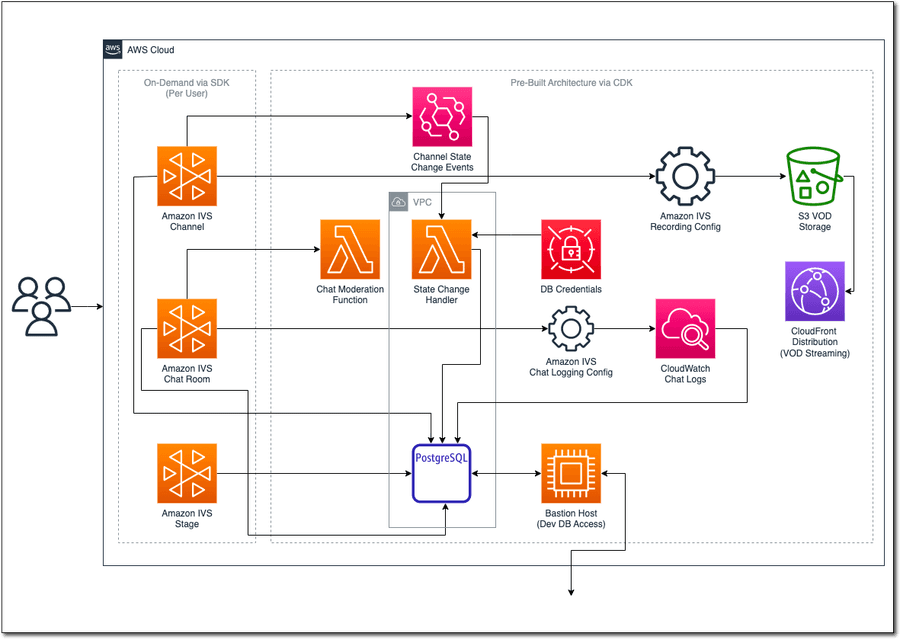
The first category we'll focus on is the pre-built resources.
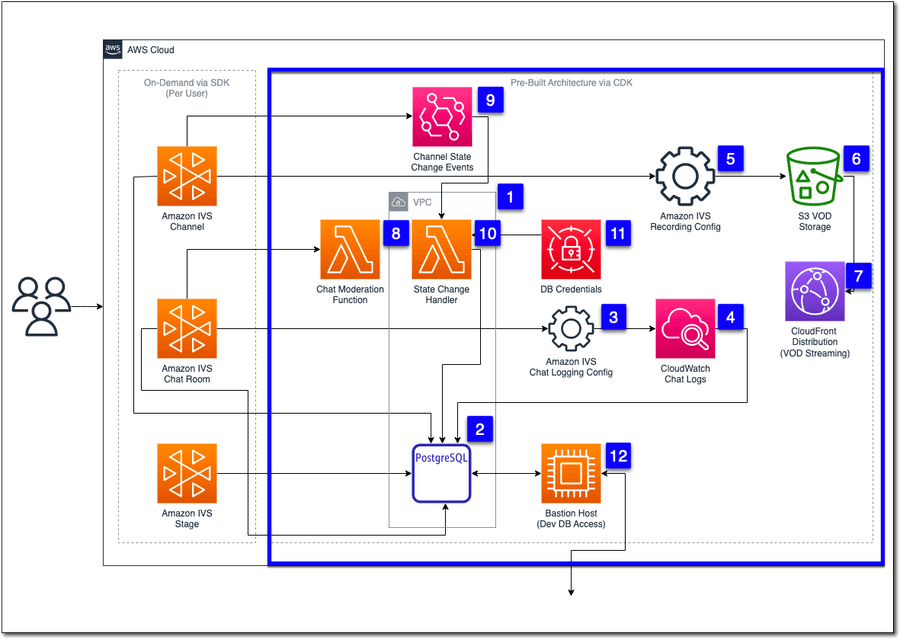
Let's break this down and see what each resource does.
1. VPC
A virtual private cloud is used to restrict access to the Amazon Relational Database Service (Amazon RDS) database instance.
An interface endpoint is added to the VPC to allow the AWS Lambda function that we'll create later on the ability to utilize the AWS Parameters and Secrets Lambda Extension to retrieve database credentials without the need for an SDK.
A security group is created to allow ingress for the database port within the VPC, as well as SSH access.
2. PostgreSQL DB Instance on Amazon RDS
This database instance is used to persist all of the user data for the application. This instance is associated with the VPC we created above, and placed in a private, isolated subnet.
3. Amazon IVS Chat Logging Configuration
To log chat messages, a reusable chat logging configuration is created. This configuration will be associated with every chat room and will log all chat events to the CloudWatch log group created in #4 below.
4. CloudWatch Log Group
This log group will contain all chat logs that will be created via the chat logging configuration (#3 above).
5. Amazon IVS Recording Configuration
This configuration will be associated with every Amazon IVS channel and ensures that live streams are recorded to Amazon Simple Storage Service (Amazon S3) and thumbnails are periodically generated.
6. Amazon S3 Bucket
This bucket that will contain the video on-demand (VOD) recorded live stream media and assets.
7. Amazon CloudFront Distribution
This distribution allows the application to expose and serve the assets stored in the VOD bucket.
8. Chat Moderation Lambda Function
This function is associated with every Amazon IVS chat room that will be created, and provides basic automated moderation with profanity filters for all chat rooms. We will look at the Lambda function code in a subsequent lesson.
9. Amazon EventBridge Rule
This EventBridge rule is triggered automatically when the stream or recording state changes on our user's Amazon IVS channels. This will allow the application to update the channel's state, and persist information related to VOD assets so that it can be retrieved for playback later on.
10. Stream State Change Lambda Function
A Lambda function that handles persisting the Amazon IVS channel and recording state. This function is triggered automatically via the EventBridge rule created in #9.
11. AWS Secrets Manager for Database Credentials
A manually created secret will contain the database user credentials.
12. Bastion Host
An Amazon Elastic Compute Cloud (Amazon EC2) instance that is used as a bastion host to allow our local development environment to access the RDS instance.
When a user registers for a new account, the following set of resources will be created via the AWS SDK for JavaScript (v3).
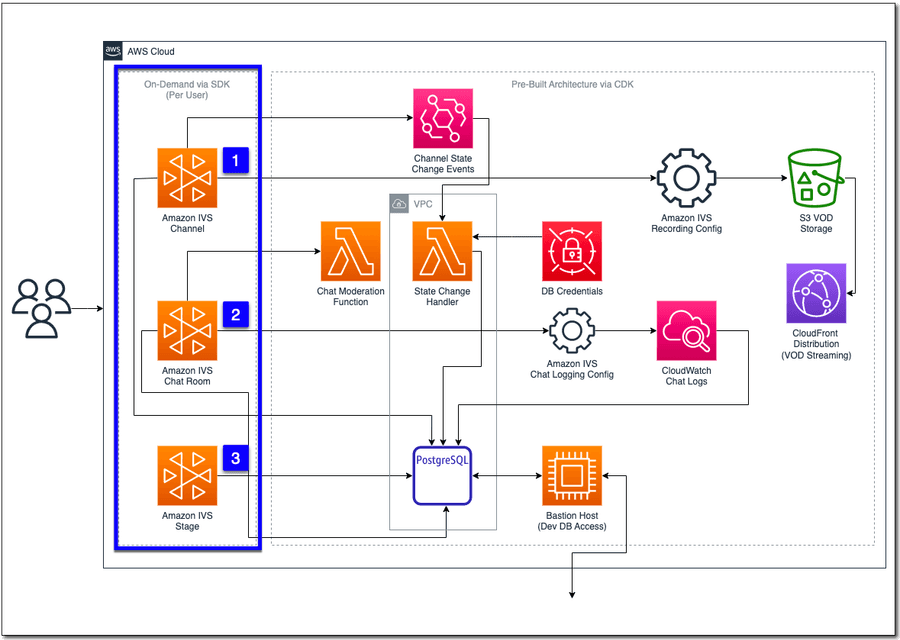
1. An Amazon IVS Channel
2. An Amazon IVS Chat Room
3. An Amazon IVS Stage
In this lesson, we learned about the pre-built and on-demand resources that are utilized by the StreamCat application. In the next lesson, we'll see an overview of the application's schema.
Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.