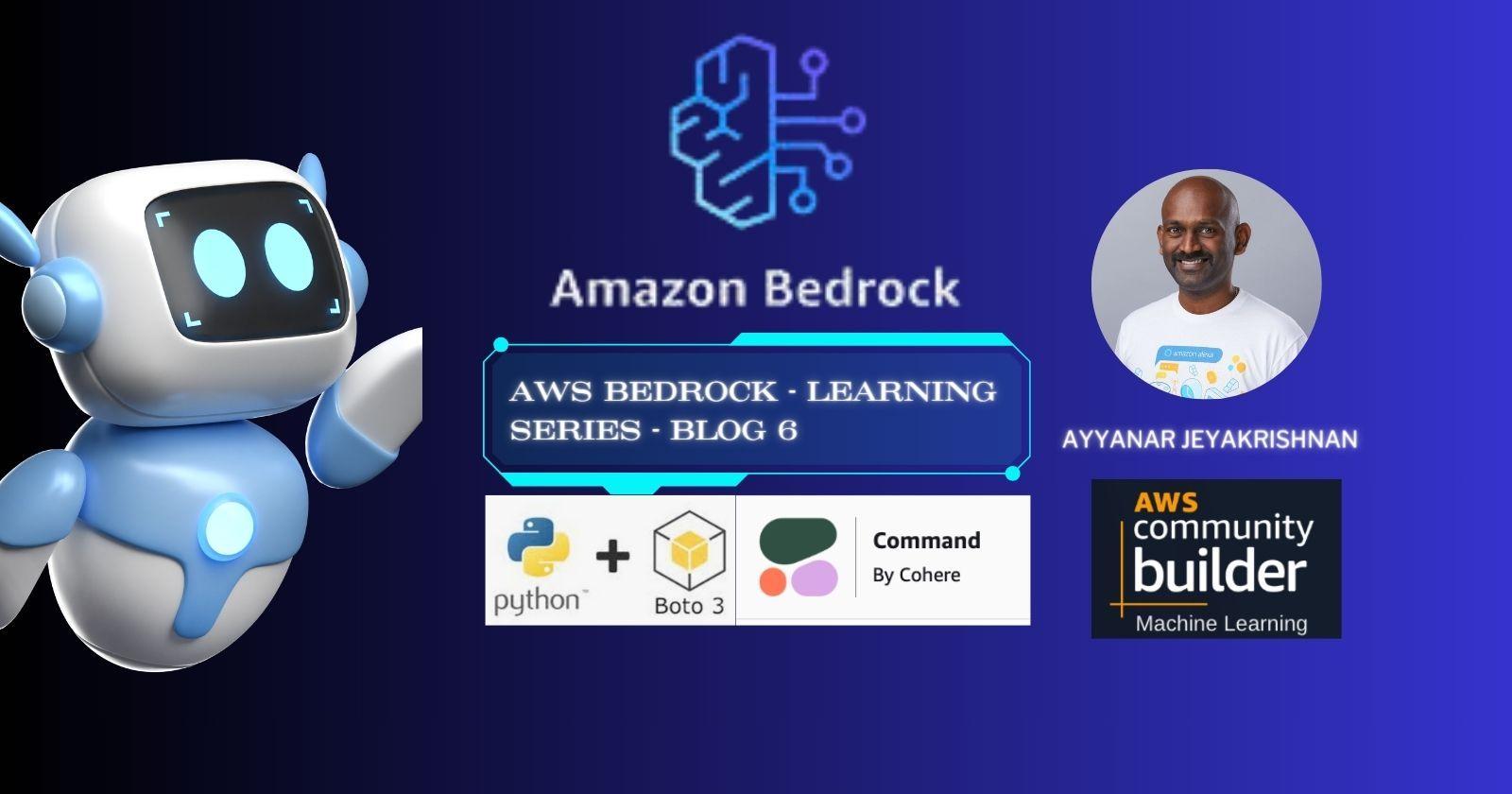
AWS BedRock - Boto3 Demo - Cohere Model
Cohere offers a range of text generation and representation models designed for diverse business applications. The Command model, with 52 billion parameters, excels in tasks such as chat, text generation, and summarization. A lighter version, Command Light, with 6 billion parameters, provides a more resource-efficient alternative.
Published Dec 13, 2023
Last Modified Mar 11, 2024
https://github.com/jayyanar/learning-aws-bedrock/blob/main/blog6-Cohere/Bedrock_Cohere.ipynb
I am using vscode local environment with AWS Credential configured.
Cohere's flagship text generation model is known as Command. It is designed to seamlessly respond to user commands and provide immediate utility in real-world business scenarios. The model excels in various applications, including summarization, copywriting, dialogue, extraction, and question answering.
Key Model Features Command boasts attributes such as text generation and precise instruction following.
Title: "Quantum Quest"
Once upon a time, in a world where the laws of physics were bent and broken, a new breed of superheroes emerged. These heroes were not born with superhuman abilities, but rather, they were ordinary people who had harnessed the power of quantum energy.
Our protagonist, Jake, was a brilliant scientist who had dedicated his life to unlocking the secrets of the quantum realm. He had always been fascinated by the potential of the microscopic world, and he dreamed of discovering a way to manipulate the energy that existed there.
One day, while working in his lab, Jake made a groundbreaking discovery. He developed a device that allowed him to enter the quantum realm and manipulate the energy there. At first, he was hesitant to use his newfound power, but when he witnessed a crime in progress, he knew he had to act.
Armed with his quantum device, Jake transformed into a new superhero, Quantum Quest. He leaped into action, using his control over quantum energy to shrink himself down to the size of an ant and battle the criminals.
As Quantum Quest, Jake encountered many challenges and enemies. He faced off against powerful villains who sought to exploit the quantum realm for their own gain, and he fought to protect the world from the dangers of quantum technology.
As he continued to use his powers, Jake discovered that he could not only shrink himself, but also grow to an enormous size, and even teleport short distances. He used his powers to defend the innocent and fight for justice, becoming a beloved hero in the process.
However, Jake's journey was not without its challenges. He struggled to balance his superhero duties with his personal life, and he often felt the weight of the responsibility he had taken on. Despite this, he remained dedicated to his mission, and he continued to fight for what was right.
In the end, Quantum Quest emerged as a true hero, a testament to the power of science and the strength of the human spirit. He had proven that anyone, even an ordinary person, could make a difference and become a force for good in the world.
The End.
Would you like me to expand on the story or provide a different ending?
Cohere's Command-Light is a generative model optimized for instruction-like prompts, offering an exceptional balance of quality, cost-effectiveness, and low-latency inference. It excels in various applications, including summarization, copywriting, dialogue, extraction, and question answering.
The model is characterized by its text generation capabilities and adeptness at following instructions.
Cohere's Embed is a model designed to transform text into numerical vectors, facilitating comprehension by various machine learning models. Widely utilized in advanced generative AI applications, Embed plays a crucial role in deciphering user inputs, search results, and documents with nuanced understanding.
Its applications span semantic search, retrieval-augmented generation (RAG), classification, and clustering, and it is characterized by its 1024-dimensional vector representation."
Text within this block will maintain its original spacing when published [0.40429688, -0.38085938, 0.19726562, '...', 0.2109375, 0.012573242, 0.18847656]
Practice Word Embedding using Cohere API - Step by Step Instruction - Follow Below notebook
Practice Prompt Engineering using Cohere API - Step by Step Instruction - Follow Below notebook
Thanks for Reading the Post -- Share your Feedback and Comments -- Stay Tuned for Next Blog