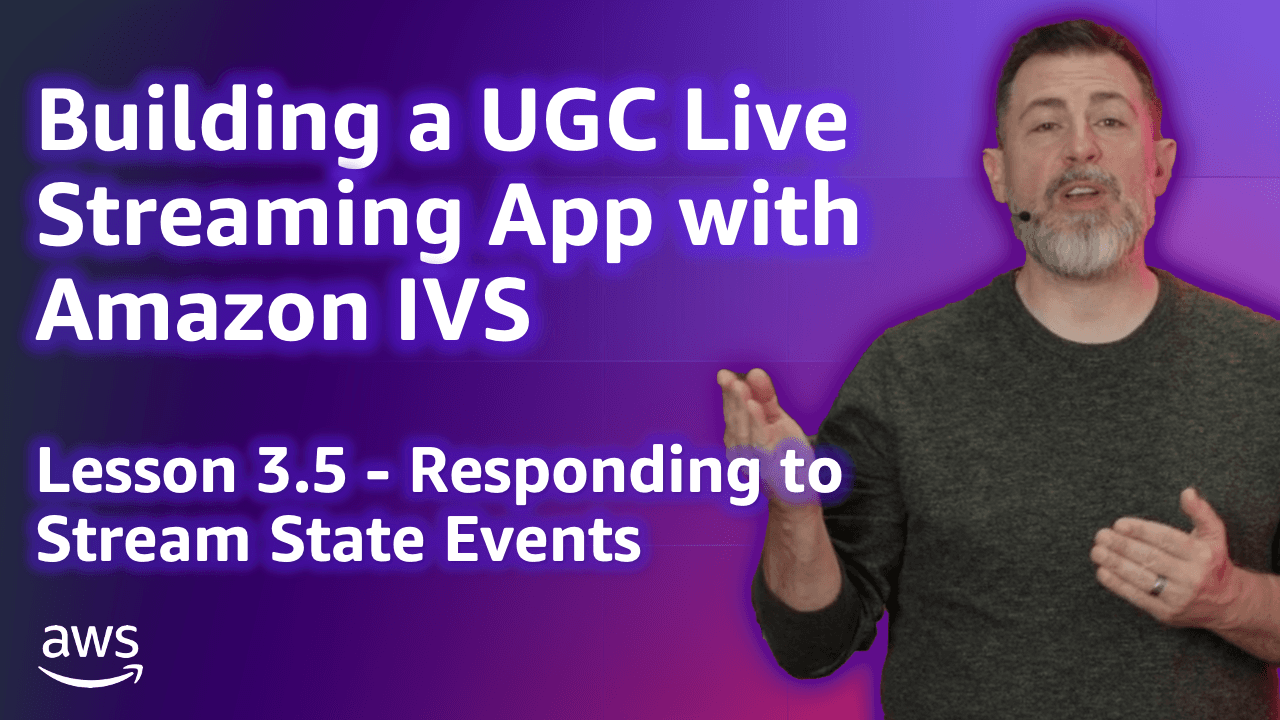
Build a UGC Live Streaming App with Amazon IVS: Responding to Stream State Events (Lesson 3.5)
Welcome to Lesson 3.5 in this series where we're looking at building a web based user-generated content live streaming application with Amazon IVS. This entire series is available in video format on the AWS Developers YouTube channel and all of the code related to the sample application used in this series can be viewed on GitHub. Refer to the links at the end of the post for more information.
Todd Sharp
Amazon Employee
Published Dec 14, 2023
In this lesson, we'll look at how StreamCat responds to stream state events to update a channel's state or publish a VOD asset for a previous broadcast. If you recall from lesson 1.4, StreamCat uses an Amazon EventBridge rule to listen for various state change events related to our Amazon IVS channels. This EventBridge rule triggers an AWS Lambda function, and that function persists and updates database records as necessary. In this lesson, we'll focus on how StreamCat handles the following events:
- Stream Start
- Stream End
- Recording Start
- Recording End
Let's look at how StreamCat responds to the various events.
If you recall lesson 1.4, the Amazon EventBridge rule that is created for StreamCat listens for the following event types:
The event that is passed to our AWS Lambda function that is triggered by this rule varies slightly depending on the specific event that triggered it, but there are several values that are common between the various event types.
Here's a sample 'Stream Start' event:
And here's a 'Recording End' event:
When a stream begins on an Amazon IVS channel, StreamCat:
- Updates the
Channel
to setisLive
totrue
- Inserts a new
Stream
object in the database.
💡 Note: Refer to lesson 1 to learn more about StreamCat's schema and the relationship betweenChannel
andStream
.
When the triggering event is either
Stream Start
or Stream End
, we can use the event.detail.event_name
property to determine the proper value to set Channel.isLive
.Next, we can either insert or update a
Stream
record depending on whether or not one exists for the given stream_id
. This method captures the title
, and category_id
of the Channel
when the broadcast beganWhen a recording starts, StreamCat either inserts or updates the existing
Stream
to persist the recording_started_at
, the recording_path
, and the Channel
's title
at the time the recording began to the VOD recording for the given stream.💡 Note: Technically, theStream
should already exist (since it's created on theStream Start
event), but sometimes these events fire nearly simultaneously, so it's best to handle the possibility that aStream
might not exist yet when theRecording Start
event is handled.
When the
Recording End
event fires, StreamCat finalizes the Stream
record to capture the recording_end_date
, recording_duration_ms
, and recording_path
.In this lesson, we saw how StreamCat persists stream state change events to the database. These state changes give StreamCat several methods to help users discover content, such as listing currently 'live' channels, and searching for a past broadcast. In a future lesson, we'll see how chat messages are also persisted to the database when a stream ends.
This concludes lesson 3. In this lesson, we learned many concepts related to broadcasting low-latency live streams with the Amazon IVS SDK, as well as how StreamCat captures stream state change events. In lesson 4, we'll start to look how StreamCat enables broadcasting for real-time live streams.
Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.