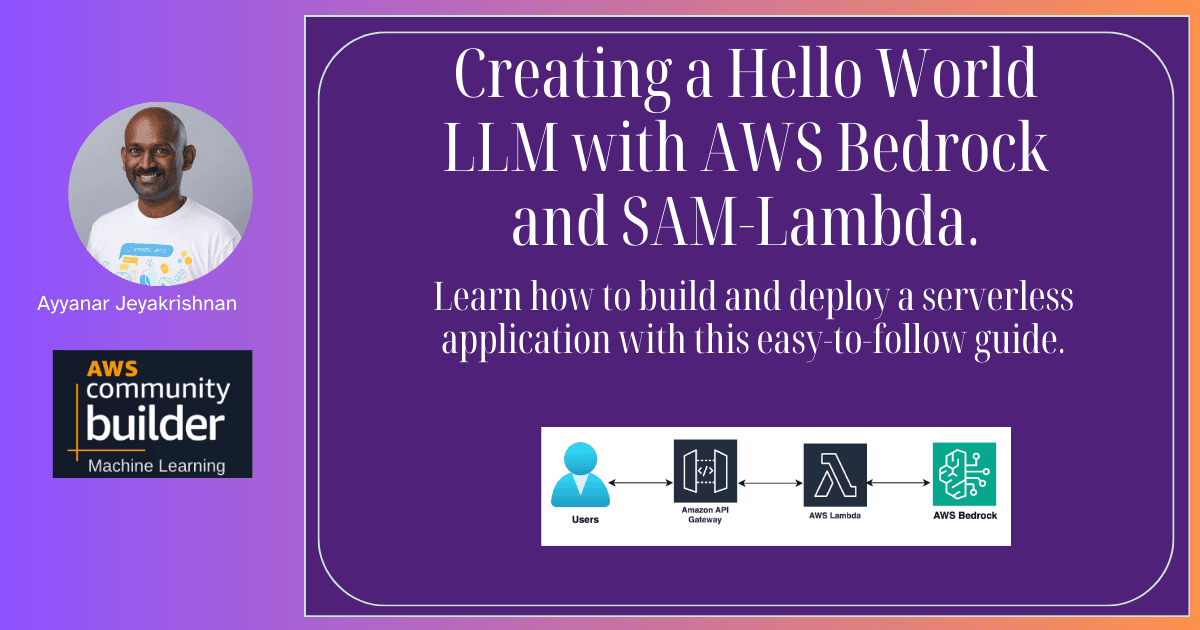
Creating a Hello World LLM with AWS Bedrock and SAM (Lambda and API Gateway)
A Step-by-Step Guide to Creating a Hello World LLM with AWS Bedrock and SAM (Lambda and API Gateway)
Published Jan 6, 2024
Last Modified Mar 11, 2024
Embarking on the journey of serverless architecture often begins with a simple "Hello World Bedrock" application, providing a foundational understanding of key concepts and SAM framework and AWS Bedrock - Serverless GenAI as a Service.
Whether you're a newcomer to serverless development or looking to explore the capabilities of AWS Bedrock, this step-by-step demonstration will equip you with the knowledge and confidence to kickstart your serverless projects. Join us as we unravel the intricacies of Bedrock and SAM, uncovering the seamless integration of Lambda functions and API Gateway for a robust serverless architecture. Let's embark on this hands-on journey together, where "Hello World" becomes the gateway to learning of AWS Bedrock and SAM.
Note: I am using MAC, So I am using Brew - You can follow below documentation to update the MAC : https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/manage-sam-cli-versions.html
You can download from Github and Perform the Sam Deploy.

Enhance the security of your Bedrock application by updating the IAM Role access policy. A sample policy is provided to serve as a reference, offering insights into best practices for securing your serverless architecture.
--> Create IAM Policy (Inline or Custom Policy) and update to the IAM Role
Verify Via API Gateway for Bedrock Response from Lambda

Ensure a smooth deployment of your serverless application by verifying the CloudFormation template. This section guides you through the necessary steps to validate your configuration before launching your application to the AWS cloud.
--> Select 1 --> AWS Quick Start Templates
--> Select 1 --> Hello World Example
--> Select 18 --> python3.12
--> Select 1 --> Zip
Based on your selections, the only dependency manager available is pip. We will proceed copying the template using pip.
Would you like to enable X-Ray tracing on the function(s) in your application? [y/N]: n
Would you like to enable monitoring using CloudWatch Application Insights?
For more info, please view https://docs.aws.amazon.com/AmazonCloudWatch/latest/monitoring/cloudwatch-application-insights.html [y/N]: n
Would you like to set Structured Logging in JSON format on your Lambda functions? [y/N]: y
Structured Logging in JSON format might incur an additional cost. View https://docs.aws.amazon.com/lambda/latest/dg/monitoring-cloudwatchlogs.html#monitoring-cloudwatchlogs-pricing for more details
--> Give a Project Name
Update the requirement.txt for Boto3 Update.
Path: E ~/simple-bedrock-lambda-app/hello_world/requirements.txt
Make Code Changes Needed from VSCode and Sync Using SAM CLI
Path: cat ~/simple-bedrock-lambda-app/hello_world/app.py
Validate and Deploy the SAM Template
Patience is key! Understand the importance of waiting for the deployment process to complete successfully. This section provides insights into monitoring the deployment progress to ensure a seamless and error-free execution.
Verify the Deployment from Cloudformation

Update the IAM Role for Bedrock Role Access Policy for Lambda Deployed
Enhance the security of your Bedrock application by updating the IAM Role access policy. A sample policy is provided to serve as a reference, offering insights into best practices for securing your serverless architecture.
--> Create IAM Policy (Inline or Custom Policy) and update to the IAM Role
Verify Via API Gateway for Bedrock Response from Lambda

Any Changes Needed from VSCode and Sync Using SAM Code
Explore the integration of Visual Studio Code (VSCode) with SAM, allowing for efficient coding and synchronization. Learn about any additional changes needed within your development environment and how to sync them using SAM code.
Check the Bedrock Cloudwatch Dashboard - Invocation
Feel Free to check on Dashboard

Check the Bedrock Cloudwatch Dashboard - Token Count

Log Insights for Cloudwatch Logs Information
--> Go to Log Insights --> Select the Cloudwatch Log Group --> <Where the logs Bedrock Logs Stored> --> Check on my Blog1 for reference --> Paste the below Query (You can custom the queries also via "Query Generator")
fields input.inputBodyJson.inputText,input.inputBodyJson.textGenerationConfig.maxTokenCount,input.inputBodyJson.textGenerationConfig.temperature,input.inputBodyJson.textGenerationConfig.topP,input.inputContentType,input.inputTokenCount,modelId,output.outputBodyJson.results.0.completionReason,output.outputBodyJson.results.0.outputText,output.outputBodyJson.results.0.tokenCount,output.outputTokenCount,region

-- Thanks for Reading - Share your comments and feedback
Newsletters: https://www.dataopslabs.com/
Youtube Channel: https://www.youtube.com/channel/UC5oU2OucjpaPT7OJWpZy49g