
How To Deploy AWS ECS Fargate service using AWS CDK
In this article, we will deploy a sample web application to an AWS ECS Fargate service using AWS Cloud Development Kit (CDK)
Published Jan 10, 2024
This article will guide you through the process of deploying Fargate using AWS CDK. According to the official AWS documentation, AWS Fargate is described as a serverless compute engine that operates on a pay-as-you-go model. It enables you to concentrate on developing applications without the need to handle servers directly.
In simpler terms, Fargate allows you to execute containers on ECS in AWS without the burden of server management. This simplifies the overall management process, as there is no need to concern yourself with selecting server types or determining when to scale the cluster. When utilizing Fargate, containers operate seamlessly without the necessity for EC2 instances.
Fargate encompasses several distinctive features that set it apart as a unique service. Let's delve into each feature in detail:
- Load Balancing:
- When initiating a Fargate service, if you specify the IP address, it automatically registers with the Load Balancer.
- This feature ensures efficient distribution of incoming traffic among multiple Fargate instances, enhancing the service's reliability and availability.
- Networking:
- All Fargate tasks operate within a Virtual Private Cloud (VPC).
- Fargate supports AWS VPC networking mode, providing a secure and isolated environment for the service.
- Utilizing VPC networking allows for the segregation of services, enabling better control and management.
- Configurability Management:
- Fargate offers extensive configuration options, providing flexibility in adjusting CPU and memory combinations to match specific requirements.
- The configurable range spans from 2 GB to 8 GB, allowing users to fine-tune the configuration at any given time.
- This adaptability ensures that the service can be tailored to accommodate varying workloads without compromising performance.
- Container Registry Support:
- Fargate seamlessly integrates with Container Registries, such as Amazon Elastic Container Registry (ECR).
- The task execution role in Fargate facilitates easy authentication, enabling the pulling of container images from ECR.
- Additionally, Fargate supports connections to Docker Hub, offering flexibility in managing container images from different sources.
In summary, Fargate's features encompass efficient load balancing, secure networking within VPC, extensive configurability, and seamless support for container registries, collectively contributing to its appeal as a versatile and user-friendly compute engine.
- AWS Account:
- To proceed with the setup, ensure that you have a valid AWS account. This is essential for accessing and utilizing AWS services.
- Configured AWS CLI:
- Make sure that the AWS Command Line Interface (CLI) is properly configured on your system. The AWS CLI is a powerful tool that allows you to interact with various AWS services through the command line.
- NPM Package Manager:
- Install the Node Package Manager (npm) on your system. This package manager is necessary for installing the AWS Cloud Development Kit (CDK), which plays a key role in deploying resources on AWS.
- Node.js Installed (Version >14.0.0):
- Ensure that Node.js is installed on your machine, and the version is greater than 14.0.0. Node.js is required to run JavaScript applications, and having a version above 14.0.0 ensures compatibility with the CDK and its dependencies.
Lets start with steps now.
I will be using my own AWS Account for this article. I intend to execute all tasks using the AWS Cloud9 IDE environment.
AWS CLI Configured as shown below
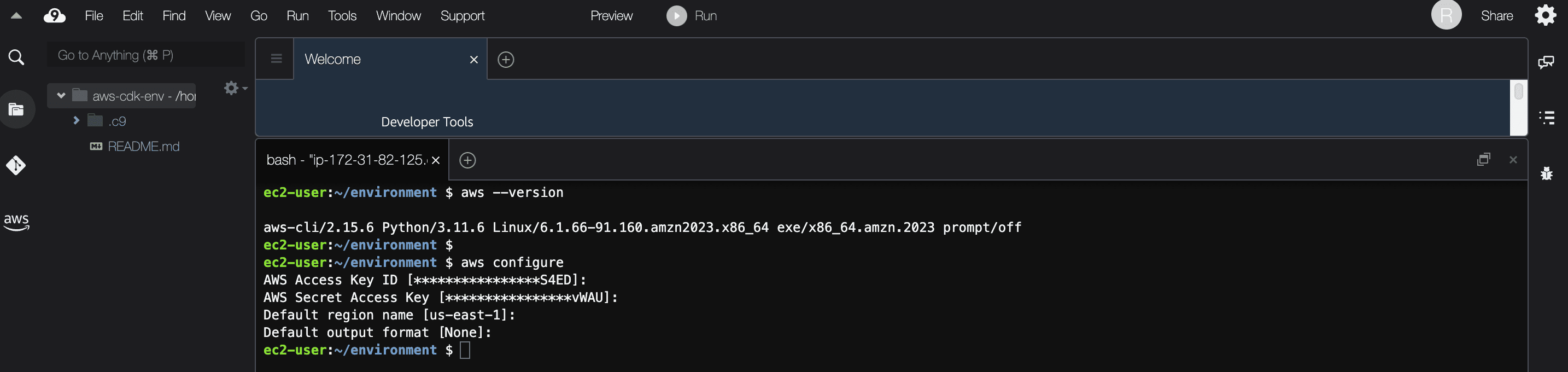
Node.js is installed & NPM Package Manager (NPM) comes by defualt with Node.js as shown below

To install AWS CDK run the below command
Installing AWS CDK on AWS Cloud9 environment is unnecessary since the AWS Cloud9 instance already comes prebuilt with it.
Check the AWS CDK version using the below command
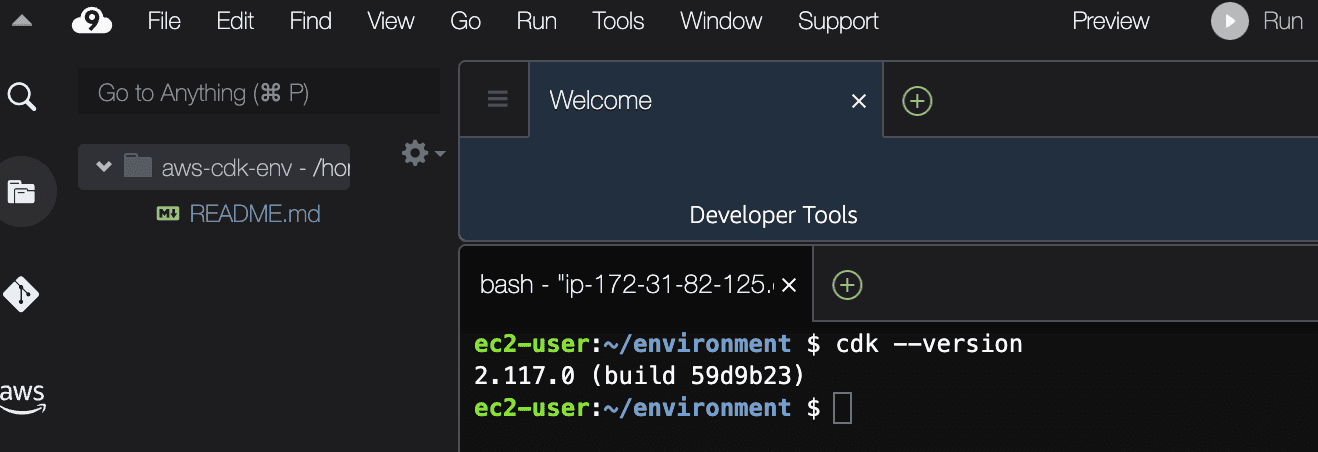
To work with the AWS CDK, you must have an AWS account and credentials and have installed Node.js and the AWS CDK Toolkit. See AWS CDK Prerequisites.
You also need TypeScript itself (version 3.8 or later). If you don't already have it, you can install it using
npm
.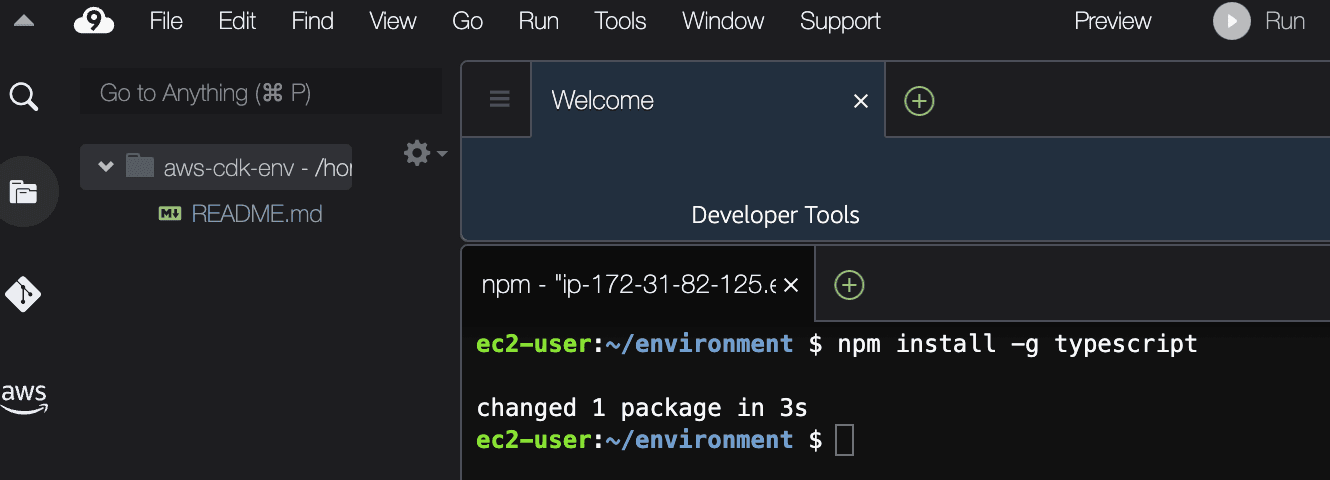
Note
If you get a permission error, and have administrator access on your system, try
sudo npm install -g typescript
.Keep TypeScript up to date with a regular
npm update -g typescript
.The following commands can be used to create the necessary folder structure:
Now, within the newly created folder, the following files need to be generated:
a. cdk.json file:
- Purpose: This file instructs the CDK CLI on how to execute the CDK code and keeps track of feature flags for safe deployment of new features and bug fixes.
b. package.json file:
- Purpose: This npm module manifest includes information about the application, such as its name, version, dependencies, and build scripts.
- Explanation: The file includes specifications for the application, such as its name, description, scripts, author, license, and dependencies.
c. main.ts file:
- Purpose: This TypeScript file contains the main code for the implementation, creating a VPC and instantiating the Fargate Service with a cluster and image.
- Explanation: The code imports necessary libraries, creates a VPC and Fargate cluster, and pulls an image from the registry to instantiate the Fargate service.
d. tsconfig.json file:
- Purpose: This file specifies the root files and compiler options required to compile the TypeScript project.
- Explanation: This file outlines compiler options for the TypeScript project, detailing how the project should be compiled.
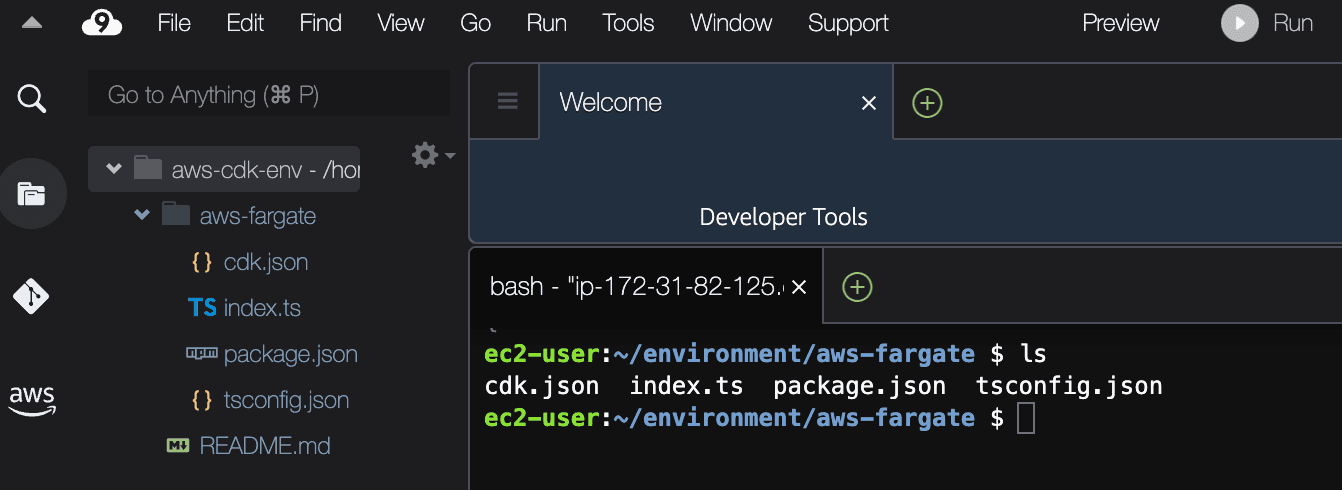
These files collectively set up the necessary structure and configuration for the demonstration. To proceed with Fargate deployment, let's move forward.
To initiate the deployment of the mentioned stack, execute the following command in the directory where the files are located:
This command will install all the dependencies specified in the stack file.

Following the dependencies installation, proceed with the following command:
This command prepares the environment by generating the CloudFormation template in AWS. The template encapsulates all the resources to be created during the deployment process.

The output indicates the successful bootstrapping of the environment.
After confirming the changes, proceed to deploy the application by running the following command:
This command initiates the deployment of the Fargate service.


The deployment process will unfold, and upon completion, you will observe the deployment confirmation:

The stack has been successfully deployed.
Here is the output reflecting the stack created on AWS.
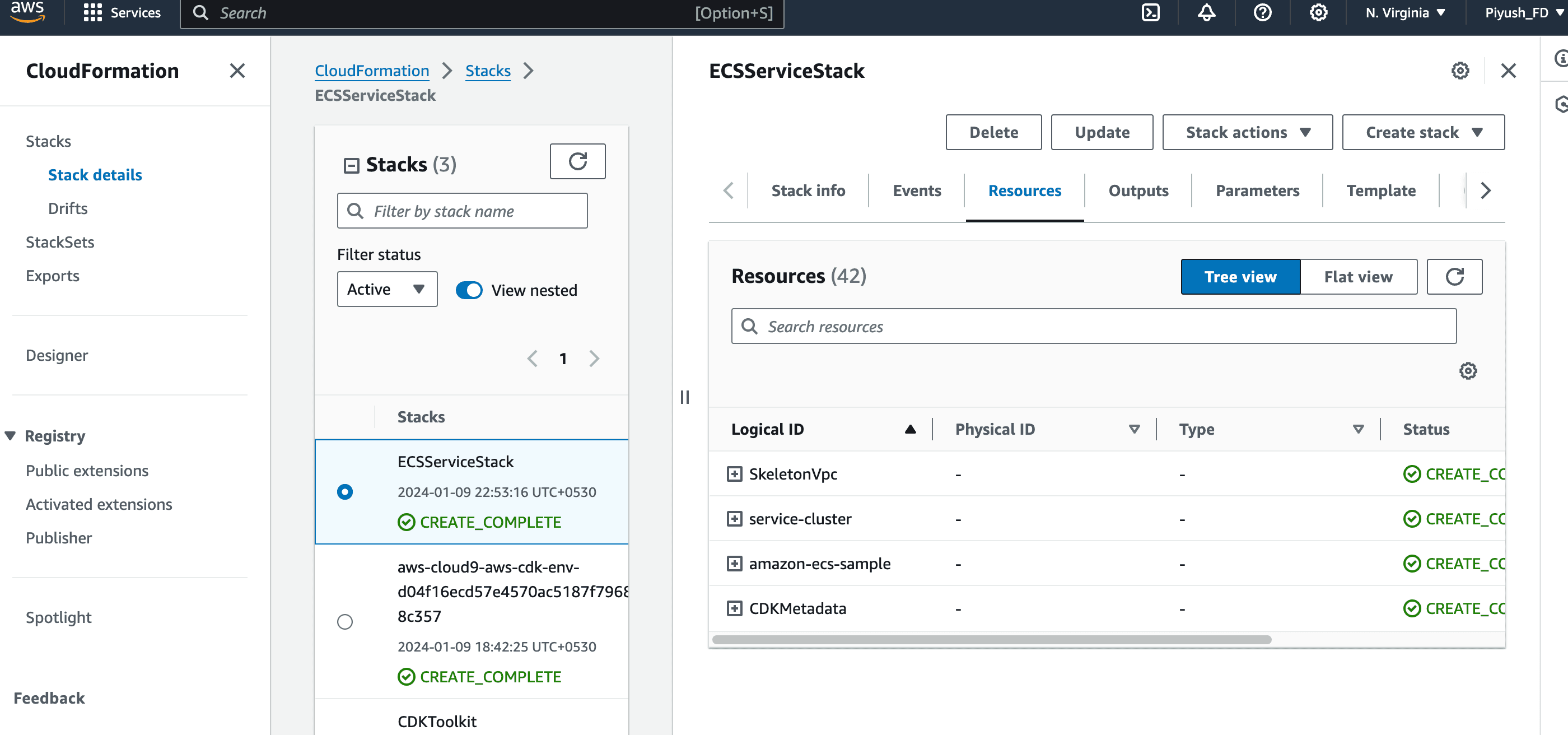

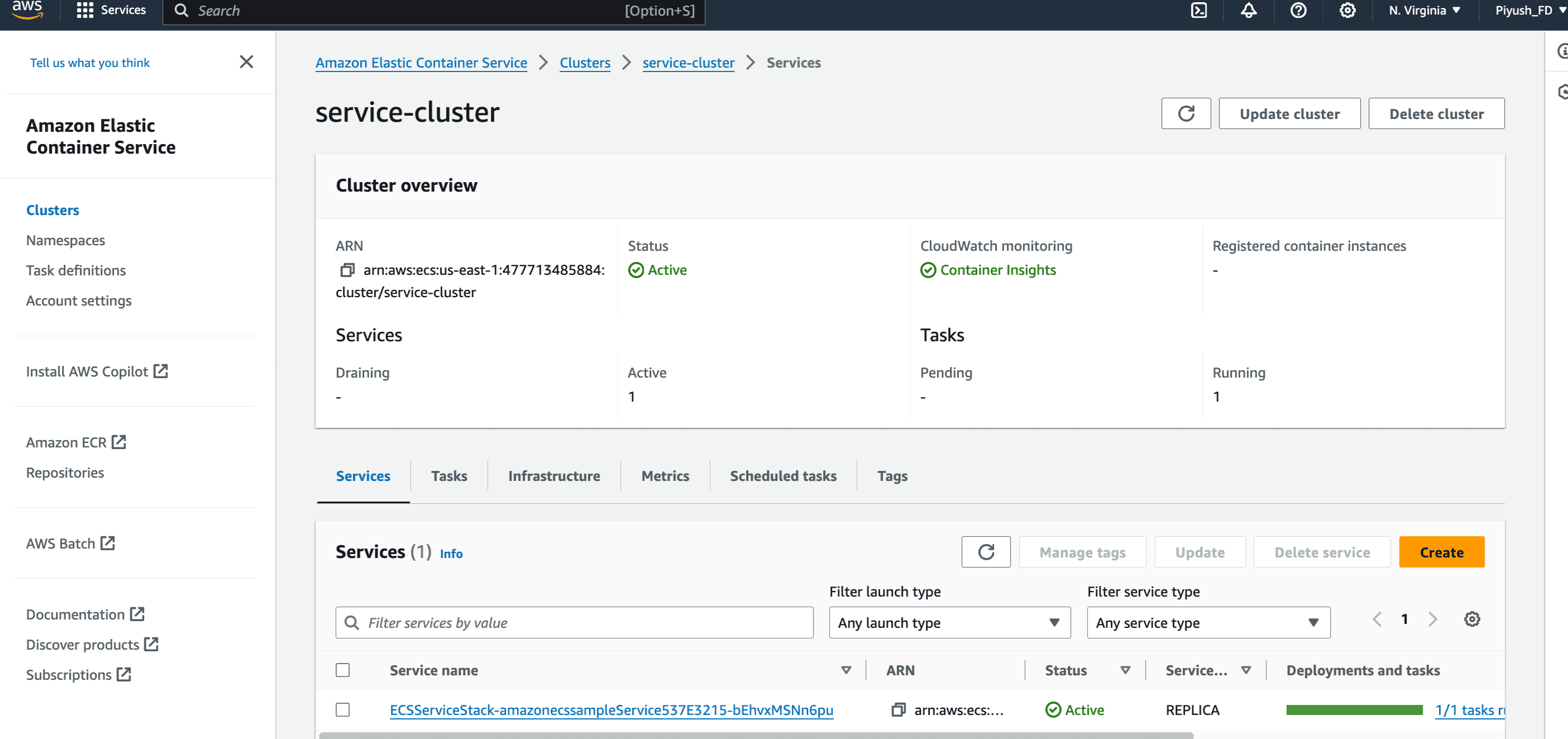
Sample Web application has been deployed successfully and is accessible via application load balancer as shown below

NOTE: Remember to execute the "cdk destroy" command afterward, as the resources in use will incur high costs. This ensures proper resource cleanup and cost management.
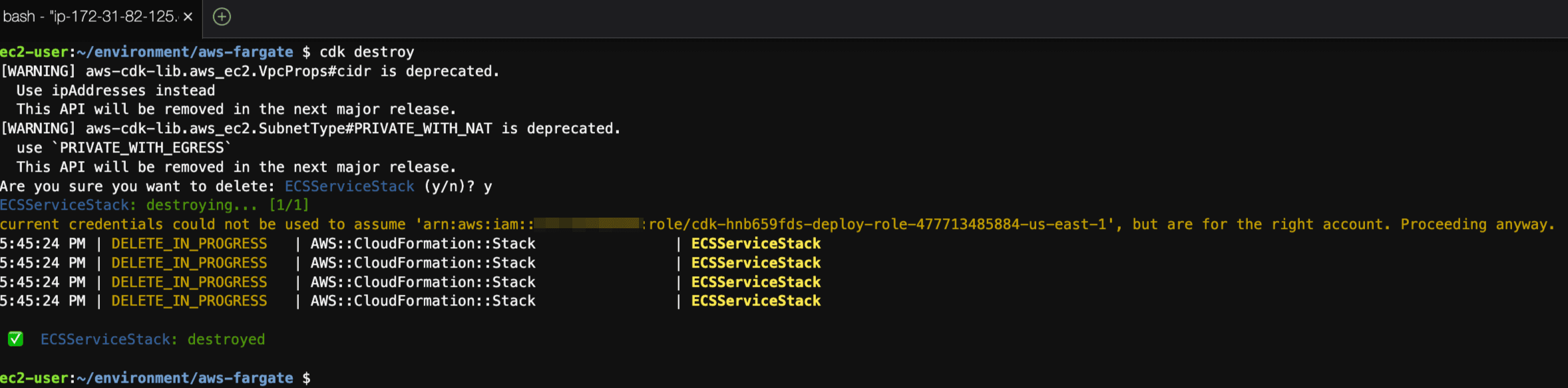
- Automated Load Balancer Configuration:
- The deployment automatically configures a load balancer, streamlining the process of distributing incoming traffic.
- Security Group Creation and Load Balancer Integration:
- A security group is created, and the load balancer is seamlessly integrated with it to enhance network security.
- ECR Permission Setup:
- Permissions for Amazon Elastic Container Registry (ECR) are established, facilitating secure authentication and image retrieval.
- Prevention of Automatic Instance Deletion:
- Safeguards are implemented to prevent instances from being automatically deleted, ensuring stability and control over resources.
- Automated Scaling:
- The demonstration showcases the achievement of automatic scaling, allowing the system to dynamically adapt to varying workloads for optimal performance.
In conclusion, this discussion demonstrated the ability to execute containers without the necessity of server management. Furthermore, the utilization of Fargate streamlined the entire operation, providing automation. It's noteworthy that Fargate seamlessly integrates with both Elastic Container Service and Elastic Kubernetes Service.
Hope you enjoyed it.
This concludes the present article. Wishing you all a wonderful day! Stay tuned for more updates. Remember to show your support by liking and sharing this post on your social networks. I'll continue to share insights within this fantastic AWS community platform. Please make sure to follow for future content!Let's build a community that thrives on the exchange of knowledge, where collaboration fuels innovation. Join me in this endeavor, and together, let's shape the future of technology.