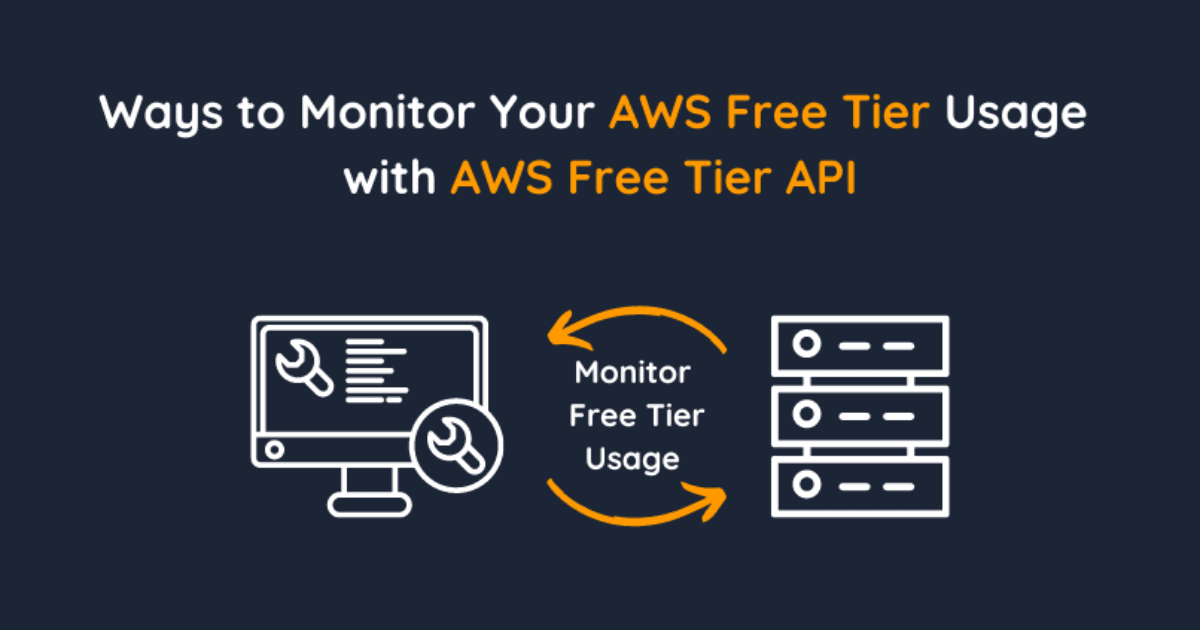
Ways to Monitor AWS Free Tier Usage with AWS Free Tier API
In this article, we will explore numerous options to monitor AWS Free Tier usage with the newly released AWS Free Tier API
Published Jan 26, 2024
Last Modified Jan 28, 2024
AWS Free Tier program provides you with the ability to explore and try out AWS services free of charge up to specified limits for each service.
The AWS Free Tier includes three different types of offerings:
- Always free offers allow customers to use a service for free up to specified limits as long as they are AWS customers.
- 12-month free offers allow customers to use a service for free up to specified limits for one year from the date the account was activated.
- Short-term trials are free to use for a specified period or up to a one-time limit, depending on the service.
There are a few ways you can keep track of your AWS Free Tier usage:
- The usage alerts in the Billing preferences of the AWS Billing and Cost Management console can send you emails when you exceed 85 percent of the Free Tier limit for each service.
- You can create a zero-spend or a monthly-cost budget in the Budgets section of the Billing and Cost Management console.
- The Free Tier page in the Billing and Cost Management console tells you the service, the type of offer, the current usage, and the forecasted usage for each offer in the current billing period.
Until recently, AWS released AWS Free Tier API that provides you the ability to check your usage of the AWS Free Tier programmatically. You can use the API directly with the AWS Command Line Interface (AWS CLI) or integrate it into an application with the AWS SDKs.
In this article, we will explore several ways to use AWS Free Tier API to monitor our AWS Free Tier Usage.
- To check for free tier usage we can call
GetFreeTierUsage
API by typing the following command
- It will then show all the free tier usage information that applies to your account like this.
- To get usage of a specific service, you can type like this
- It will then return usage information of AWS Lambda service
- To check for an actual usage amount that is greater than the limit, you can type like this.
- It will return all the usage information if which actual usage amount is greater than the limit.
- To return output in a specific response format, you can type like this
- It will return a response that returns the response format according to the format we provided our query.
In this use case, we will create a usage report notification that will notify our Lambda usage daily every day at 11:00 AM UTC or 06:00 PM GMT+7.
- To send Daily Email Usage Report Notifications we will utilize several AWS Services like Amazon SNS, Amazon Lambda, Amazon EventBridge Scheduler, and Amazon SNS.
- We will build our infrastructure using AWS CDK.
- First, create our project folder.
- Start initializing our CDK Project.
- create
index.mjs
insidesrc
folder
- Start typing the following code into
src/index.mjs
- Go to
lib/cdk-scheduled-reporting-stack.js
- Type the following code
- Then start deploying our stack with your own
email address
- CDK will then output something like this, take note of the function name.
- Make sure you confirm your SNS Topic subscriptions first from your email account.

- You can test your lambda function
CdkScheduledResportingSta-UsageReportFunction40879-IjQDqOovyRy4
like this
- After running the above command, you will receive your sales notification in your email like this.
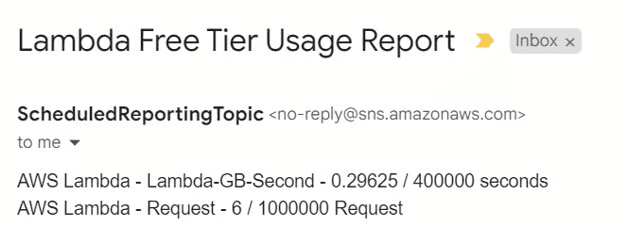
- By receiving the above email, that means our service is deployed and working perfectly. You will receive a notification similar to the above example every day at 11:00 AM UTC or 06:00 PM GMT+7.
In this use case we will utilize Free Tier API to build our own Dashboard that integrate into our own application.
- To solve this use case, we will utilize AWS Lambda with Function URL enabled.
- We will use AWS CDK to build our infrastructure.
- First, create our project folder name
free-tier-backend-api
.
- Then initialize our CDK project using the following command.
- Then create our lambda function handler inside
src/index.mjs
with the following code.
- Then go to `lib/freetier-backend-api-stack.js to start creating our stack by typing the following code.
- After that, we can start deploying our infrastructure by typing this command into our terminal.
- After our deployment is completed, CDK will output our function URL like this
https://vzthe2pe7kloemnpoyxwrdej7i0hmwps.lambda-url.ap-southeast-1.on.aws/
(take note of this).
- You can try testing our function URL whether it can return our data like this.
- Then we can start integrating it into our own web by typing this code into our HTML file, for example like this.
- The html file will output something like this.
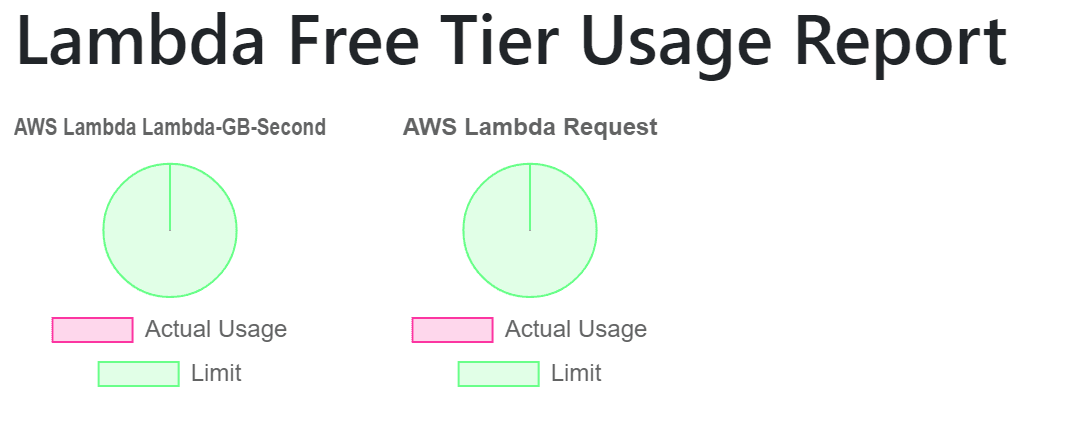
- If the output is correct, that means we successfully integrated Free Tier Usage API into our own dashboard.
will be updated next...
Using the `GetFreeTierUsage` API provides numerous options for monitoring Free Tier usage and enables prompt action. This functionality ensures efficient utilization of the AWS Free Tier offer, preventing any additional costs.