
Add Generative AI to a JavaScript Web App
Learn how to integrate genAI with minimal code changes using JS, Cognito credentials to invoke the Amazon Bedrock API in a React single page app.
How Does This Application Work?
An instance of a Large Language Model
First: Chat With Amazon Bedrock
Second: Knowledge Bases for Amazon Bedrock
Let's Deploy React Generative AI Application With Amazon Bedrock and AWS Javascript SDK
Step 1 - Enable AWS Amplify Hosting:
Step 2 - Access to the App URL:
Let's Try React Generative AI Application With Amazon Bedrock Javascript SDK
šØšØšØšØšØ This blog is outdated, check the new version Add Generative AI to a JavaScript Web App 2.0 šØšØšØšØšØšØ
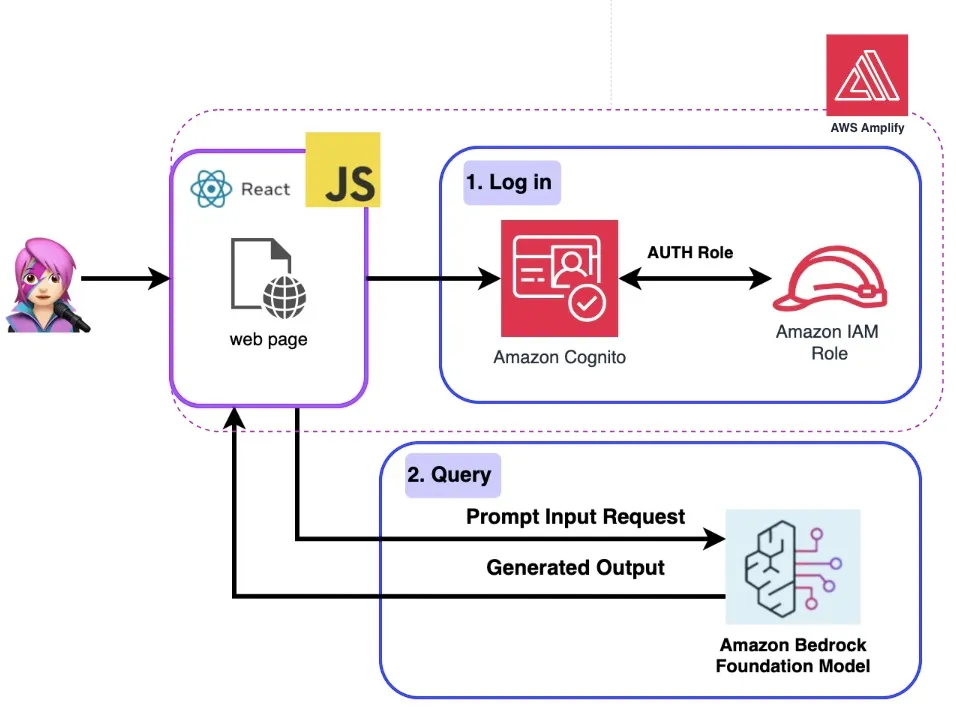
- Chat with Amazon Bedrock
- Knowledge Bases for Amazon Bedrock
Ā
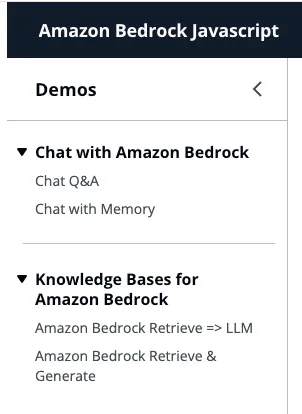
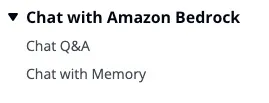
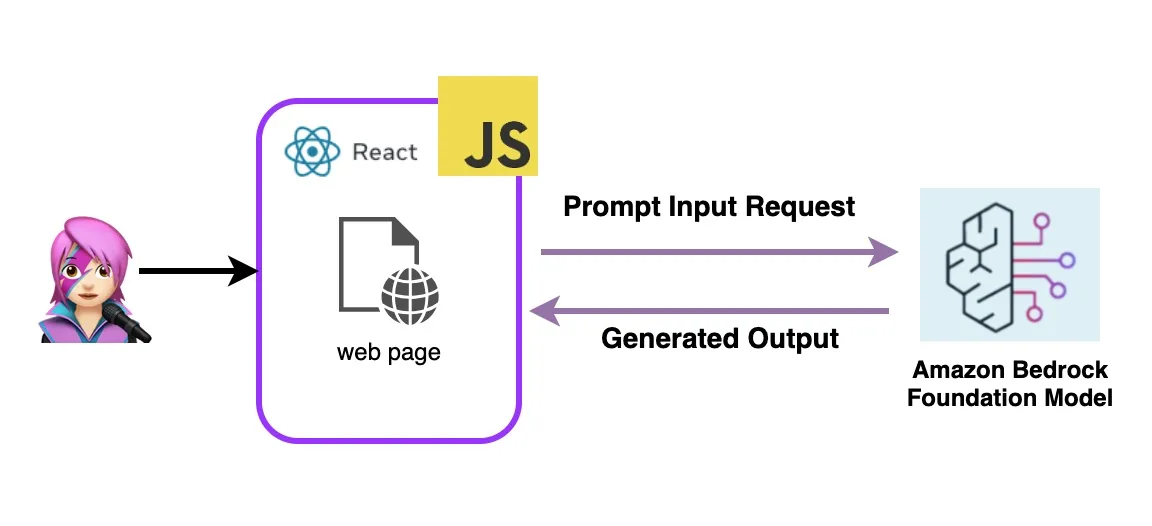
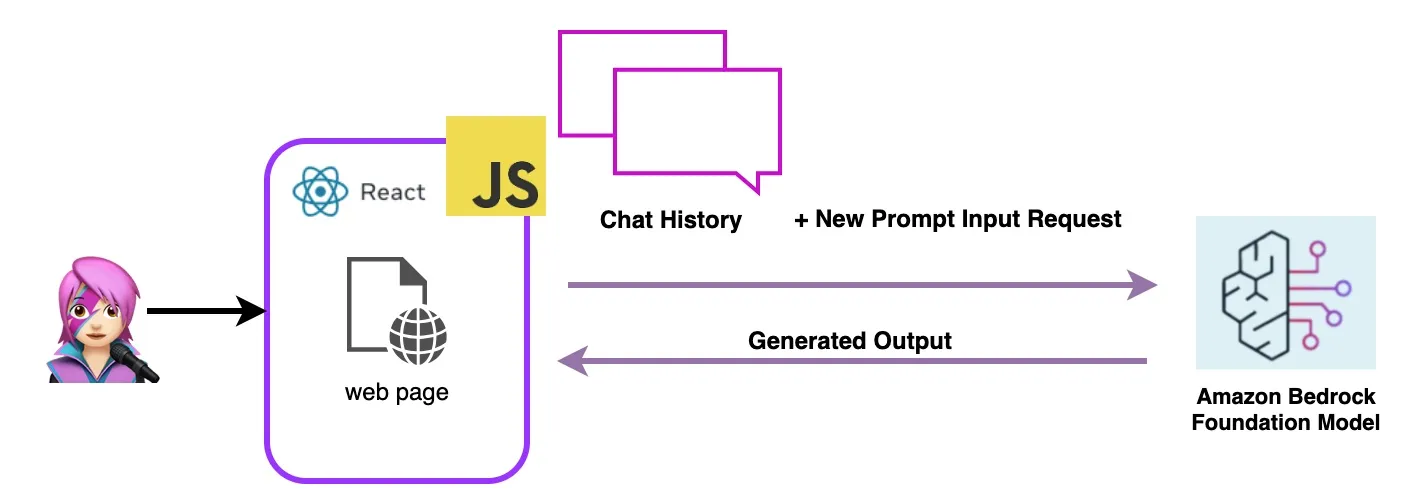
chat_history
key in the memory to get past dialogs, hence you use that key as memoryKey in BufferMemory.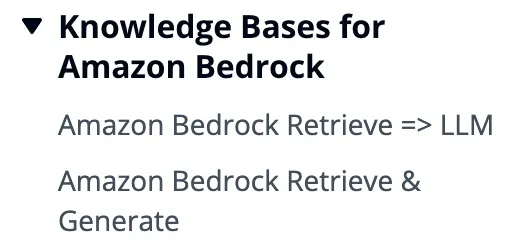
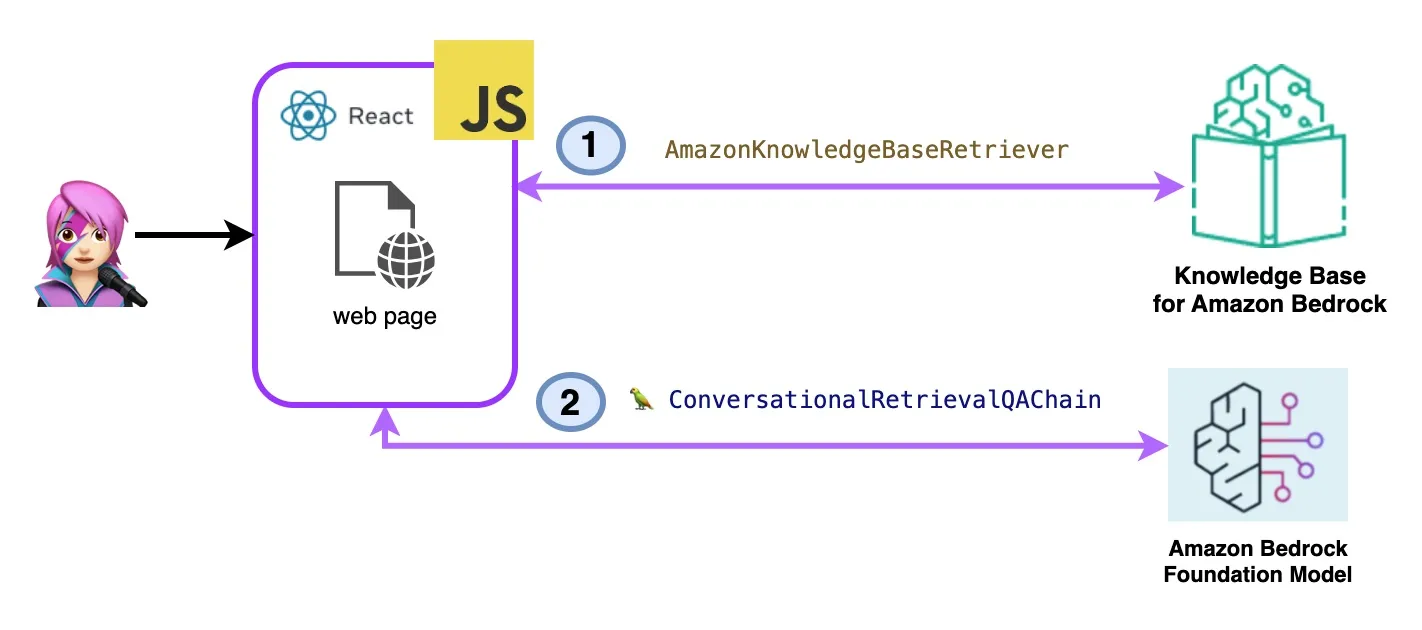
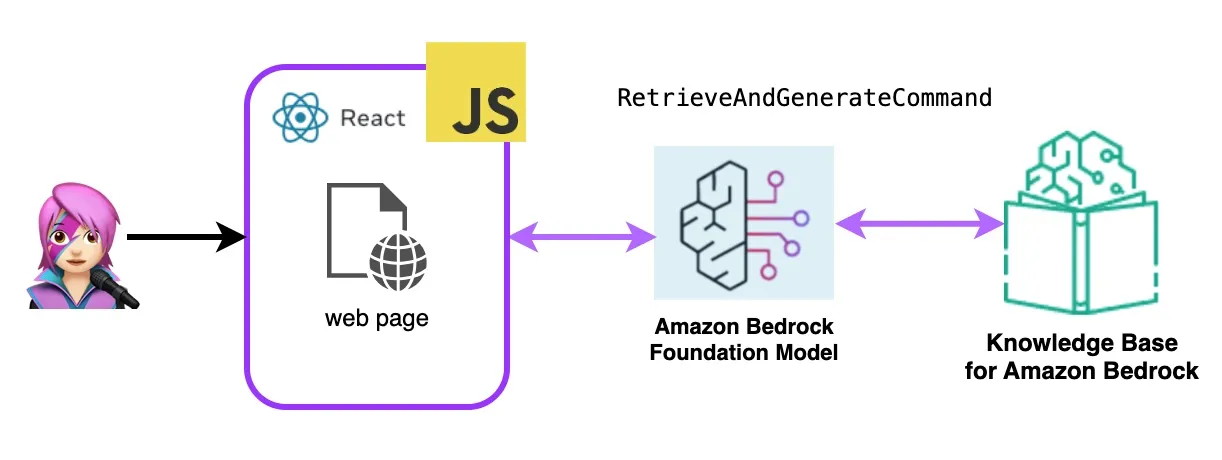
- first fork this repo:
- Create a New branch:
dev-branch
. - Then follow the steps in Getting started with existing code guide.
- In Step 1 Add repository branch, select main branch and Connecting a monorepo? Pick a folder and enter
reactjs-gen-ai-apps
as a root directory.Add repository branch - For the next Step, Build settings, select
building-a-gen-ai-gen-ai-personal-assistant-reactjs-apps(this app)
as App name, in Enviroment select Create a new envitoment and writedev.
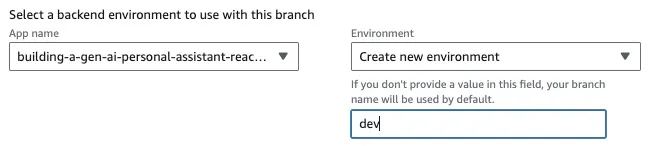
- If there is no existing role, create a new one to service Amplify.
- Deploy your app.
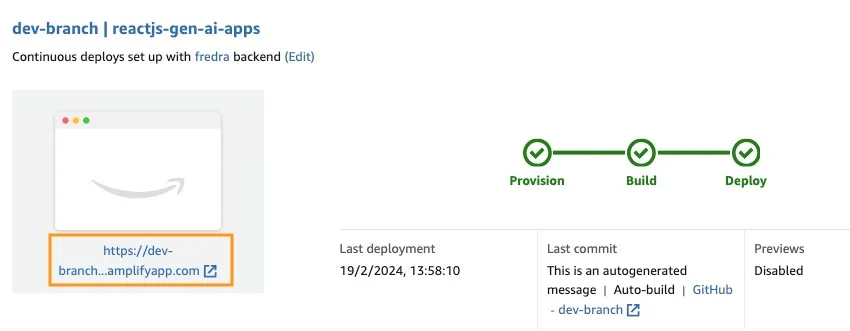
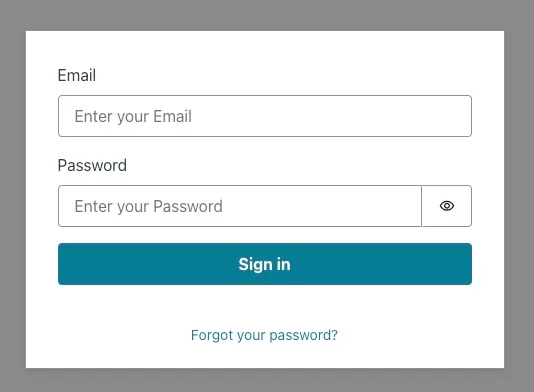
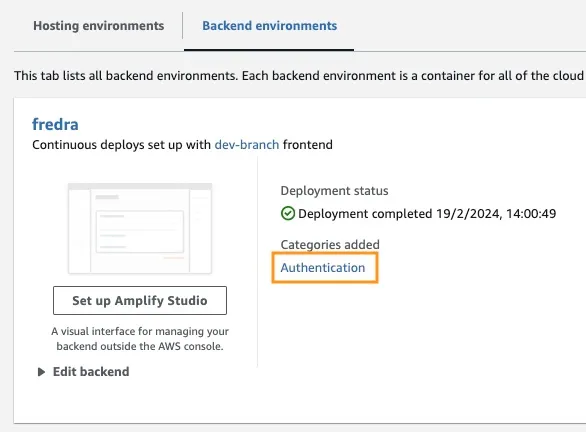
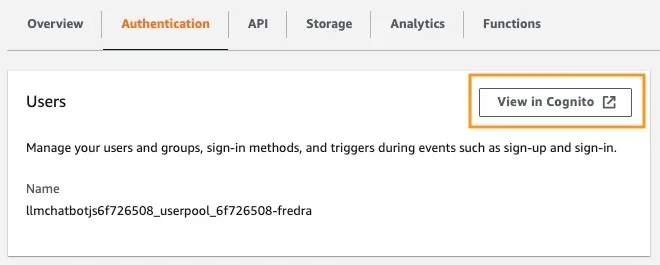
hideSignUp: false
in App.jsx, but this can introduce a security flaw by giving anyone access to it.Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.