Introduction to Threat Detection and Management on AWS
Secure your cloud infrastructure by mastering threat detection & management on AWS. This beginner's guide introduces key AWS services, security concepts & a hands-on use case. Start building a robust security posture today.
Brandon Carroll
Amazon Employee
Published Apr 11, 2024
In today's cloud-centric world, securing your infrastructure is paramount. As organizations embrace the cloud, understanding threat detection and management becomes crucial. This article will provide an overview of why threat detection matters and introduce key AWS services and components involved in this process. If you're just learning how to protect your cloud infrastructure, having an understanding of Threat Detection and Management on AWS is a must. If the topic is all together new to you, have a look at this post where I lay out the basics. When it comes to AWS, there is a wide range of services and tools to help you detect and manage threats effectively. In this series of articles I will be introducing you to why threat detection is important, some of the key services and features you'll be working with on AWS, and a use case with working examples. Let's dive in.
Cyber threats can have devastating consequences for businesses, ranging from data breaches and financial losses to damaging reputation and operational disruptions. By implementing effective threat detection and management strategies, you can proactively identify and mitigate potential risks, ensuring the security and integrity of their cloud environments. Early detection and rapid response to threats are key to minimizing the impact of cyber attacks and protecting sensitive data.
Before we get into the details, let's familiarize ourselves with some key services and components on the AWS that play a critical role in threat detection and management. Some of these services you should probably already be familiar with, but here is the list with a simple explaination.
- Virtual Private Cloud (VPC): A logically isolated section of the AWS Cloud where you can launch AWS resources in a virtual network that you define.
- Network ACLs (Network Access Control Lists): An optional layer of security for your VPC that acts as a stateless firewall for controlling inbound and outbound traffic at the subnet level.
- Security Groups: A virtual firewall that controls inbound and outbound traffic for your EC2 instances.
- AWS WAF (Web Application Firewall): A web application firewall that helps protect your web applications from common web exploits and bots.
- AWS Shield: A managed Distributed Denial of Service (DDoS) protection service that safeguards applications against DDoS attacks.
- Amazon GuardDuty: A threat detection service that continuously monitors for malicious activity and unauthorized behavior to protect your AWS accounts and workloads.
- Amazon Inspector: An automated security assessment service that helps improve the security and compliance of applications deployed on AWS.
- AWS Config: A service that enables you to assess, audit, and evaluate the configurations of your AWS resources.
- Amazon CloudWatch: A monitoring and observability service that provides data and actionable insights for AWS resources.
You're not necessarily going to see all of these discussed in this series of articles, but I want a baseline for you to work from and these are the most common services (in my opinion). With that, let's make sure we have the same basic understanding of some security terms.
As we get more into threat detection and management, I'll assume you understand some key security terms. The terms are as follows:
- Threat: A potential cause of an unwanted incident that may result in harm to a system or organization.
- Vulnerability: A weakness or flaw in a system that can be exploited by a threat actor to gain unauthorized access or cause harm.
- Risk: The potential for a threat to exploit a vulnerability and cause harm to an asset or organization.
- Incident: An occurrence that violates an explicitly or implicitly defined security policy or security practice.
- Intrusion Detection System (IDS): A system that monitors network traffic and system activities for malicious behavior or policy violations.
- Intrusion Prevention System (IPS): A system that not only detects potential threats but also takes action to prevent or mitigate the detected events.
- Firewall: A network security system that monitors and controls incoming and outgoing network traffic based on predetermined security rules.
- Denial of Service (DoS) Attack: An attack that aims to make a system or network resource unavailable to its intended users by overwhelming it with traffic or requests.
To give you a sense of Threat Detection and Management on AWS, let's consider a practical use case where we need to detect and manage threats targeting a web application hosted on AWS. We'll leverage various AWS services and security best practices to achieve this goal. I'm going to use python to deploy these features because:
- I like Python and I can always use the practice.
- You're likely going to see and use python in cybersecurity work, AWS work, and so on.
The following use case will help you understand how to implement threat detection and management on AWS by setting up various security measures and monitoring tools. By following this example, you will accomplish the following:
- Secure Network Configuration: You will create a secure Virtual Private Cloud (VPC) environment with appropriate network access controls, including subnets, network ACLs, and security groups. This lays the foundation for a secure network infrastructure.
- Web Application Protection: You will deploy AWS Web Application Firewall (WAF) and AWS Shield to protect our web application from common web exploits like SQL injection, cross-site scripting (XSS) attacks, and distributed denial-of-service (DDoS) attacks.
- Threat Detection and Vulnerability Assessment: You will enable Amazon GuardDuty to monitor our AWS environment for potential threats and malicious activities. Additionally, we will run Amazon Inspector assessments to identify potential vulnerabilities or deviations from best practices in our web application.
- Monitoring and Response: You will set up Amazon CloudWatch alarms to receive notifications when Amazon GuardDuty detects potential threats. Furthermore, we will configure AWS Config to monitor for changes in security group configurations, ensuring compliance with our security policies.
By the end of this series, you will have implemented a comprehensive threat detection and management solution on AWS. This includes securing the network infrastructure, protecting the web application from common attacks, continuously monitoring for threats and vulnerabilities, and configuring automated responses and notifications for detected threats. This hands-on approach will provide a practical understanding of how to leverage AWS services to enhance the security posture of our cloud environment. In this article we will build the base architecture. To do this, I've provided a Python script. If Python is new to you, I suggest this Coursera course to get you started. I think it's important to start introducing you to Python early since you're likely to see it often when working in Security, the Cloud, and now Generative AI.
To begin with we are going to create a base architecture to implement threat detection services on. There are many ways you can go about doing this, but for this base configuration I am going to use Python. For base configurations I often use Python, Terraform, or CloudFormation so that I can easily repeat the builds with letter effort. It's also nice to have a good starting point so we can focus on the security features rather than building an architecture every time we want to test something. So, here's an example of the Python code using the AWS SDK for Python (Boto3) to create the following resources:
- a VPC
- 2 public and 2 private subnets
- Corresponding route tables
- an Internet Gateway for the public subnets
- an Application Load Balancer
- two EC2 instance with Apache running on them
- A Certificate to use with the ALB
- Network ACLs
- Security groups
- EC2 instance connect endpoints to access the shell of the instances that are in private subnets
I've added comments in the code below to give you an idea of what each part does, but I will not be explaining it in detail in this post.
To delete the resources created by the above script you will need to delete the load balancer, target group, EC2 instances, NAT Gateway, EC2 instance connect endpoint, VPC, and possibly the certificate if you generated one.
To deploy this architecture you can run it locally. The only element missing here is the credentials to the AWS account. See the AWS Boto3 documentation for an example of how to handle your credentials. Once you've deployed this part of the code you will have architecture that looks like what is shown in figure 1.

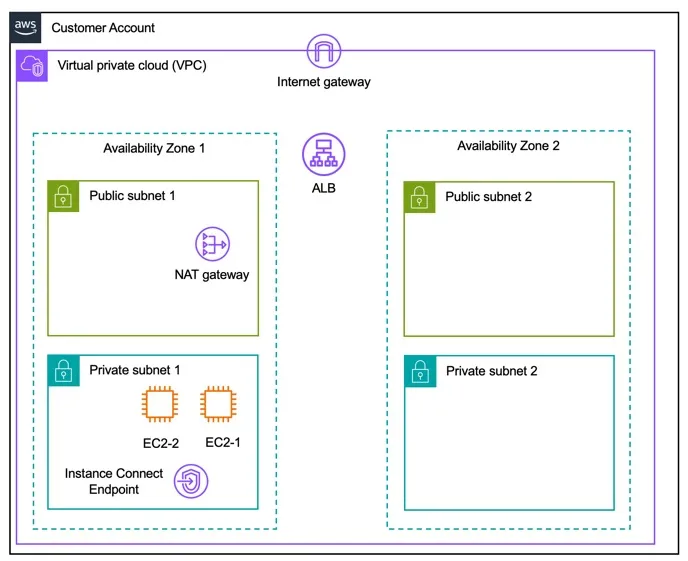
As you can see, we've built the baseline for implementing our threat detection capabilities. You can test the functionality of the environment by browsing to the URL of the load balancer. Currently the load balancer allows HTTPS traffic to the two EC2 instances in our private subnet so you may need to trust the certificate. The EC2 instances can be access via the EC2 instance connect endpoint and they have outbound connectivity through the NAT Gateway in the public subnet. This is our base architecture.
Now with our base architecture we can move to the next article in this series, Securing Your Web Application with AWS WAF and AWS Shield.
Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.