
Empowering AWS Students: AWS Insights and Guidance via Amazon Titan Text Premier with Amazon Bedrock
Dive into the world of cloud computing like never before with "The Legend of AWS Warrior"! Witness student progress in real-time, track learning journey, and receive personalized feedback, all while navigating through a fun and interactive gaming environment. Enhanced with the power of Amazon Bedrock and LangChain, this platform not only makes learning AWS services engaging but also highly effective. Step into this immersive learning experience and transform your understanding of cloud computing.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
prompt_contexts = {}
for index, row in pivot_table.iterrows():
completion_time_row_dict = row.to_dict()
completion_time = '\n'.join([f"{k}: {v if not pd.isna(v) else 'Unable to finish'}" for k, v in completion_time_row_dict.items()])
task_ranking_row = pivot_table_rank.loc[index]
task_ranking_row_dict = task_ranking_row.to_dict()
task_ranking = '\n'.join([f"{k}: {int(v) if not np.isnan(v) else 'Unable to finish'}" for k, v in task_ranking_row_dict.items()])
try:
df_columns_index_time_row = df_columns_index_time.loc[index]
df_columns_index_time_row_dict = df_columns_index_time_row.to_dict()
time_diff = '\n'.join([f"{k}: {v if not pd.isna(v) else ''}" for k, v in df_columns_index_time_row_dict.items()])
except KeyError:
time_diff = 'Special case, he is the last one to finish the task, so there is no time difference for the next student.'
prompt_contexts[index] = f"""
The time completed for each AWS task:
{completion_time}
The time ranking for each AWS task in his class:
{task_ranking}
The time difference between the student and the next student completed the task in his class:
{time_diff}
1
2
3
4
5
6
7
8
9
10
11
12
The time completed for each AWS task:
T01_CredentialTest.Test01_ValidCredential: 2024-06-04 10:12:09
...
T09_ElasticLoadBalancingTest.Test10_ElasticLoadBalancerDummyRule: 2024-07-02 23:33:16
The time ranking for each AWS task in his class:
T01_CredentialTest.Test01_ValidCredential: 80
...
T09_ElasticLoadBalancingTest.Test10_ElasticLoadBalancerDummyRule: 58
The time difference between the student and the next student completed the task in his class:
T01_CredentialTest.Test01_ValidCredential: 15
...
T09_ElasticLoadBalancingTest.Test09_ElasticLoadBalancerListenerDefaultRule: 0
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
import numpy as np
# Create a dictionary to store the results
valid_values_dict = {}
# Iterate over each column in the pivot table
for col in pivot_table.columns:
# Count the number of valid values in the column
num_valid_values = np.count_nonzero(~np.isnan(pivot_table[col].values))
# Store the result in the dictionary
valid_values_dict[col] = num_valid_values
# Print the dictionary
completed_no_dict_str = '\n'.join([f"{k}: {v}" for k, v in valid_values_dict.items()])
1
2
3
T01_CredentialTest.Test01_ValidCredential: 87
....
T09_ElasticLoadBalancingTest.Test10_ElasticLoadBalancerDummyRule: 63
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
import datetime
from langchain_core.prompts import PromptTemplate
from langchain_core.output_parsers import StrOutputParser
from langchain_aws import BedrockLLM
model = BedrockLLM(
credentials_profile_name="default",
model_id="amazon.titan-text-premier-v1:0",
region_name="us-east-1",
model_kwargs={"maxTokenCount": 1024, "temperature": 0.5, "topP": 0.95}
)
prompt = PromptTemplate.from_template(
"""
You are a teacher who wants to provide feedback to a student. The student has completed the following AWS tasks:
Scenarios:
You are going to build an application on AWS based on serverless architecture requirements provided, which contains an Amazon SQS queue that is subscribed to an Amazon SNS topic. You have to make sure the system has High Availability, disaster recovery and being Secure for remote access.
Some details / hints as to the order you should do this:
Create a new VPC with CIDR (10.0.0.0/16)
Create 4 Subnets across 2 Availability Zones (AZ), each AZ with 1 public subnet and 1 isolated subnet
Create correct Route Tables & Security Groups for all AWS services included in project
Create VPC Endpoints and setup Security Group for the Webserver lambda function i.e. S3, DynamoDB, Secret Manager and SQS.
Create a SQS queue “To_Be_Processed_Queue” with 300 seconds VisibilityTimeout
Create a SQS queue “Error_Queue”
Create a SNS topic “ErrorTopic” with SQS “Error_Queue” subscription
Create Application Load Balancer (ALB) across 2 AZs
pointing to WebServer Lambda functions
Create a S3 bucket
Deploy Lambda function “WebLambda” for Web Server
Set IAM role permission to access Aurora Serverless Database, S3
Runtime: python3.8
Deploy Lambda function “WebInitDatabaseLambda” for Database Initialization.
Set IAM role permission to access Aurora Serverless Database, S3, DynamoDB
Configure CloudWatch alarms to trigger SNS ErrorTopic
Create a DynamoDB Table.
Student Performance:
+++++++++++++++++++++++++++++++++++++++++++++++++++
{context}
+++++++++++++++++++++++++++++++++++++++++++++++++++
For Example,
"T01_CredentialTest.Test01_ValidCredential", it means "Credential Test for Valid Credential".
"T02_VpcTest.Test01_VpcExist", it means "VPC Test for VPC Exist".
This assignment start at {start} and deadline is {end}.
Today is {now}
For ranking, the class has {no_of_students} students.
The task and total number of students who completed the task are as follows:
{completed_no_dict_str}
Ranking is an important indicator for the student to know his performance.
Higher the value of Time difference implies the student should complete the task at his own alone and this is good!
Based on the completion time and ranking, provide feedback to the student on his performance.
Feedback should be constructive and encouraging.
Don't just list out the completed or failed tasks.
Analyze the completed tasks and failed tasks to provide suggestions in AWS domain to improve his AWS skills according to the tasks he has completed and failed.
Don't say anything else except the feedback in a paragraph.
Response:"""
)
output_parser = StrOutputParser()
chain = prompt | model | output_parser
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
from time import sleep
import pandas as pd
from tqdm import tqdm # Import tqdm
response_dict = {}
for item in tqdm(prompt_contexts.items()):
response = chain.invoke( {
"context": item[1],
"start": "8 May 2024",
"end": "5 July 2024",
"now": datetime.datetime.now().strftime("%d %B %Y"),
"no_of_students": len(pivot_table),
"completed_no_dict_str": completed_no_dict_str
})
response_dict[item[0]] = response
sleep(5)
Dear student, I am very pleased to see your dedication and hard work in completing the AWS tasks. Your ability to create a new VPC with CIDR (10.0.0.0/16) and 4 subnets across 2 availability zones, each with 1 public subnet and 1 isolated subnet, demonstrates your strong understanding of AWS networking. Additionally, your proficiency in creating VPC endpoints and setting up security groups for the WebServer lambda function is impressive. Your attention to detail in creating SQS queues with specific settings, such as the "To_Be_Processed_Queue" with a 300-second visibility timeout, showcases your ability to apply AWS best practices. However, I encourage you to focus on improving your skills in creating security group rules for the WebLambda and Database lambda functions. This will enhance the security and accessibility of your AWS resources. Furthermore, I suggest working on creating CloudWatch alarms to trigger SNS topics, which will enable you to monitor and respond to system errors more effectively. Keep up the excellent work, and I look forward to seeing your continued growth in the AWS domain! |
---|
Dear Student, I appreciate your efforts in completing the AWS tasks, especially in the areas of VPC creation, security group configuration, and SQS/SNS setup. Your timely completion of these tasks is commendable. However, I encourage you to focus on improving your performance in tasks related to Lambda function settings, environment variables, and CloudWatch alarms. It's important to note that while you completed these tasks, you ranked lower compared to your peers. Additionally, I recommend working on tasks related to DynamoDB table creation and Auto Scaling group settings, as you have not yet completed them. To enhance your AWS skills, consider exploring AWS documentation, participating in hands-on labs, and attending workshops. Remember, practice and continuous learning are key to mastering AWS. Keep up the good work, and I'm confident you'll make significant progress in your AWS journey. Best regards, Your Teacher. |
Dear Student, I am impressed by your performance in completing the AWS tasks. Your dedication and hard work are evident in the timely completion of tasks such as creating a VPC with CIDR (10.0.0.0/16), setting up four subnets across two availability zones, and creating correct route tables and security groups for all AWS services. Your ability to work independently and efficiently is commendable, as evidenced by the higher time difference in tasks such as creating VPC endpoints and setting up security groups for the WebServer lambda function. Your proficiency in AWS services is also evident in your completion of tasks such as creating SQS queues, SNS topics, and ALBs. Your attention to detail and ability to follow instructions are also noteworthy, as evidenced by your completion of tasks such as setting IAM role permission to access Aurora Serverless Database, S3, and DynamoDB. However, there are a few areas where you can improve your AWS skills. For instance, you may want to focus on improving your skills in creating VPCs with isolated route tables and gateway endpoints, as well as creating security groups for the WebServer lambda function. Additionally, you may want to work on improving your skills in creating SNS topics and setting up SNS subscriptions to SQS queues. Overall, your performance has been excellent, and I am confident that with continued practice and learning, you will become an expert in AWS. Keep up the good work! |
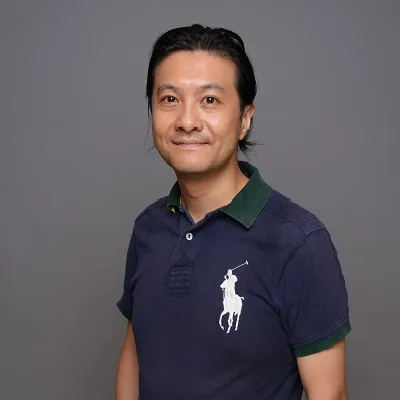