Learn Python and build a game with an AI companion
A generative AI–powered assistant can help you learn programming while building small projects such as a text game.
game.py
file. In my setup, I have my IDE open and the Amazon Q chat open on one side of the editor.Tell me 5 simple text games that I can build in Python.
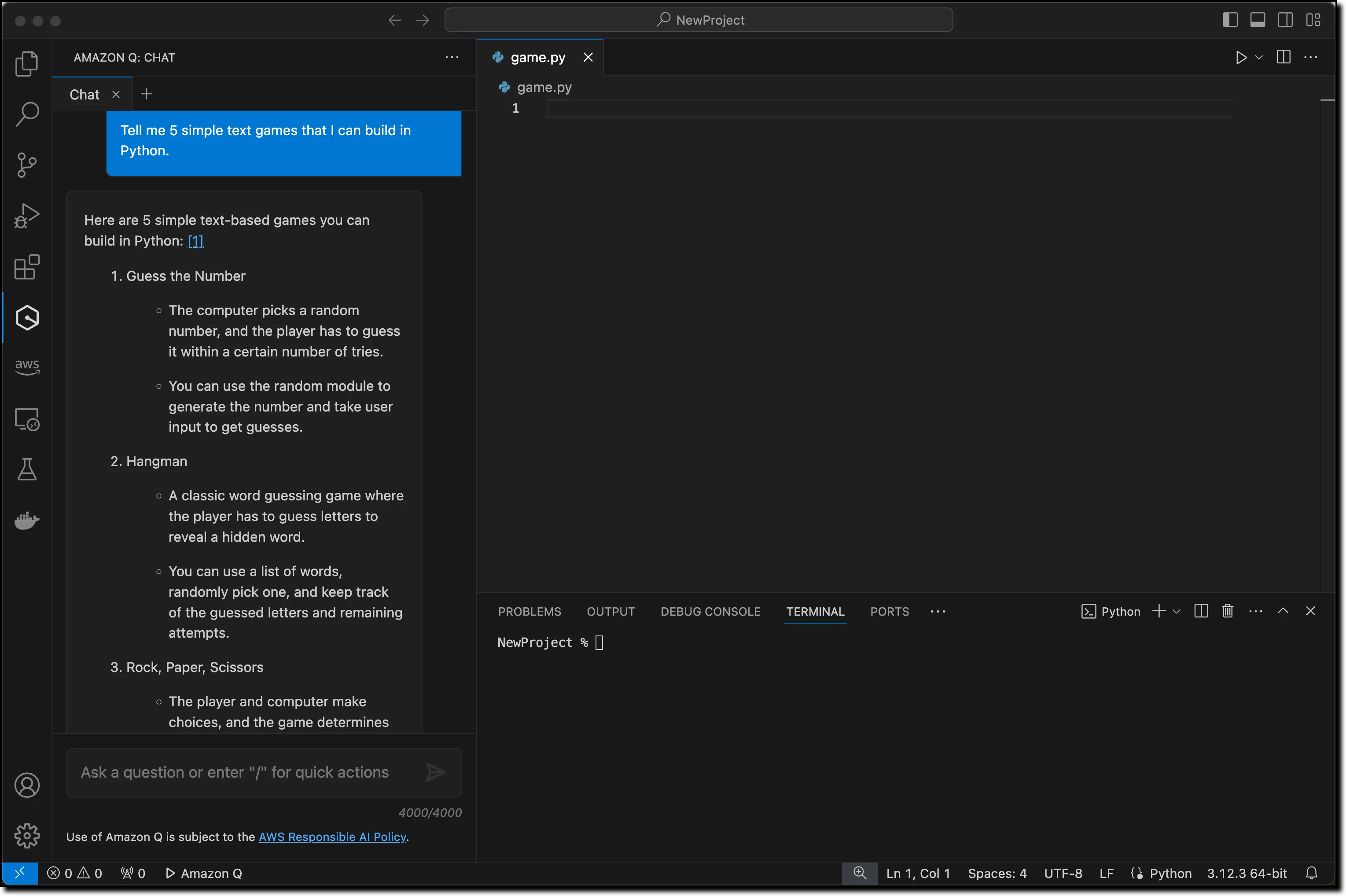
Give me the starting Python code for a Rock, Paper, Scissors game.
- The
get_choices()
function prompts the user to enter their choice (rock, paper, or scissors) and randomly selects the computer's choice from the same options. It returns a dictionary containing the player's and computer's choices. - The
check_win()
function takes the player's and computer's choices as arguments. It first prints out the choices made by both players. Then, it checks for a tie condition. If it's not a tie, it checks the player's choice and the computer's choice to determine the winner based on the rules of Rock, Paper, Scissors. It returns the appropriate result message. - The
choices
dictionary is created by calling theget_choices()
function. - The
result
variable stores the result message returned by thecheck_win()
function, passing in the player's and computer's choices. - Finally, the result message is printed to the console.
play_game
function handling the game loop.Create a play_game function that loops until the player enters "bye".
How can I create a constant in Python?
Refactor the code and put the three options (rock, paper, scissors) into a global list. Because that list is constant, use an UPPERCASE name such as CHOICES.
Replace recursion in the get_choices function with a loop.
while True
loop.To check who wins, update the code to use a BEATS dictionary that, for any choice, returns the option that looses against that choice. Refactor the code to compute CHOICES from BEATS. Then, rename the check_win function as "who_wins" and make it return "player", "computer", or "tie".
who_wins
function, the now returns only one word (either player
, computer
, or tie
), the play_game
function now implements the formatting and the dsiplay of text.get_choices
). The player is getting input from the terminal, while the computer uses some strategy (currently randomness) to find the right choice. Because these are completely different procedures, I ask to separate the functions:Separate get_choices in two functions, one for the player and one for the computer.
get_player_choice
and a get_computer_choice
functions. Here's the full code with the changes.get_player_choice
function can be improved. For example, "bye" is repeated twice. I select the function in the editor and choose Amazon Q and Optimize from the contextual menu. I can use the same approach to Explain, Fix, or Refactor code, or use a custom prompt with the selected code to do something different, such as asking the code to be changed in a specific way.Update the code to store of the number of player and computer wins inside the play_game function. Print the scores at every turn. At the end, print who won more.
play_game
function. Even if global variables can be ok in a small program, I prefer to avoid them because they can create problems when an application grows and becomes more complex.Compute the opposite of the BEATS dictionary and call it BEATEN_BY.
Optimize the computer strategy with these changes. In the get_computer_choice function, pass in input a list of all player previous choices. Then, get a random element from the list and return the choice that beats that one (the value of BEATEN_BY[random element]). The list is updated after the computer makes its choice. If the list is empty, return a random choice.
Optimize the computer startegy to only use the latest n entries in the player history, with n equal 10. Instead of n, call the variable MEMORY_LENGTH.
get_computer_choice
function and the MEMORY_LENGTH
constant.get_computer_choice
function can be improved. For example, slicing an array in Python works even if there are less element than what is requested. I select the function and, using the contextual menu, I ask Amazon Q to optimize the code.Run the play_game function only if this is run and not imported as a module.
Replace the BEATS, BEATEN_BY, and CHOICES data structures with a single RockPaperScissorsGame class that implementes the same functionalities. This class shoud have an embedded BEATS structure from which all other data is computed.
Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.