How to build a single-page application deployment using AWS CDK
Learn how to deploy a single-page application (or any other static assets) using AWS CDK
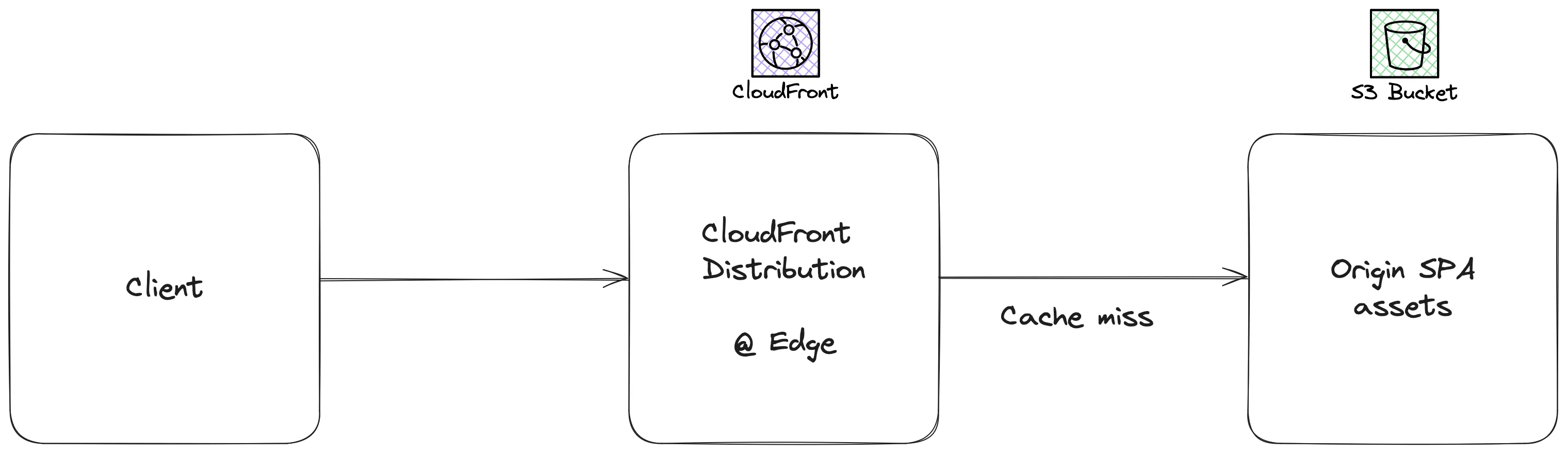
- S3 Bucket
- CloudFront Distribution
1
2
3
4
5
6
7
const s3Bucket = new cdk.aws_s3.Bucket(this, "s3-bucket-content", {
blockPublicAccess: cdk.aws_s3.BlockPublicAccess.BLOCK_ALL,
encryption: cdk.aws_s3.BucketEncryption.S3_MANAGED,
enforceSSL: true,
removalPolicy: cdk.RemovalPolicy.RETAIN,
bucketName: "cdk-spa-assets-pattern",
});
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
const originAccessIdentity = new cdk.aws_cloudfront.OriginAccessIdentity(
this,
"cloudfront-origin-access-identity"
);
s3Bucket.grantRead(originAccessIdentity);
const cloudfrontS3Origin = new cdk.aws_cloudfront_origins.S3Origin(
s3Bucket, {
originAccessIdentity: originAccessIdentity,
}
);
const distribution = new cdk.aws_cloudfront.Distribution(
this,
"cloudfront-content-distribution", {
defaultBehavior: {
origin: cloudfrontS3Origin,
},
defaultRootObject: "index.html",
priceClass: cdk.aws_cloudfront.PriceClass.PRICE_CLASS_100,
}
);
OriginAccessIdentity
construct, this is then used to grant read access to the S3 bucket before assigning it to the CloudFront distribution itself.Distribution
construct here as it’s the newer implementation (replacing the CloudFrontWebDistribution
construct). As part of that, the API has changed slightly and we need to define a CloudFront origin itself - we are using S3 as our origin and can pass our origin identity permission into it to allow the distribution to read from the bucket.index.html
- so whenever you build your SPA assets, you simply push them into the bucket and you will be good to go!- Static assets can be pushed into S3 bucket and served via the global CloudFront CDN network.
- Extremely cost-effective for working with a lot of network requests.
- Can work for SPA static websites or other general static content that might be required to power your applications.