Serverless Image Generation Application Using Generative AI on AWS
Use Amazon Bedrock to build an image generation solution in Go and deploy it using AWS CDK.
Abhishek Gupta
Amazon Employee
Published Nov 1, 2023
Last Modified May 22, 2024
Whether it's crafting personalized content or tailoring images to user preferences, the ability to generate visual assets based on a description is quite powerful. But text-to-image conversion typically involves deploying an end-to-end machine learning solution, which is quite resource intensive. What if this capability was an API call away, thereby making the process simpler and more accessible for developers?
This tutorial will walk you through how to use AWS CDK to deploy a Serverless image generation application implemented using AWS Lambda and Amazon Bedrock, which is a fully managed service that makes base models from Amazon and third-party model providers (such as Anthropic, Cohere, and more) accessible through an API. Developers can leverage leading foundation models through a single API, while maintaining the flexibility to adopt new models in the future.
The solution is deployed as a static website hosted on Amazon S3 accessible via an Amazon CloudFront domain. Users can enter the image description which will be passed on to a Lambda function (via Amazon API Gateway) which in turn will invoke Stable Diffusion model on Amazon Bedrock to generate the image.
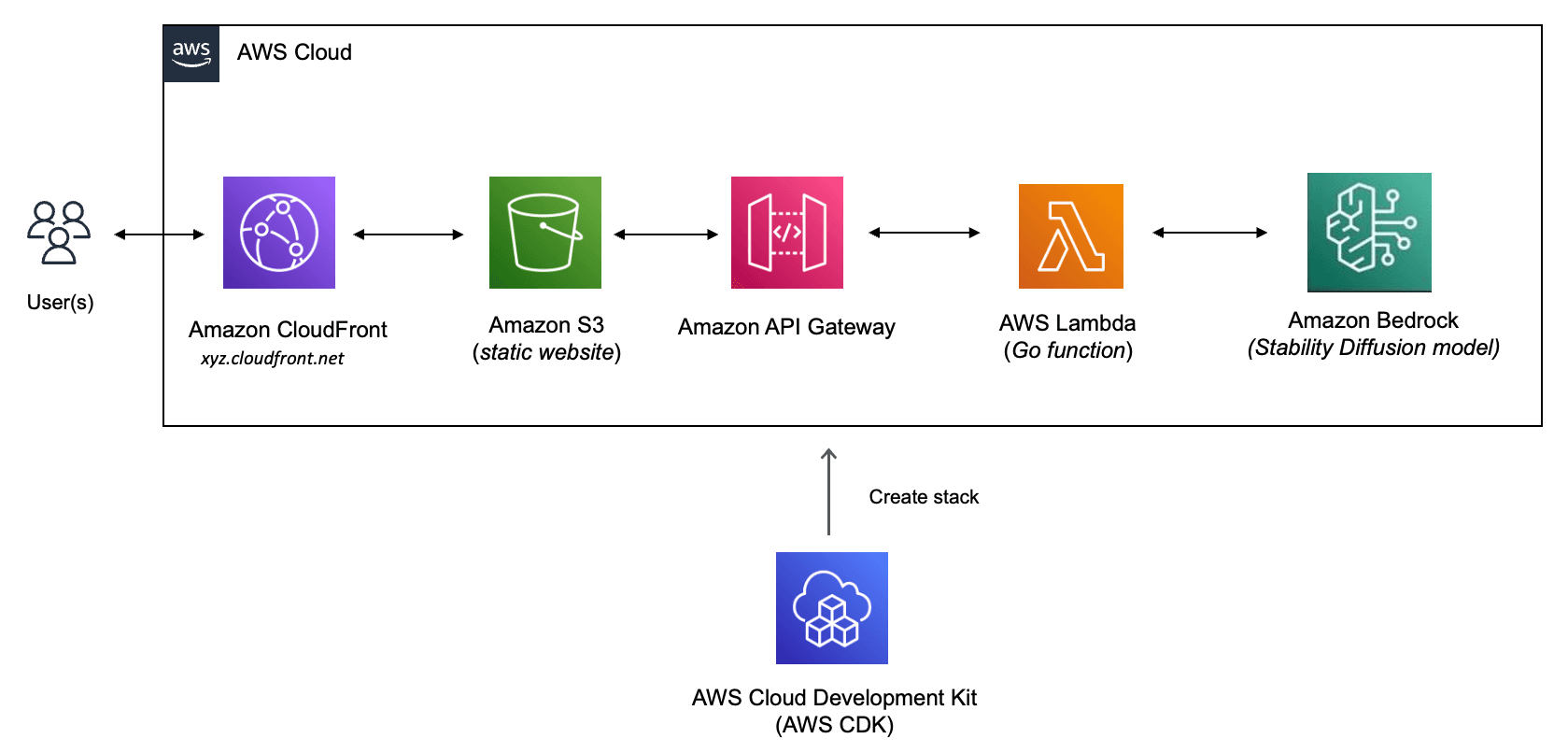
The entire solution is built using Go - this includes the Lambda function (using aws-lambda-go library) as well as the complete solution deployment using AWS CDK.
The code is available on GitHub.
About | |
---|---|
β AWS Level | 200 - Intermediate |
β± Time to complete | 30 minutes |
π° Cost to complete | Free when using the AWS Free Tier |
π» Code Sample | Code sample used in tutorial on GitHub |
π’ Feedback | Any feedback, issues, or just a π / π ? |
β° Last Updated | 2023-11-01 |
Before starting this tutorial, you will need the following:
- An AWS Account (if you don't yet have one, you can create one and set up your environment here).
- Go (v1.19 or higher).
- Git.
Clone this GitHub repository and change to the right directory:
To start the deployment, simply invoke
cdk deploy
.You will see a list of resources that will be created and will need to provide your confirmation to proceed (output shortened for brevity).
This will start creating the AWS resources required for the application.
If you want to see the AWS CloudFormation template which will be used behind the scenes, runcdk synth
and check thecdk.out
folder.
You can keep track of the progress in the terminal or navigate to AWS console:
CloudFormation > Stacks > BedrockLambdaImgeGenWebsiteStack
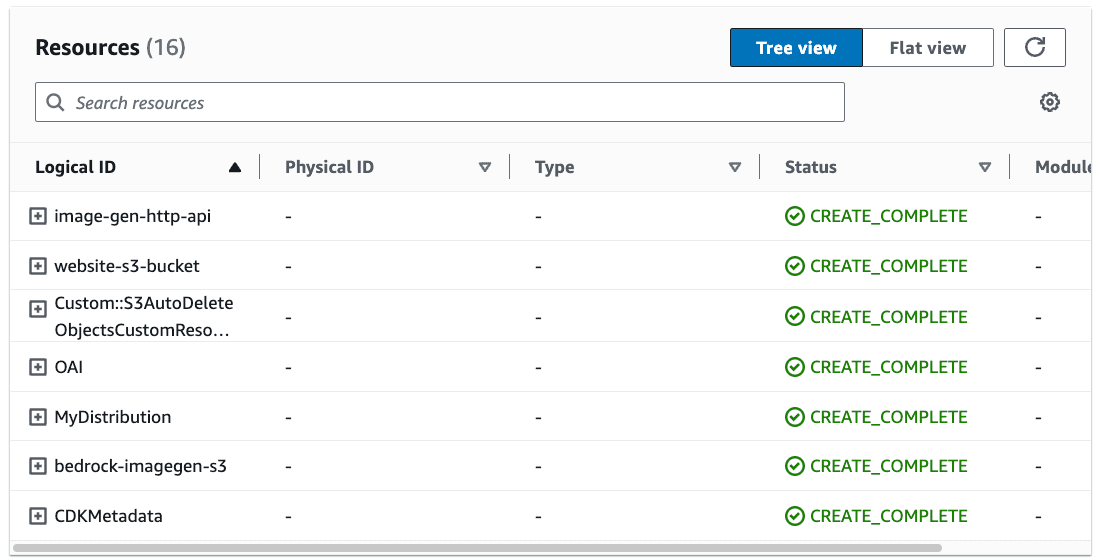
Once all the resources are created, you can try out the application. You should have:
- The image generation Lambda function and API Gateway.
- An S3 bucket to host the website HTML page.
- CloudFront distribution.
- And a few other components (like IAM roles, permissions, S3 Bucket policy etc.)
The deployment can take a bit of time since creating the CloudFront distribution is a time-consuming process. Once complete, you should get a confirmation along with the values for the S3 bucket name, API Gateway URL, and the CloudFront domain name.
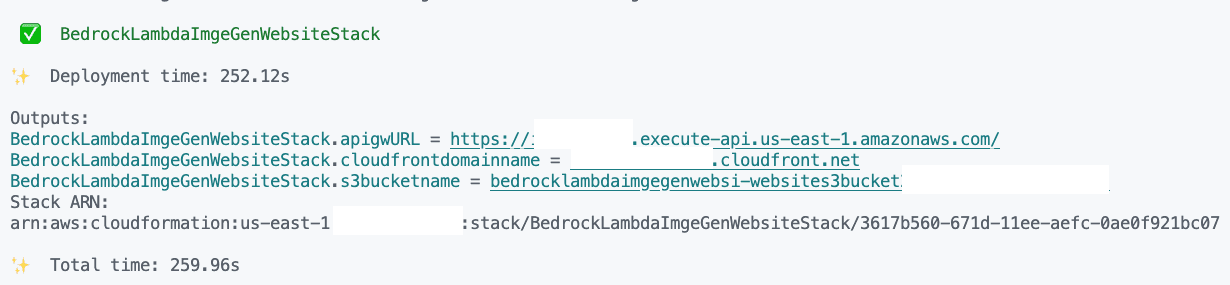
Open the
index.html
file in the GitHub repo, and locate the following text ENTER_API_GATEWAY_URL
. Replace this with the API Gateway URL that you received as the CDK deployment output above.To copy the file to S3, I used the AWS CLI:
Verify that the file was uploaded:
Now you are ready to access the website!
Enter the CloudFront domain name in your web browser to navigate to the website. You should see the website with a pre-populated description that can be used as a prompt.
Click Generate Image to start the process. After a few seconds, you should see the generated image.

The Stability Diffusion model allows us to refine the generation parameters as per our requirements.
The Stability.ai Diffusion models support the following controls:
- Prompt strength (
cfg_scale
) controls the image's fidelity to the prompt, with lower values increasing randomness. - Generation step (
steps
) determines the accuracy of the result, with more steps producing more precise images. - Seed (
seed
) sets the initial noise level, allowing for reproducible results when using the same seed and settings.
Click Show Configuration to edit these.
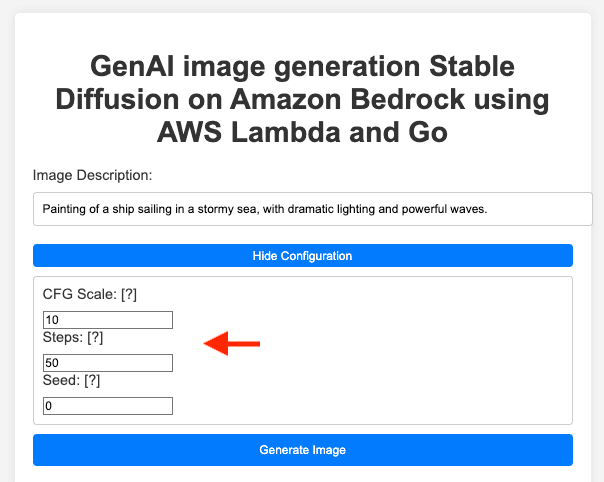
Max values forcfg_steps
andsteps
are 30 and 150 respectively.
Once you're done, to delete all the services, simply use:
You were able to set up and try the complete solution. Before we wrap up, let's quickly walk through some of important parts of the code to get a better understanding of what's going the behind the scenes.
Since we will only focus on the important bits, a lot of the code (print statements, error handling etc.) has been omitted for brevity.
You can refer to the CDK code here.
We start by creating the API Gateway and the S3 bucket.
Then we create the CloudFront Origin Access Identity and grant S3 bucket read permissions to the CloudFront Origin Access Identity principal. Then we create the CloudFront Distribution:
- Specify the S3 bucket as the origin.
- Specify the Origin Access Identity that we created before.
Then, we create the image generation Lambda function along with IAM permissions (to the function execution IAM role) to allow it to invoke Bedrock operations.
Finally, we configure Lambda function integration with API Gateway, add the HTTP routes and specify API Gateway endpoint, S3 bucket name and CloudFront domain name as CloudFormation outputs.
You can refer to the Lambda Function code here.
In the function handler, we extract the prompt from the HTTP request body, and the configuration from the query parameters. Then it's used to call the model using bedrockruntime.InvokeModel function. Note the JSON payload sent to Amazon Bedrock is represented by an instance of the
Request
struct.The output body returned from Amazon Bedrock Stability Diffusion model is a JSON payload which is converted into a
Response
struct which contains the generated image as a base64
string. This is returned as an events.APIGatewayV2HTTPResponse object along with CORS
headers.In this tutorial, you used AWS CDK to deploy a serverless image generation solution that was implemented using Amazon Bedrock and AWS Lambda and was accessed using a static website on S3 via a CloudFront domain.
To stay updated with Generative AI content, visit this Space. If you are interested in an introductory guide to using the AWS Go SDK and Amazon Bedrock Foundation Models (FMs), check out this blog post.
Happy building!
Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.