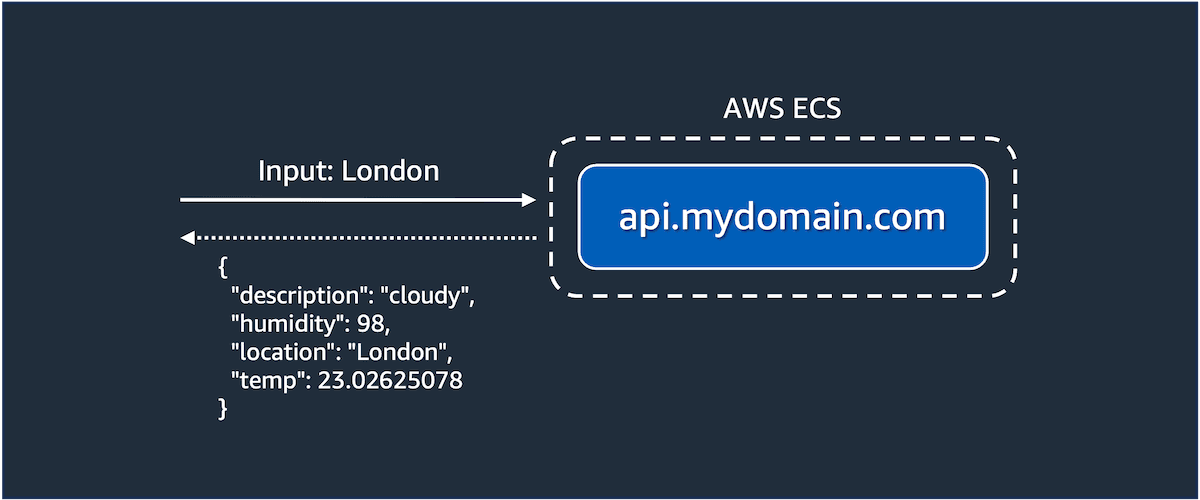
Developing Neural Searches with Custom Models
Are you excited with the OpenSearch models feature but need to use custom models? No problems. In this tutorial, you will learn how to use your own deployed model with OpenSearch.
- Models from Hugging Face or custom models deployed onto a container or virtual machine
- Models deployed on Amazon SageMaker, including via JumpStart
- ...any API that you can connect to from the OpenSearch cluster.
git clone https://github.com/build-on-aws/getting-started-with-opensearch-models
cd getting-started-with-opensearch-models/custom-ml-api
docker compose up -d
1
curl -u admin:secret -H 'Content-Type: application/json' http://localhost:8888/weather -d '{"text_inputs": "London"}'
1
2
3
4
5
6
{
"description": "cloudy",
"humidity": 98,
"location": "London",
"temp": 23.0262507888756
}
1
2
3
4
5
6
7
8
9
PUT /_cluster/settings
{
"persistent": {
"plugins.ml_commons.connector_access_control_enabled": true,
"plugins.ml_commons.trusted_connector_endpoints_regex": [
"^https://api.mydomain.com/.*$"
]
}
}

GET
or a POST
. In this case, a POST
is used as a POST
body is sent with the request:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
POST /_plugins/_ml/connectors/_create
{
"name": "weather",
"description": "weather",
"version": 1,
"protocol": "http",
"credential": {
"secretArn": "<YOUR_SECRET>",
"roleArn": "<YOUR_ROLE>"
},
"actions": [
{
"action_type": "predict",
"method": "POST",
"headers": {
"content-type": "application/json",
"Authorization": "Basic ${credential.secretArn.secretValue}"
},
"url": "https://api.mydomain.com/weather",
"request_body": "{ \"text_inputs\": \"${parameters.input}\" }",
}
]
}
model_id
of that task is retrieved using the task_id
. With that, the model can be deployed:1
2
3
4
5
6
7
8
9
10
11
12
POST /_plugins/_ml/models/_register
{
"name": "minilm-l6",
"function_name": "remote",
"model_group_id": "28yhqosBjS86SRECErTY",
"description": "test model",
"connector_id": "W3ZnuosBt2q9Vp9BKukn"
}
GET /_plugins/_ml/tasks/vd9nuosBjS86SRECXYn6
POST /_plugins/_ml/models/vt9nuosBjS86SRECXokQ/_deploy
1
2
3
4
POST /_plugins/_ml/models/vt9nuosBjS86SRECXokQ/_predict
{
"parameters": {"input": "London"}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
{
"inference_results": [
{
"output": [
{
"name": "response",
"dataAsMap": {
"description": "rainy",
"humidity": 76,
"location": null,
"temp": 22.281690890440444
}
}
],
"status_code": 200
}
]
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
{
"name": "weather",
"description": "weather",
"version": 1,
"protocol": "http",
"credential": {
"secretArn": "<YOUR_SECRET>",
"roleArn": "<YOUR_ROLE>"
},
"actions": [
{
"action_type": "predict",
"method": "POST",
"headers": {
"content-type": "application/json",
"Authorization": "Basic ${credential.secretArn.secretValue}"
},
"url": "https://api.mydomain.com/embed",
"pre_process_function": "\n StringBuilder builder = new StringBuilder();\n builder.append(\"\\\"\");\n String first = params.text_docs[0];\n builder.append(first);\n builder.append(\"\\\"\");\n def parameters = \"{\" +\"\\\"input_text\\\":\" + builder + \"}\";\n return \"{\" +\"\\\"parameters\\\":\" + parameters + \"}\";",
"post_process_function": "\n def name = \"sentence_embedding\";\n def dataType = \"FLOAT32\";\n if (params.results == null || params.results.length == 0) {\n return params.message;\n }\n def shape = [params.results.length];\n def json = \"{\" +\n \"\\\"name\\\":\\\"\" + name + \"\\\",\" +\n \"\\\"data_type\\\":\\\"\" + dataType + \"\\\",\" +\n \"\\\"shape\\\":\" + shape + \",\" +\n \"\\\"data\\\":\" + params.results +\n \"}\";\n return json;\n ",
"request_body": "{ \"text_inputs\": \"${parameters.input_text}\" }",
}
]
}
pre_process_function
and a post_process_function
is used. These allow the request and response to be transformed when working with a model, so it is in the shape expected by OpenSearch.1
2
3
4
5
6
POST /_plugins/_ml/models/-OnayIsBvAWGexYmHu8G/_predict
{
"parameters": {"input_text": "hello world"},
"return_number": true,
"target_response": ["sentence_embedding"]
}
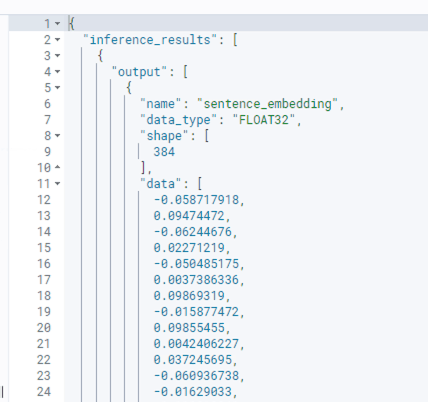
1
{ "text_inputs": "hello world" }
1
2
response_data ={ "results" : [-0.058717918, 0.09474472, -0.06244676, ... , -0.0073602716]}
return jsonify(response_data)
post_process_function
then processes the APIs response. The example below has again formatted here for readability. For a working example, see the connector definition above:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
"post_process_function": "
def name = "sentence_embedding";
def dataType = "FLOAT32";
if (params.results == null || params.results.length == 0) {
return params.message;
}
def shape = [params.results.length];
def json = "{
"name": + name + "," +
"data_type": + dataType + "," +
"shape": + shape + "," +
"data": + params.results +
"}";
return json;",
params.results
. Any attributes in the response object can be accessed via the params object. This function is used to specify which attributes in the response to map and return to OpenSearch. Pre and post functions can be used as required to transform the request and response to the desired format.1
2
3
4
name: STRING (e.g. "sentence_embedding")
data_type: STRING (e.g. "FLOAT32")
shape: INT[] (e.g. [384])
data: FLOAT32[] (e.g. [-0.321, 1.234, etc])
data_type
is a string representation of the data type used in the data attribute, for example FLOAT32 or INT32.dimension
attribute defined on the index vector property.post_process_function
so that it matches.1
2
3
4
5
6
7
8
9
10
11
12
13
14
GET /nlp_pqa_2/_search
{
"_source": [ "question" ],
"size": 30,
"query": {
"neural": {
"question_vector": {
"query_text": "What is the meaning of life?",
"model_id": "-OnayIsBvAWGexYmHu8G",
"k": 30
}
}
}
}

1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
PUT _ingest/pipeline/nlp-pipeline
{
"description": "An example neural search pipeline",
"processors" : [
{
"text_embedding": {
"model_id": "-OnayIsBvAWGexYmHu8G",
"field_map": {
"question": "question_vector"
}
}
}
]
}
PUT /nlp_pqa
{
"settings": {
"index.knn": true,
"index.knn.space_type": "cosinesimil",
"default_pipeline": "nlp-pipeline",
"analysis": {
"analyzer": {
"default": {
"type": "standard",
"stopwords": "_english_"
}
}
}
},
"mappings": {
"properties": {
"question_vector": {
"type": "knn_vector",
"dimension": 384,
"method": {
"name": "hnsw",
"space_type": "l2",
"engine": "faiss"
},
"store": true
},
"question": {
"type": "text",
"store": true
},
"answer": {
"type": "text",
"store": true
}
}
}
}
1
2
POST /nlp_pqa_2/_doc/3
{"question":"How many legs does an Elephant have?","answer":"At least 4."}

