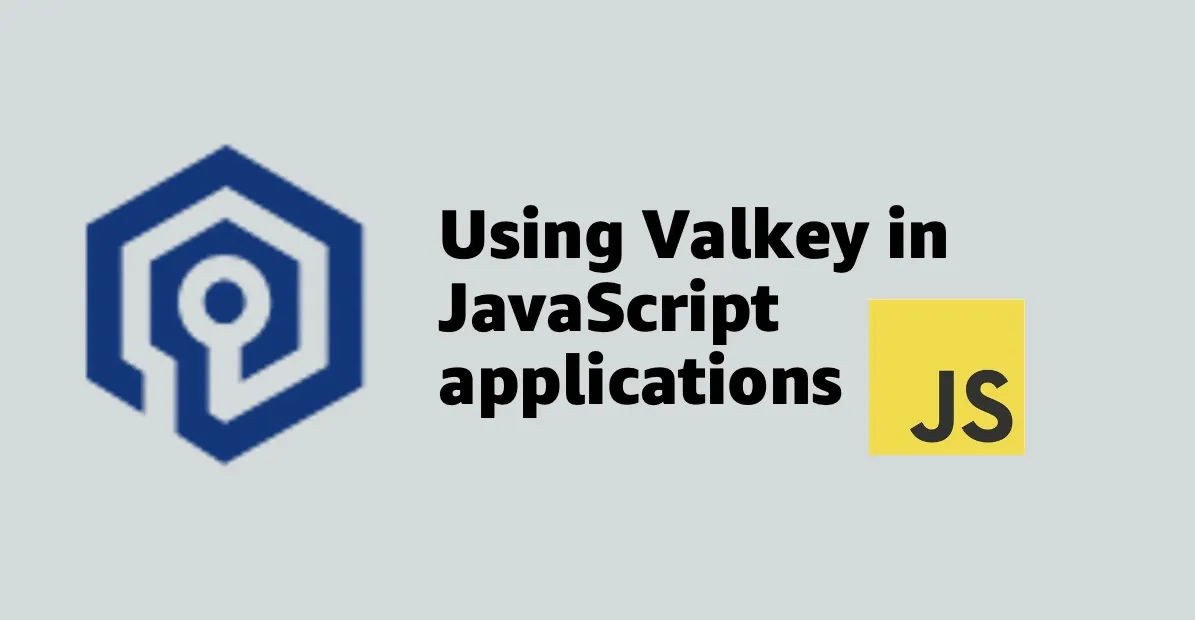
Getting started with Valkey using JavaScript
Run existing Redis apps with Valkey and learn how to use it with LangChain
Abhishek Gupta
Amazon Employee
Published Jun 17, 2024
Valkey is an open source alternative to Redis. It's a community-driven, Linux Foundation project created to keep the project available for use and distribution under the open source Berkeley Software Distribution (BSD) 3-clause license after the Redis license changes.
I think the path to Valkey was well summarised in this inaugural blog post:
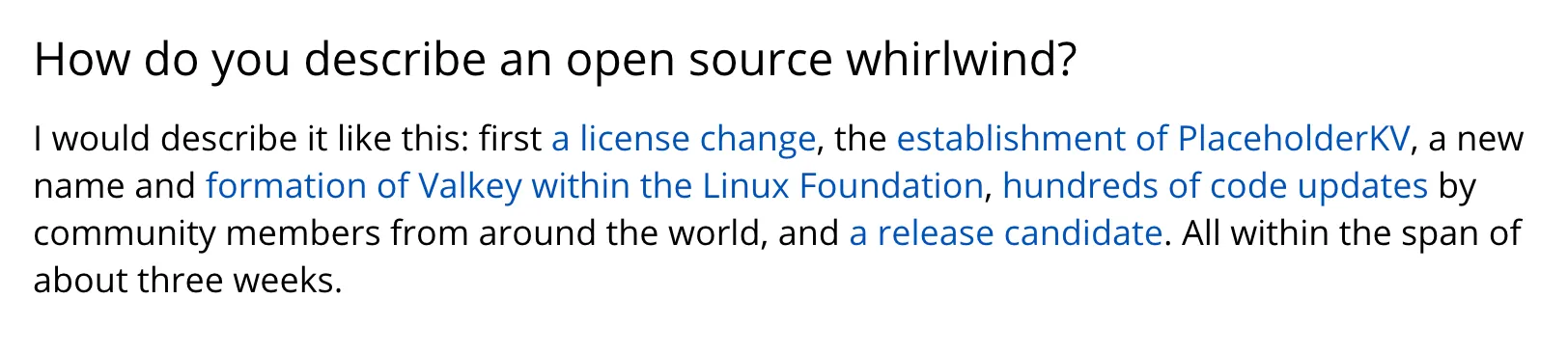
I will walk through how to use Valkey for JavaScript applications using existing clients in Redis ecosystem as well as iovalkey (a friendly fork of ioredis).
node-redis is a popular and widely used client. Here is a simple program that uses the Subscriber component of the PubSub API to subscribe to a channel.
To try this with Valkey, let's start an instance using the Valkey Docker image:
Also, head over to https://valkey.io/download to get OS specific distribution, or use Homebrew (on Mac) -
brew install valkey
. You should now be able to use the Valkey CLI (valkey-cli
).Get the code from GitHub repo:
Start the subscriber app:
Publish a message and ensure that the subscriber is able to receive it:
Nice! We were able to write a simple application with an existing Redis client and run using Valkey (instead of Redis). Sure, this is an over-simplified example, but we there were no code changes required.
ioredis is another popular client. To be doubly sure, lets try
ioredis
with Valkey as well. Lets write a publisher application:Run the publisher, and confirm that the subscriber app is able to receive it:
You should see these logs in the subscriber application:
As mentioned,
iovalkey
is a fork of ioredis
. I made the following changes to port the producer code to use iovalkey
:Commented out
import Redis from 'ioredis';
- Added
import Redis from 'iovalkey';
- Installed
iovalkey
-npm install iovalkey
Here is the updated version - yes, this was all I needed to change (at least for this simple application):
Run the new
iovalkey
based publisher, and confirm that the subscriber is able to receive it:You should see these logs in the subscriber application:
Awesome, this is going well. We are ready to sprinkle some generative AI now!
Along with Python, JavaScript/TypeScript is also being used in the generative AI ecosystem. LangChain is a popular framework for developing applications powered by large language models (LLMs). LangChain has JS/TS support in the form of LangchainJS.
One of the common use cases is to use Redis as a chat history component in generative AI apps. LangchainJS has this built-in, so let's try it out with Valkey.
To install LangchainJS:
For the LLM, I will be using Amazon Bedrock (its supported natively with LangchainJS), but feel free to use others.
For Amazon Bedrock, you will need to configure and set up Amazon Bedrock, including requesting access to the Foundation Model(s).
Here is the chat application. As you can see, it uses the
RedisChatMessageHistory
component.Run the application:
Start a conversation:

If you peek into Valkey, notice that the conversations are saved in a
List
:Don't run` keys *` in production - its just for demo purposes
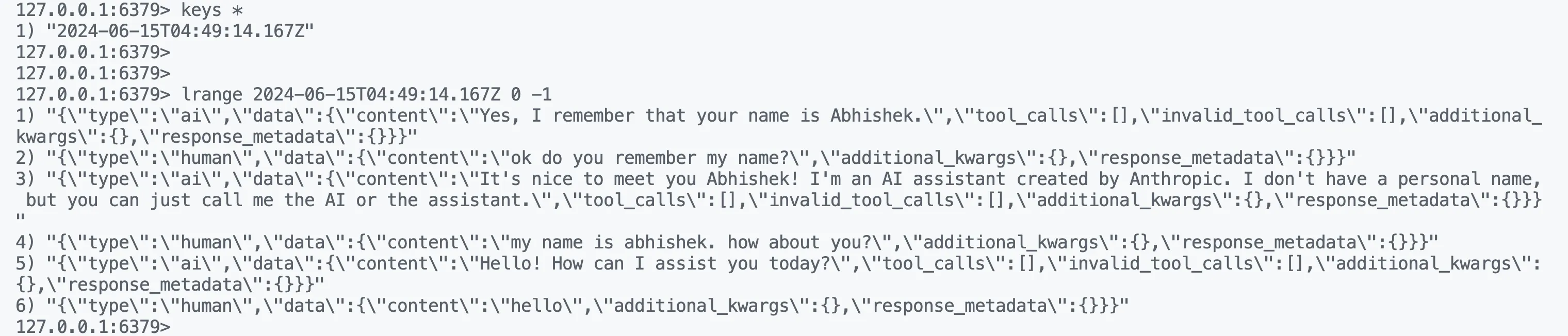
The current implementation uses the
node-redis
client, but I wanted to try out iovalkey
client. I am not a JS/TS expert, but it was simple enough to port the existing implementation.You can refer to the code on GitHubAs far as the client (chat) app is concerned, I only had to make a few changes to switch the implementation:
- Comment out
import { RedisChatMessageHistory } from "@langchain/redis";
- Add
import { ValkeyChatMessageHistory } from "./valkey_chat_history.js";
- Replace
RedisChatMessageHistory
withValkeyChatMessageHistory
(while creating thememory
instance)
It worked the same way as above. Feel free to give it a try!
It's still early days for the Valkey (at the time of writing), and there is a long way to go. I'm really interested in the how the project evolves and also the client ecosystem for Valkey.
Happy Building!
Any opinions in this post are those of the individual author and may not reflect the opinions of AWS.